How do I use namespaces with TypeScript external modules?
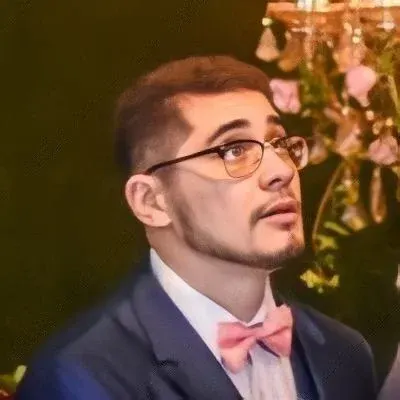
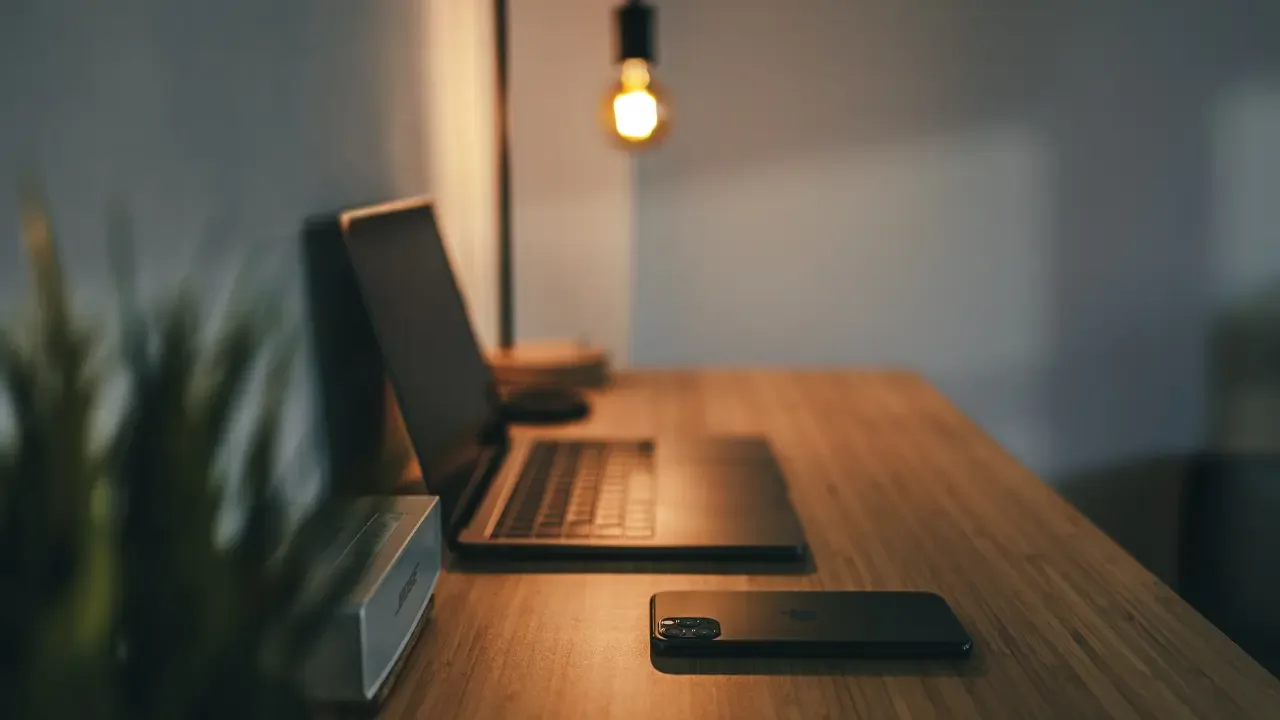
Understanding Namespaces with TypeScript External Modules
Are you struggling with using namespaces in TypeScript external modules? 🤔 Don't worry, you're not alone! Many developers find this concept confusing, but fear not! In this guide, we'll break down the common issues you might encounter and provide easy solutions to help you leverage namespaces effectively. Let's dive in! 💪
The Problem
Let's start by examining the code snippet you provided:
// baseTypes.ts
export namespace Living.Things {
export class Animal {
move() { /* ... */ }
}
export class Plant {
photosynthesize() { /* ... */ }
}
}
// dog.ts
import b = require('./baseTypes');
export namespace Living.Things {
// Error, can't find name 'Animal', ??
export class Dog extends Animal {
woof() { }
}
}
// tree.ts
// Error, can't use the same name twice, ??
import b = require('./baseTypes');
import b = require('./dogs');
namespace Living.Things {
// Why do I have to write b.Living.Things.Plant instead of b.Plant??
class Tree extends b.Living.Things.Plant {
}
}
From the provided code, there are three main issues that can make namespaces with TypeScript external modules confusing:
Undefined References: In the
dog.ts
file, you get an error because theAnimal
class is not recognized. You attempted to extend theAnimal
class, but it is not in scope. 😫Name Collision: In the
tree.ts
file, you encounter an error because you have importedbaseTypes.ts
asb
and then attempted to importdogs.ts
again asb
. This leads to a conflict, as you cannot use the same name twice. 😣Namespace Aliasing: Lastly, in the
tree.ts
file, you question why you need to useb.Living.Things.Plant
instead of justb.Plant
. This aliasing can become confusing and make your code less readable. 🤔
The Solution
Now let's go over the solutions to these issues one by one, so you can use namespaces in TypeScript external modules with ease! 🎉
1. Undefined References: Import Complete Path
To fix the undefined reference error in the dog.ts
file, you need to provide the complete path to the Animal
class when extending it. Update your code as follows:
// dog.ts
import b = require('./baseTypes');
export namespace Living.Things {
export class Dog extends b.Living.Things.Animal {
woof() { }
}
}
By prefixing Animal
with b.Living.Things.
