How do you JSON.stringify an ES6 Map?
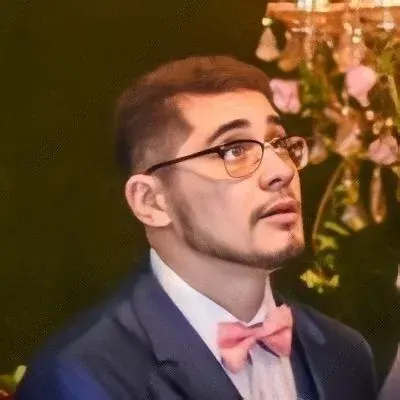
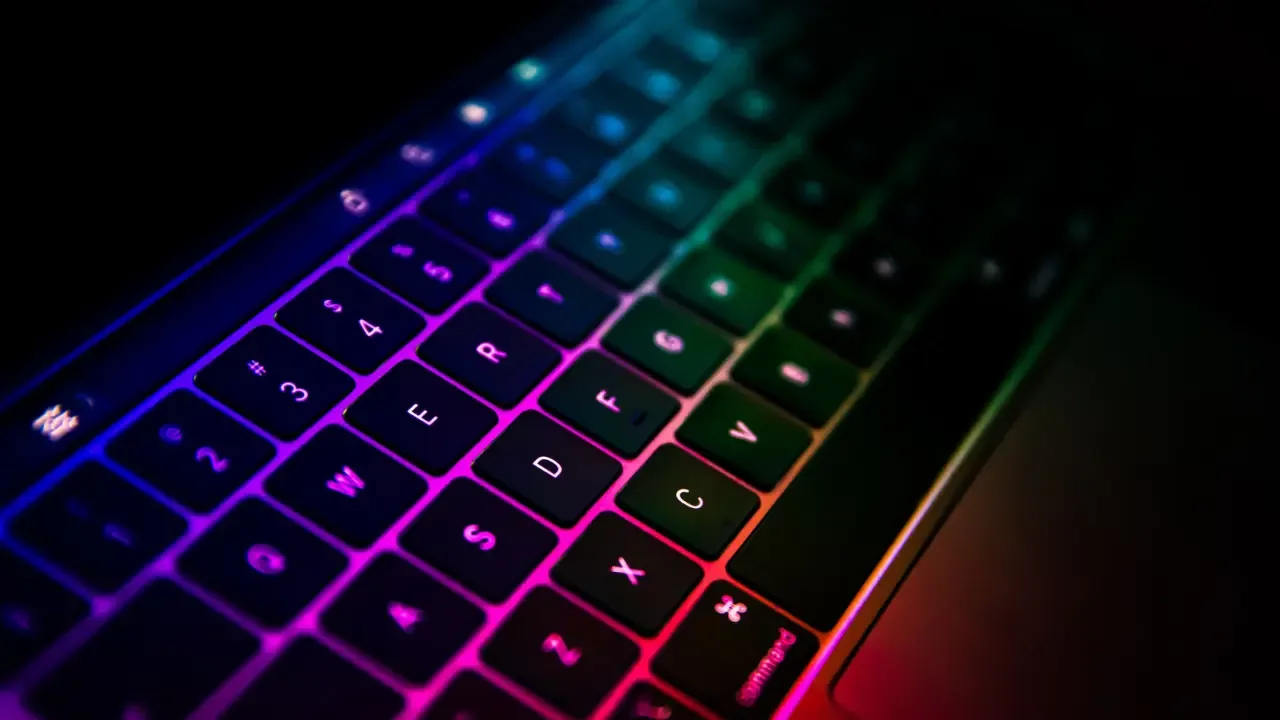
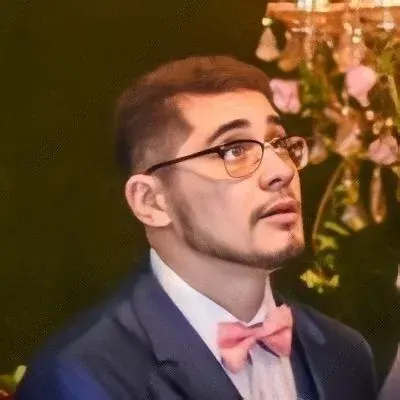
π‘ How to JSON.stringify an ES6 Map πΊοΈ
So, you've decided to level up your JavaScript game by using ES6 Maps. Good move! They offer more flexibility and functionality compared to traditional JavaScript objects. However, there's a challenge you've encountered: How do you jsonify (or string-ify) an ES6 Map using JSON.stringify()?
No worries, my friend! π€ I've got you covered. Let's dive into the solution!
β οΈ The Problem:
The native JSON.stringify() method in JavaScript does not natively support Maps. It only works with JSON-compatible data types like objects, arrays, strings, numbers, booleans, and null. So when you try to stringify your Map, you might end up with an empty object or an error.
β The Solution:
To handle this issue and properly stringify your ES6 Map, you have a few options. Let's go through each one:
Option 1: Convert Map to Array of Key-Value Pairs
The first solution is to convert your Map data into an array of key-value pairs and then stringify the array. Here's an example:
const myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
const mapArray = Array.from(myMap); // Convert Map to Array
const jsonString = JSON.stringify(mapArray); // Stringify the array
console.log(jsonString);
The output will be:
[["key1","value1"],["key2","value2"]]
Option 2: Write a Custom Serializer Function
Another approach is to write a custom serializer function that converts the Map to a plain object before stringifying. Here's an example:
function mapToJson(map) {
return JSON.stringify([...map]);
}
const myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
const jsonString = mapToJson(myMap); // Use the custom serializer function
console.log(jsonString);
The output will be the same as Option 1:
[["key1","value1"],["key2","value2"]]
Option 3: Use a Third-Party Library
If you prefer not to reinvent the wheel or need additional features, you can leverage third-party libraries like json-stringify-extended
or flatted
. These libraries provide extended JSON.stringify() functionality, supporting Maps and other complex data types. Here's an example using json-stringify-extended
:
const stringifyExtended = require('json-stringify-extended');
const myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
const jsonString = stringifyExtended(myMap); // Use the extended stringify method
console.log(jsonString);
The output will be the same as the first two options.
π₯ Your Turn:
Now that you know how to JSON.stringify an ES6 Map, it's time to put it into action! Choose the option that suits your needs and take your JavaScript projects to the next level.
π Share your thoughts:
Have you encountered any other challenges while working with ES6 Maps or JSON.stringify()? How did you solve them? Share your experiences in the comments section below! Let's learn from each other. π
π Don't forget to share this post and help your fellow developers who might be struggling too!
Happy coding! π©βπ»π¨βπ»