How do I store an array in localStorage?
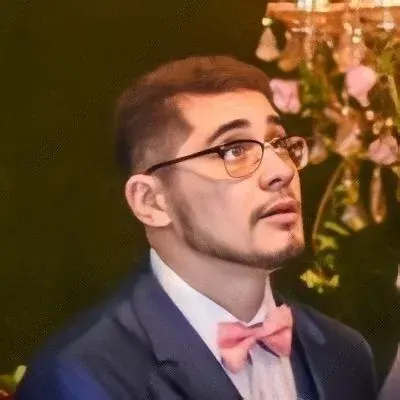
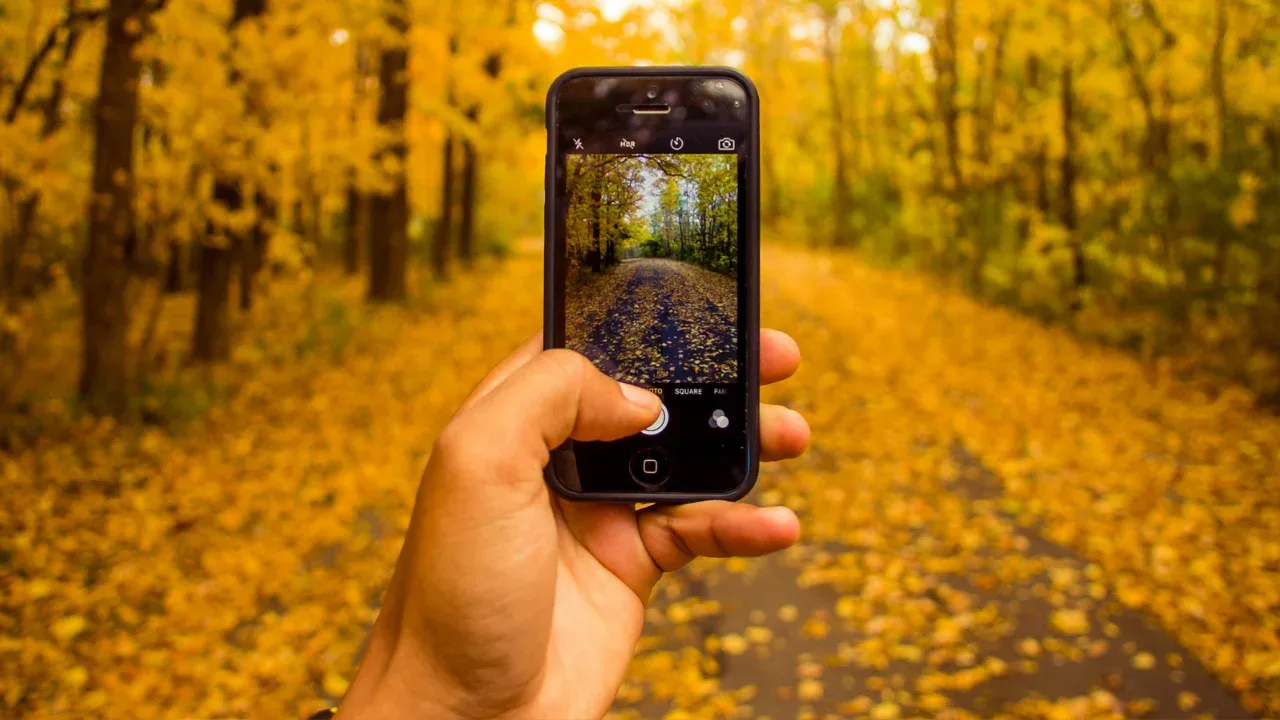
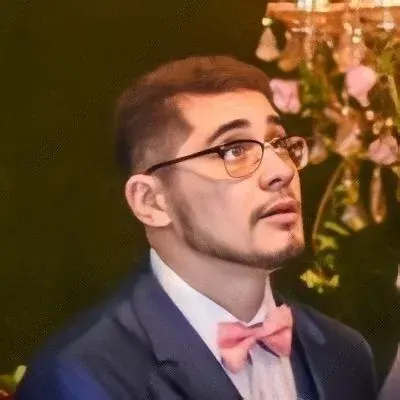
How to Store an Array in localStorage 📦
So you want to store an array in localStorage, huh? Guess what, you're not alone in this quest! Many developers have faced this challenge and found themselves scratching their heads 🤔. But fear not! In this guide, I'll walk you through the common issues and provide easy solutions to help you overcome this hurdle 🏃♀️.
The Problem 🧩
Let's start by understanding the problem you encountered. In your code snippet, you mentioned that you're able to create and manipulate the array names
. However, when it comes to storing it in localStorage, things get tricky 😫.
Your attempted solution looks like this:
var localStorage[names] = new Array();
localStorage.names[0] = prompt("New member name?");
Here's where the misconception lies. localStorage is not an object that you can directly assign properties to like the code above suggests. It is actually a key-value pair storage system that can only handle strings as values 👀.
The Solution 🎉
To successfully store an array in localStorage, you need to convert it into a string format. Luckily, JavaScript provides us with some handy functions to achieve this.
Step 1: Convert the Array to a String
Use the JSON.stringify()
method to convert your array into a string representation:
var names = ["Alice", "Bob", "Charlie"];
var namesString = JSON.stringify(names);
Step 2: Store the String in localStorage
Now that you have your array as a string, you can store it in localStorage using the setItem()
method:
localStorage.setItem("names", namesString);
Step 3: Retrieve the Array from localStorage
When you need to retrieve the array from localStorage, you'll need to reverse the process. First, retrieve the string value using the getItem()
method, then convert it back to an array using JSON.parse()
:
var storedNamesString = localStorage.getItem("names");
var storedNames = JSON.parse(storedNamesString);
And voilà! You now have your array back and ready to be used in your code 🎉.
A Quick Note about localStorage Limitations ⚠️
Keep in mind that localStorage has its limitations. The total amount of data you can store using localStorage varies across different browsers, typically ranging from 2-10MB. If you exceed this limit, you may encounter issues or even have your data truncated.
Let's Recap! 📝
Convert the array to a string using
JSON.stringify()
.Store the string in localStorage using
setItem()
.Retrieve the string from localStorage using
getItem()
.Convert the string back to an array using
JSON.parse()
.
Now that you know how to store an array in localStorage, you can level up your web development skills and save your precious data without losing your sanity 🚀.
If you found this guide helpful or have any questions, let me know in the comments below. Happy coding! 💻💡
Note: Don't forget to handle potential errors, such as null values or invalid JSON formats. And remember, the power to conquer localStorage is in your hands! 💪