How do I test for an empty JavaScript object?
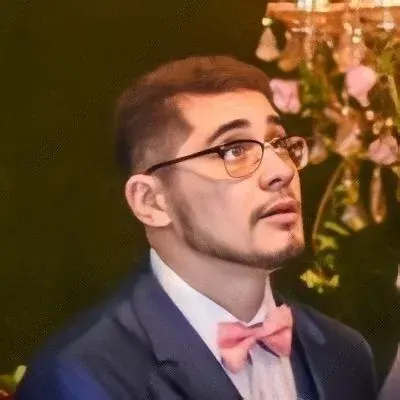
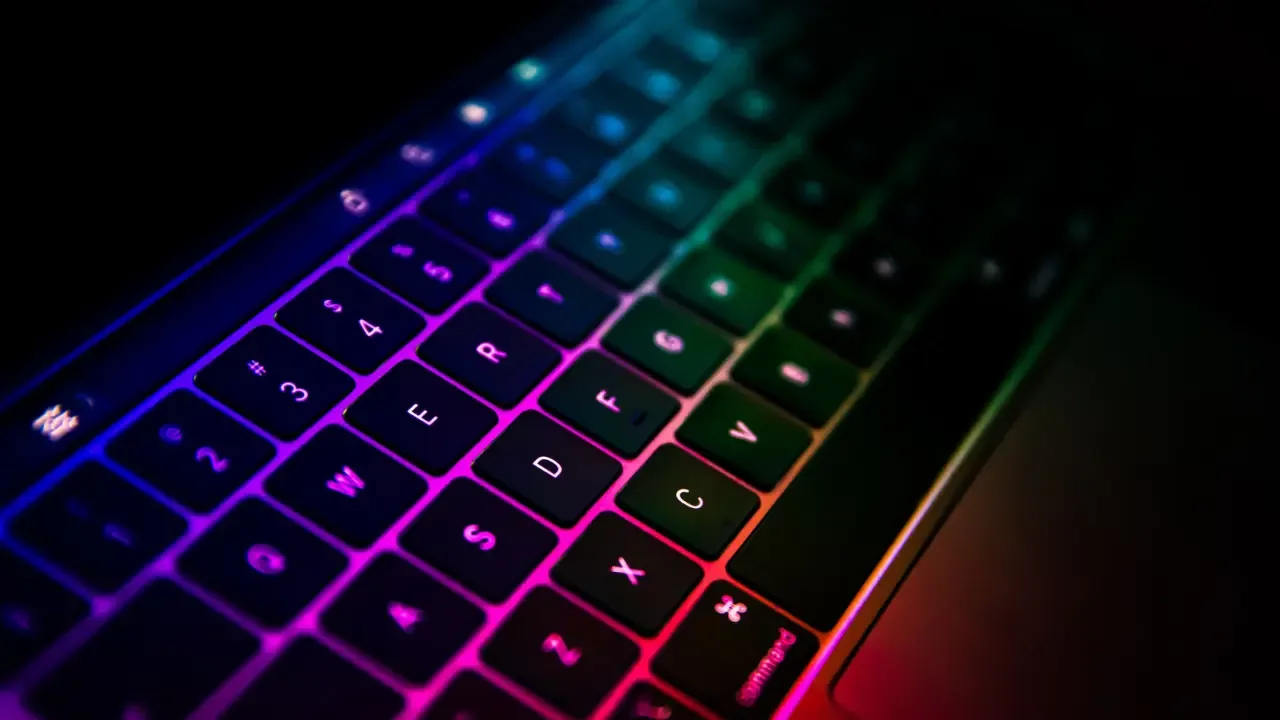
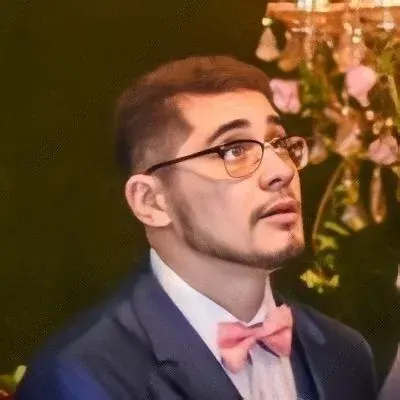
How to Test for an Empty JavaScript Object ๐งช๐
So, you made an AJAX request and sometimes you get an empty object as the response. Don't worry, we've got you covered! In this guide, we'll walk you through the process of testing for an empty JavaScript object and provide easy solutions to tackle this common issue. Let's dive in! ๐ป๐ก
The Common Issue: Empty JavaScript Object ๐ฆ
After receiving an AJAX response, there might be instances where the returned object is empty, like this:
var a = {};
Now, how can we efficiently check if the object a
is empty or not? Let's explore some solutions! ๐๐
Solution 1: Using the Object.keys()
Method ๐
One way to determine if a JavaScript object is empty is by using the Object.keys()
method. This method returns an array of keys from the object. If the array is empty, it means the object is also empty. Here's an example:
if (Object.keys(a).length === 0) {
console.log("The object is empty!");
} else {
console.log("The object is not empty!");
}
In the code snippet above, we check if the length of the array returned by Object.keys(a)
is equal to zero. If it is, we can conclude that the object a
is empty. Easy peasy, right? ๐๐
Solution 2: Using JSON.stringify()
๐งฌ
Another approach to determine if an object is empty is by using the JSON.stringify()
method. This method converts the object into a JSON string representation. If the resulting string is "{}"
, then voilร , the object is empty! Check out the code snippet below:
if (JSON.stringify(a) === "{}") {
console.log("The object is empty!");
} else {
console.log("The object is not empty!");
}
By comparing the JSON string representation of the object with "{}"
, we can determine its emptiness. Cool, right? ๐๐
The Call-to-Action: Share Your Thoughts! ๐ฃ๐ฌ
We hope these easy solutions help you test for an empty JavaScript object. Have any other creative ideas or thoughts to share? Leave them in the comments below! We'd love to hear from you. ๐๐ญโ๏ธ
Remember, understanding how to test for an empty JavaScript object is a handy skill when dealing with AJAX responses. ๐๐ช
Happy coding! ๐๐