How do you access the matched groups in a JavaScript regular expression?
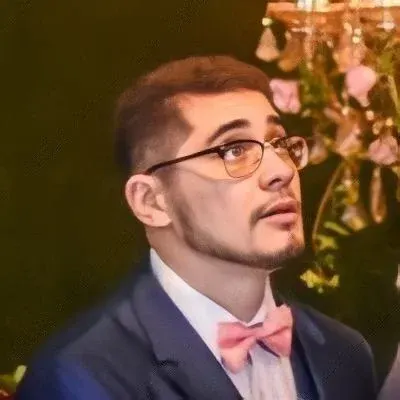
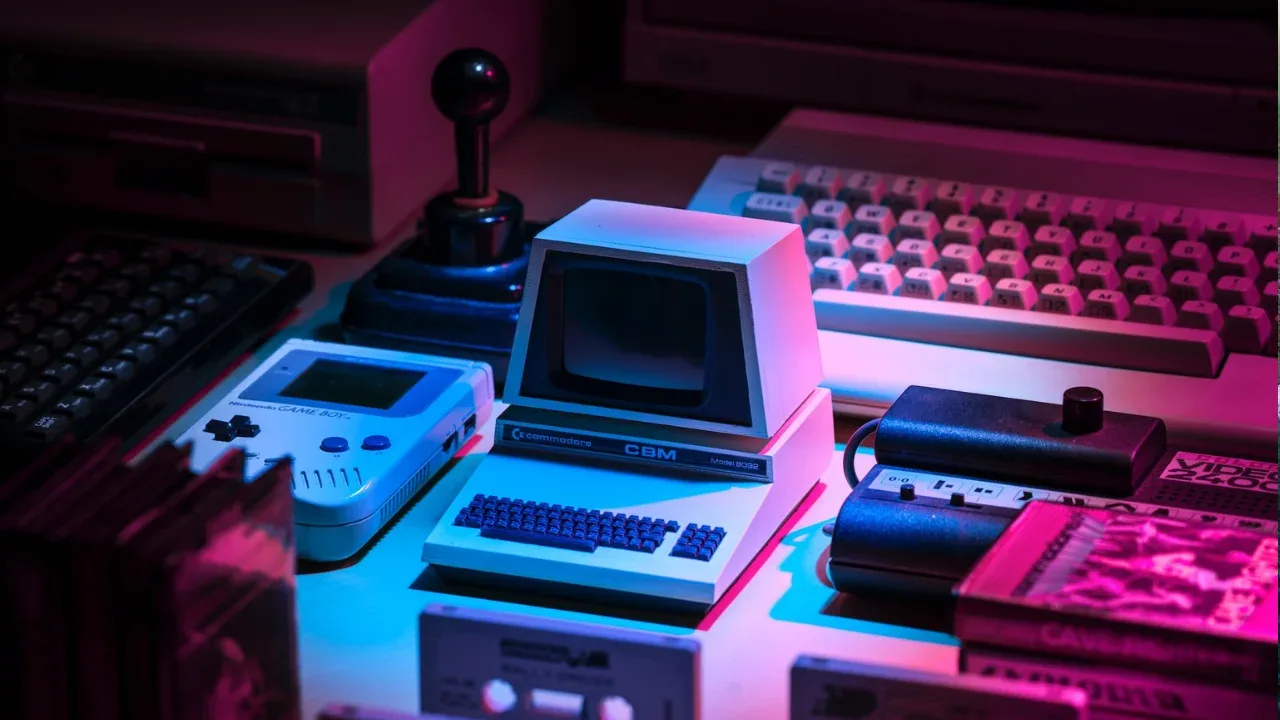
📝 💻 How to Access Matched Groups in JavaScript Regular Expressions
Are you having trouble accessing matched groups in JavaScript regular expressions? Don't worry! In this blog post, we'll explore a common issue and provide you with easy solutions to help you access those pesky parenthesized substrings. Let's dive in!
🔍 The Problem: You have a string and want to match a specific portion using a regular expression. However, when you try to access the matched group, you either get "undefined" or an unexpected value.
