How do I update/upsert a document in Mongoose?
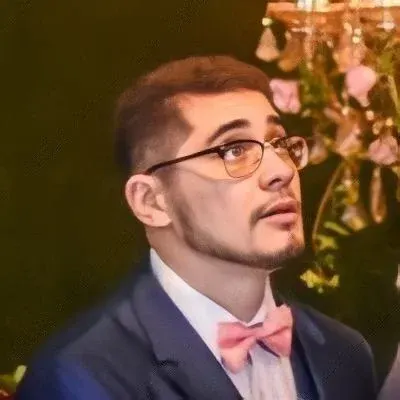
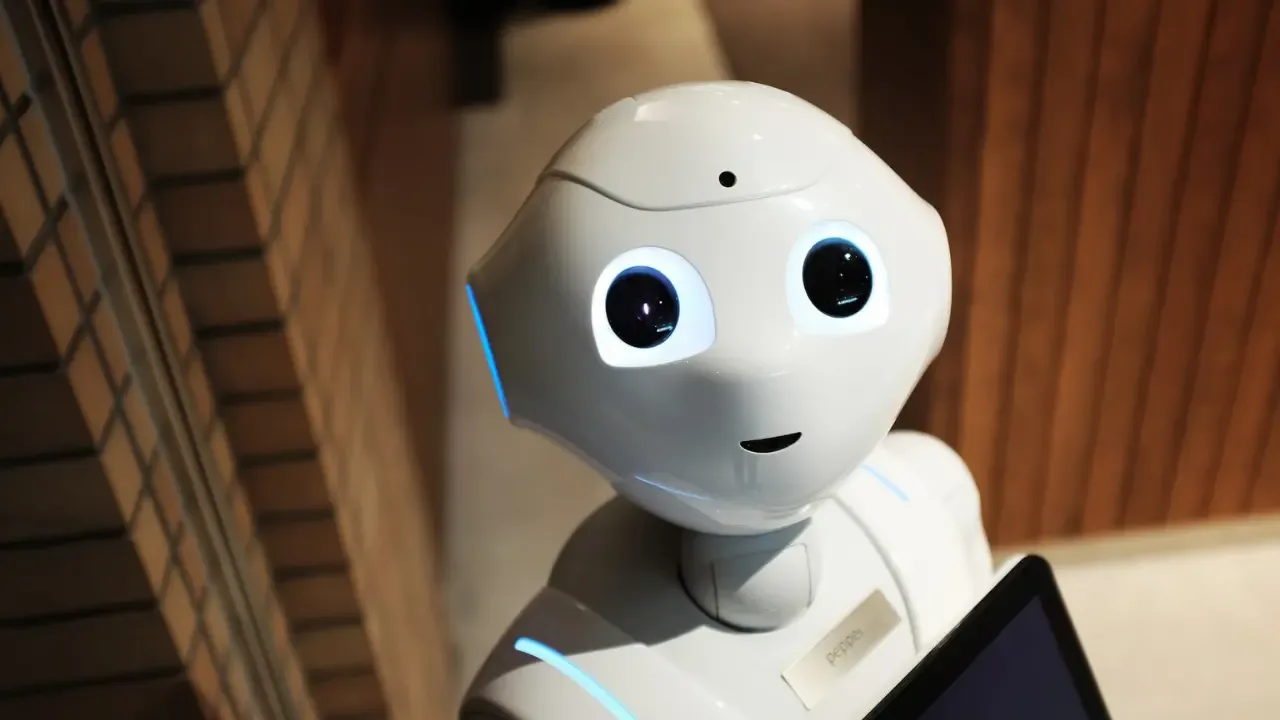
How to Update/Upsert a Document in Mongoose
πHey there, fellow developers! π Are you struggling with the concept of updating documents in Mongoose? Fear not, because I'm here to guide you through it. Updating or upserting documents shouldn't be a headache-inducing task, and I'm here to make it super easy for you! Let's dive in. πββοΈ
The Problem: Updating Existing Documents
From the context you provided, it seems like you have a Contact
schema and model in your Mongoose application. When receiving a request from the client, you need to update an existing contact if it already exists, or add a new contact if it doesn't.
