How do I URl encode something in Node.js?
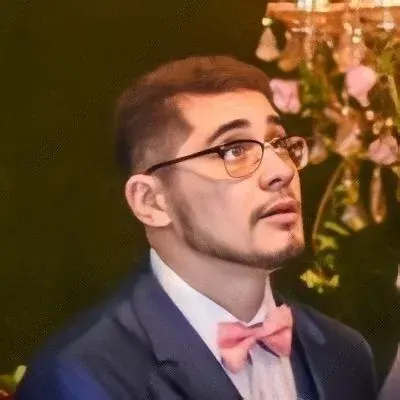
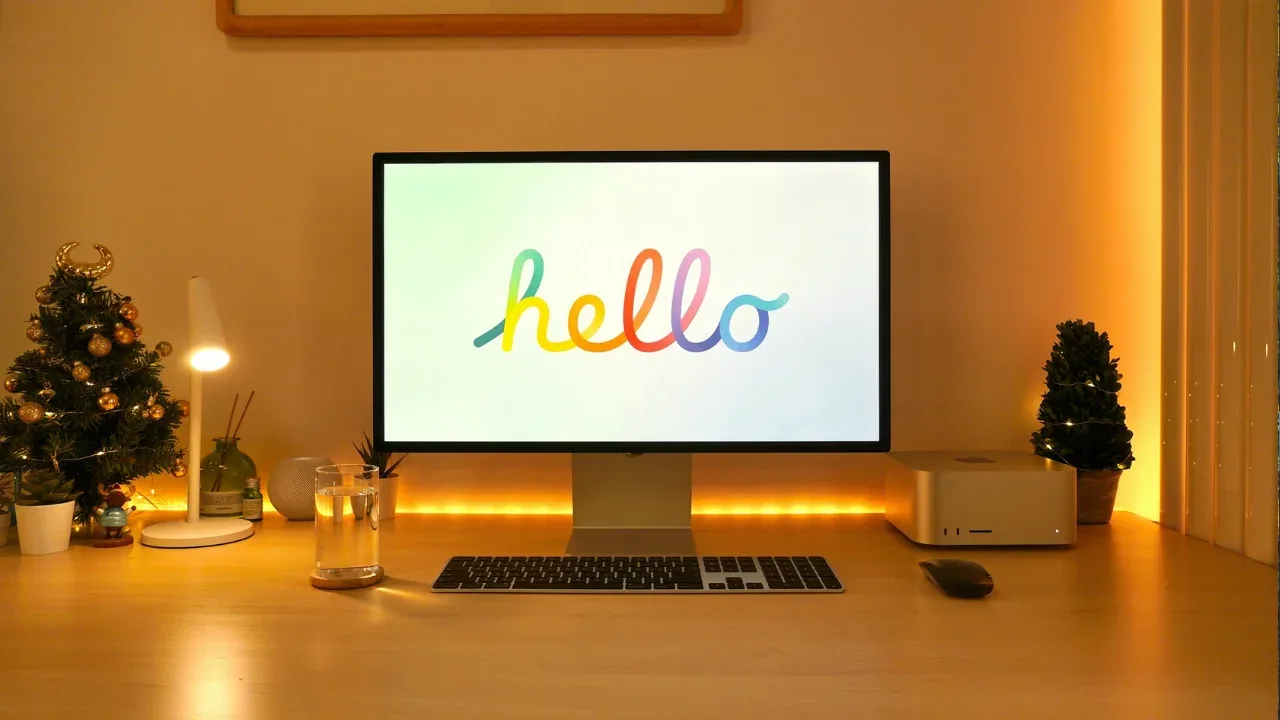
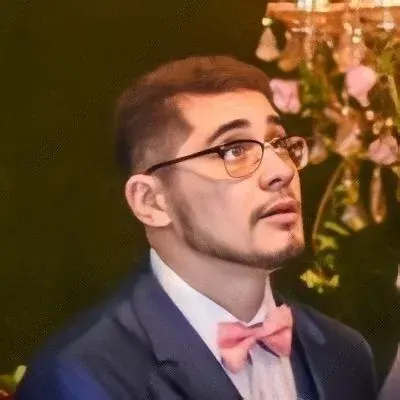
How to URL Encode in Node.js: A Beginner's Guide
So you want to URL encode something in Node.js, huh? Well, you've come to the right place! 😎 In this guide, we'll explore everything you need to know about URL encoding in Node.js, address common issues, and provide easy solutions. Let's dive in!
Understanding URL Encoding
Before we jump into the how, let's quickly understand what URL encoding is. URL encoding is the process of converting special characters and reserved characters into a format that can be safely transmitted over the internet. This ensures that the URL is valid and doesn't break when users or other services interact with it.
The Quest for URL Encoding in Node.js
So you have this piece of code that you want to URL encode:
SELECT name FROM user WHERE uid = me()
Now, the question is, do you need to download a module for URL encoding in Node.js? The answer is: it depends. 😅
If you want to use Node.js' built-in functionality, you don't need any additional modules. However, if you're using a third-party library like request
, you need to check if it provides URL encoding functionality. In this case, lucky for you, the request
module does support URL encoding!
URL Encode Using the request
Module
To URL encode a string using the request
module, simply use the encodeURIComponent()
function. Here's an example:
const request = require('request');
const url = 'https://api.example.com/search?q=' + encodeURIComponent('SELECT name FROM user WHERE uid = me()');
request(url, (error, response, body) => {
// Handle the response
});
In the above example, we concatenate the URL with the URL-encoded string using encodeURIComponent()
. This function takes care of replacing special characters with their URL-encoded equivalents.
URL Encode Using Node.js' Built-in Functionality
If you don't want to use the request
module or any third-party libraries, you can make use of the built-in querystring
module in Node.js. Here's how you can achieve URL encoding using this module:
const querystring = require('querystring');
const url = 'https://api.example.com/search?' + querystring.encode({ q: 'SELECT name FROM user WHERE uid = me()' });
request(url, (error, response, body) => {
// Handle the response
});
In the example above, we use the querystring.encode()
function to encode the query parameter value. This function takes an object as input and returns a URL-encoded string.
Share Your URL Encoding Success Story! 💌
Congratulations! You've successfully learned how to URL encode something in Node.js. Now, it's time to put your newfound knowledge to use and elevate your coding game! Feel free to share your experiences, use cases, or any challenges you faced along the way in the comments section below. Let's learn from each other and grow together! 💪
Happy URL encoding, fellow developers! 😄