📦 The Example: Let's consider this example:
var myString = "something format_abc"; // I want "abc"
var arr = /(?:^|\s)format_(.*?)(?:\s|$)/.exec(myString);
console.log(arr); // Prints: [" format_abc", "abc"] .. so far so good.
console.log(arr[1]); // Prints: undefined (???)
console.log(arr[0]); // Prints: format_undefined (!!!)
🤔 What's Going Wrong? You might be scratching your head, wondering what went wrong. But fear not, the issue is not with your regular expression code. The problem lies in the value you are testing against.
✨ The Solution: In the example provided, the actual string we are testing against is "date format_%A." This behavior of returning "undefined" for "%A" seems strange, but it's not directly related to the question at hand. If you're interested, you can check out our blog post on "Why is a matched substring returning 'undefined' in JavaScript?" for more details.
🔧 The Explanation:
The strange behavior mentioned above occurs because of how the console.log()
function handles its parameters. It follows a similar format to a printf
statement, and when you log a string with a special value, it tries to find the value of the next parameter. This confusion results in unexpected values being displayed.
👣 Next Steps: Now that you understand the root cause of the issue and why it occurred, you can take specific steps to avoid similar problems in the future:
Be aware of special characters or values in your strings and how they can affect output.
Consider using different logging methods or techniques to avoid parameter confusion.
💬 Engage and Share: We hope this blog post has helped you navigate through the issue of accessing matched groups in JavaScript regular expressions. If you found this information valuable, don't forget to share it with your friends and colleagues! Plus, we'd love to hear your thoughts and experiences in the comments below.
Happy coding! ✨🚀
<hr />
📚 Additional Resources:
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
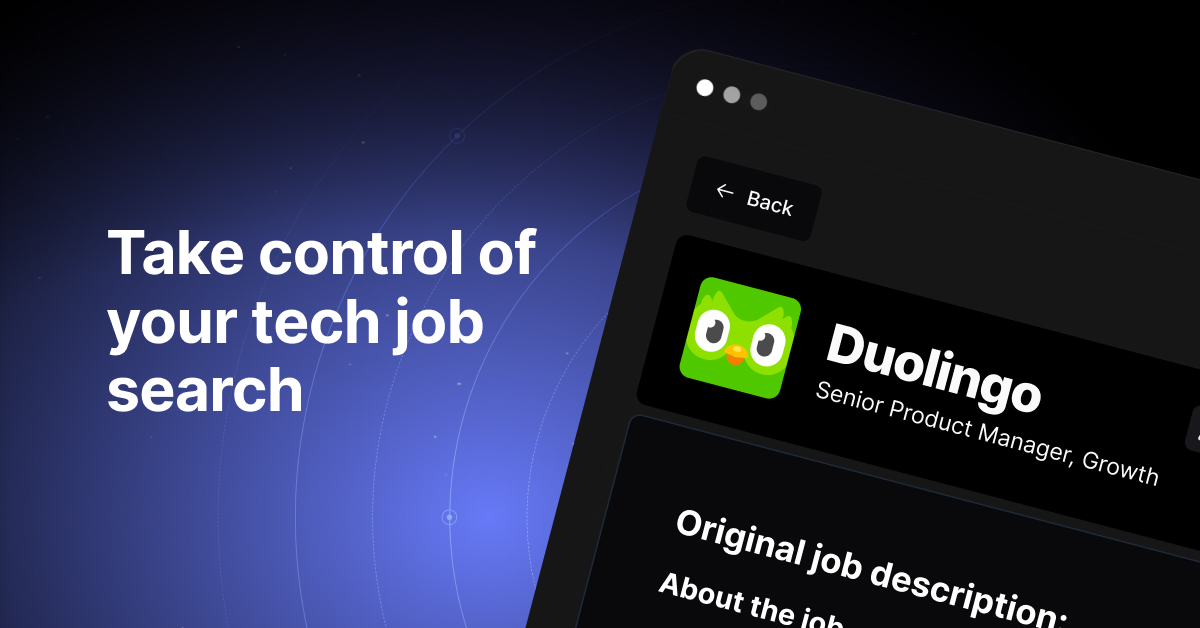