, you are now correctly referring to the Animal
class from the imported module. Problem solved! 🙌
2. Name Collision: Avoid Redundant Import Statements
To avoid the name collision error in the tree.ts
file, make sure you don't import the same module twice using the same name. Update your code as follows:
// tree.ts
import b = require('./baseTypes');
import d = require('./dogs'); // Use different alias here
namespace Living.Things {
class Tree extends b.Living.Things.Plant {
// Now you can use b.Plant instead of b.Living.Things.Plant
}
}
By using a different alias (d
) for the dogs.ts
module import, you avoid the conflict and can use both modules without any issues. Problem solved, once again! 🎊
3. Namespace Aliasing: Use import as Syntax
To simplify your code and make it more readable, you can use the import as
syntax to create a shorter reference to the nested namespace. Update your code as follows:
// tree.ts
import b = require('./baseTypes');
import { Living } from './baseTypes'; // Import the nested namespace
namespace Living.Things {
class Tree extends Living.Things.Plant {
// Now you can use just Plant instead of Living.Things.Plant
}
}
By importing the nested Living
namespace directly, you can reference its members without repeating the entire namespace path. Isn't that much cleaner? 😎
Conclusion
Congratulations for reaching the end of this guide! You've now mastered the usage of namespaces with TypeScript external modules. Remember these key takeaways:
Import the complete path when referencing classes from an imported module.
Avoid name collisions by using unique aliases for each imported module.
Simplify your code by using the
import as
syntax to create shorter references to nested namespaces.
Now it's time for you to put this knowledge into action! Go ahead and refactor your code using these solutions, and get rid of those errors and confusion once and for all. Happy coding! 💻💡
Have you encountered any other issues with TypeScript namespaces and external modules? Share your experiences and thoughts in the comments below and let's have a discussion! 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
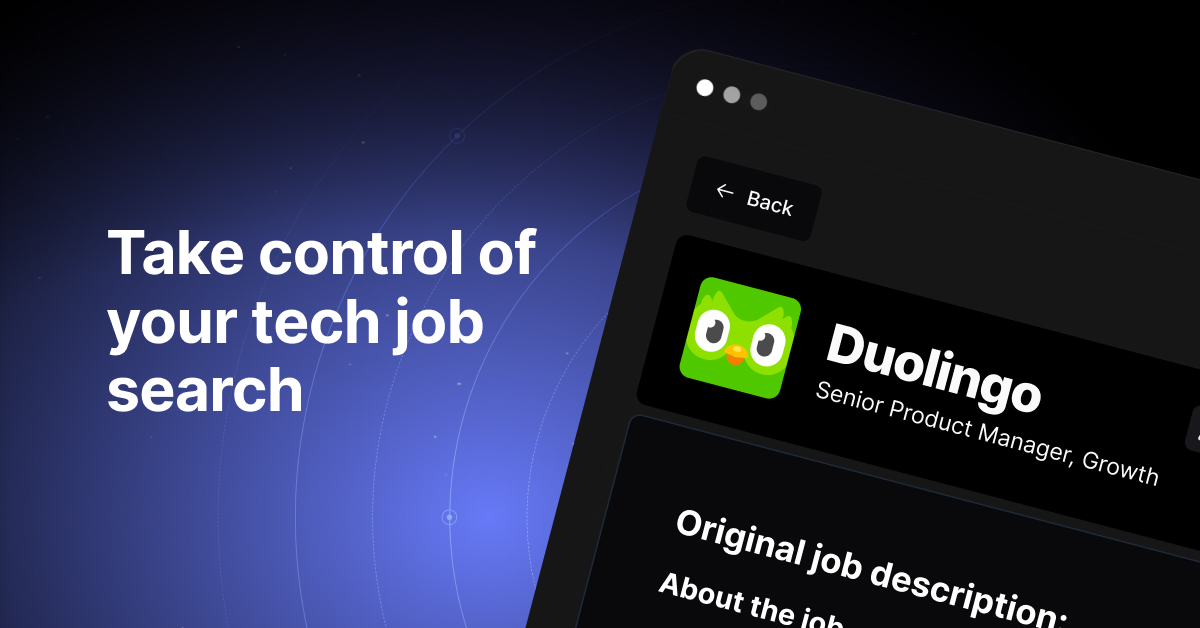