How do JavaScript closures work?
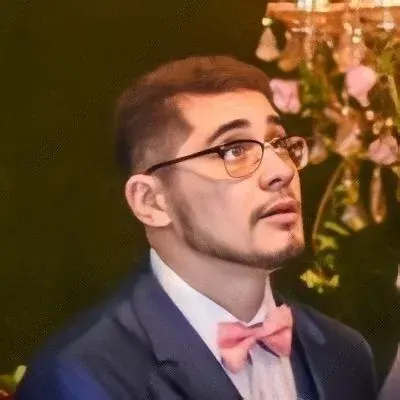
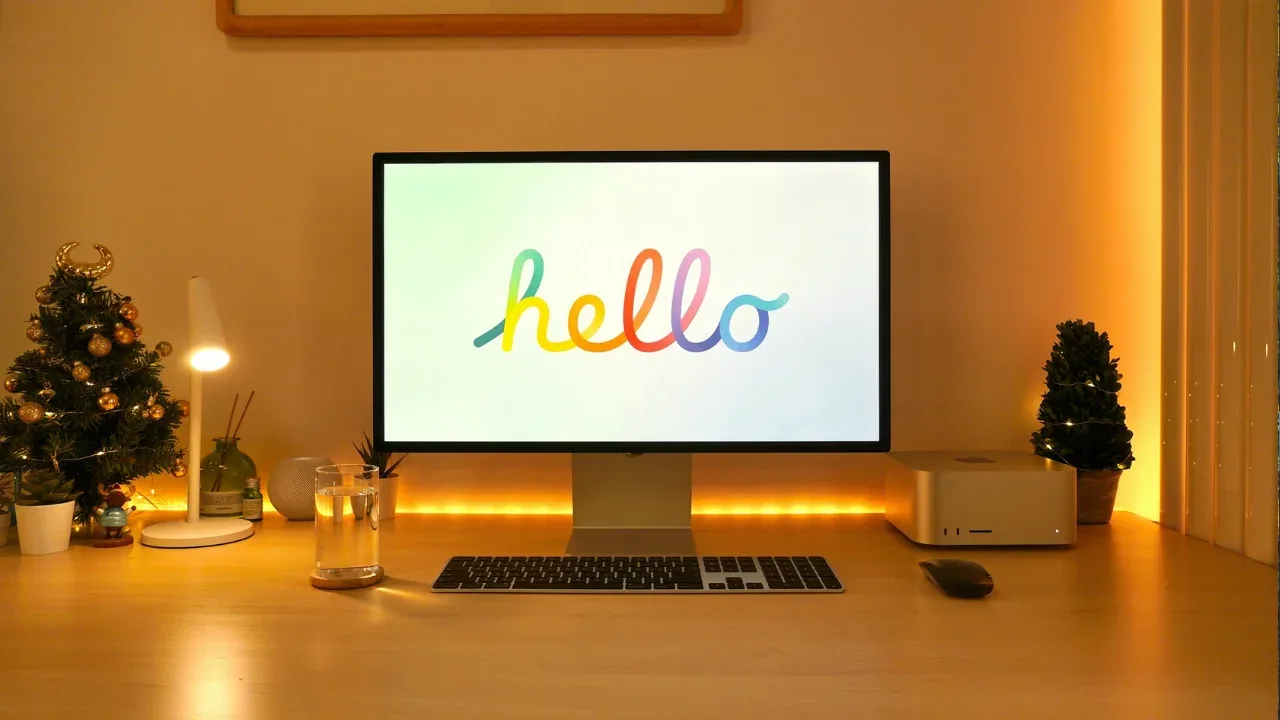
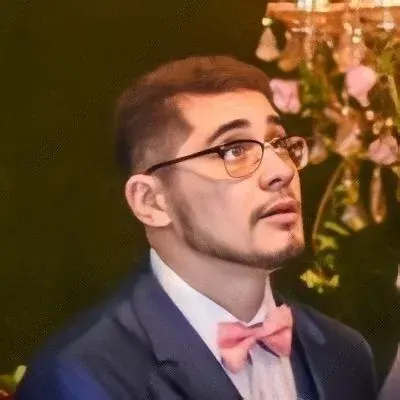
📝 Title: Demystifying JavaScript Closures: Unlocking the Secrets of Functions
Intro: Hey there, web developers! 🌐💻 If you've ever found yourself scratching your head when it comes to understanding JavaScript closures, then you're in luck! 🍀 In today's blog post, we're going to delve deep into the world of closures, demystify their magic, and equip you with the knowledge you need to confidently wield their power. Let's get started on this epic JavaScript journey! 🚀
🔍 Understanding the Basics: Before we embark on our adventure, let's quickly review some fundamental concepts. JavaScript closures are a powerful feature that lets functions access variables from an outer scope even after that scope has been closed. 🎩🔒 They allow you to create functions that remember and manipulate the environment in which they were created. How cool is that? 🤓
🏞️ Visualizing Closures: To better understand closures, let's visualize them using a real-world example. Imagine you're going on a camping trip. 🏕️ In your backpack, you have a map (the function) and a compass (the variable). The compass represents the external environment, while the map represents the function and its inner environment. 🔍🧭
🌟 How Closures Work: In JavaScript, when a function is defined inside another function, the inner function has access to variables in the outer function's scope, forming a closure. Here's the trick: even if the outer function finishes executing, the inner function still remembers and can access those variables. 💡🔓
💡 Example to Illuminate: Let's dive into a code snippet that showcases closures and their power:
function outerFunction() {
const message = "Hello, closure!";
function innerFunction() {
console.log(message);
}
return innerFunction;
}
const myClosure = outerFunction();
myClosure();
In this example, outerFunction
returns innerFunction
as a result. When myClosure()
is called, it can still access the message
variable from its parent scope, revealing the secret message. 😲✉️
🗝️ How to Harness Closure Magic: Now that we've covered the fundamentals, it's time to put closures to work! Here are a few practical use cases where closures shine:
1️⃣ Encapsulating Private Data: Closures allow you to create private variables and functions that are inaccessible from outside the parent function. This helps prevent unintended modifications or unexpected access. It's like having your own secret room hidden from prying eyes! 🚪🔒
2️⃣ Memoization and Caching: By utilizing closures, you can store expensive computations or network requests, saving time and resources. Closures serve as a memory box where you can stash valuable data, ensuring quick access when needed. Snapping your fingers has never been so efficient! ✨📦
✨ Conclusion: Congratulations, fellow code adventurers! 🎉✨ You've successfully conquered the world of JavaScript closures! We've traveled together, unraveling the mysteries and unlocking their incredible potential. Now, it's your turn to utilize closures in your coding quests and level up your JavaScript skills! 💪🔥
Ready to embark on your next coding adventure? 🚀 Share your closure success stories, ask questions, or let us know your favorite use case in the comments below! Let's keep the closure conversation going! 💬💌