Here are the issues you faced:
Calling
contact.save()
throws an error if a contact with the same phone number already exists. This makes sense because you've set thephone
field to be unique.You can't directly call
update()
on thecontact
object since that method is not available on a document.If you try using
Contact.update()
with theupsert
option, passing thecontact
object as the second parameter leads to an infinite loop.When you use an associative array of the request properties instead, the update works, but you lose the reference to the specific contact's
createdAt
andupdatedAt
properties.
All valid frustrations! But fret not - I'm here to provide you with the perfect solution. π
The Solution: findOneAndUpdate()
To update an existing document if it exists, or add it if it doesn't, we can make use of the findOneAndUpdate()
method provided by Mongoose. This method atomically finds and updates a document based on a specific query.
Here's how you can use it:
var query = { phone: request.phone };
var update = {
phone: request.phone,
status: request.status
};
var options = {
upsert: true,
new: true, // Return the modified document, not the original
setDefaultsOnInsert: true // Set default values for fields if inserting a new document
};
Contact.findOneAndUpdate(query, update, options, function(err, contact) {
if (err) {
// Handle the error
console.error(err);
return;
}
// Use the updated/contact document as needed
console.log(contact);
});
With this approach, you can efficiently handle the scenario where you want to update existing contacts or add new ones without worrying about errors or losing important document properties.
The Call-to-Action: Share Your Thoughts and Experiences
π¬I hope this guide helped clarify the process of updating/upserting documents in Mongoose. Now it's your turn to put this knowledge into action! Try implementing findOneAndUpdate()
in your own projects and let me know your experiences. Did you encounter any further challenges or have any additional tips to share? I'd love to hear from you in the comments! Let's continue this conversation and help each other become better developers. Happy coding! π©βπ»π¨βπ»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
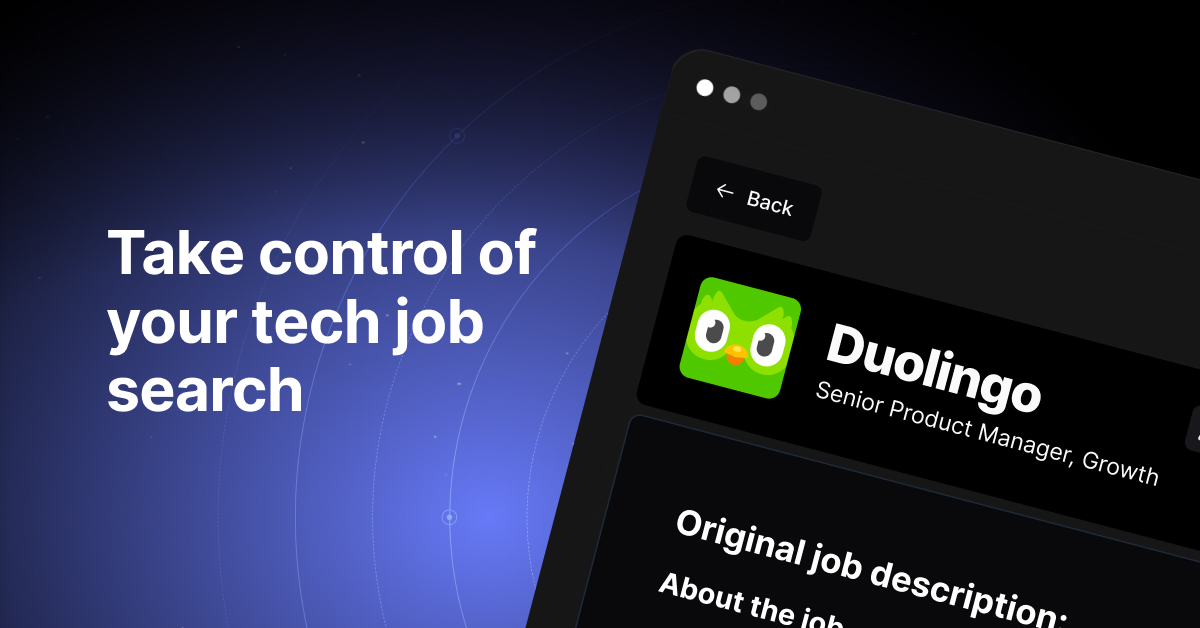