How do I return the response from an asynchronous call?
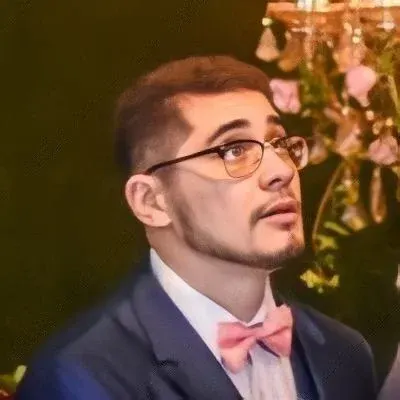
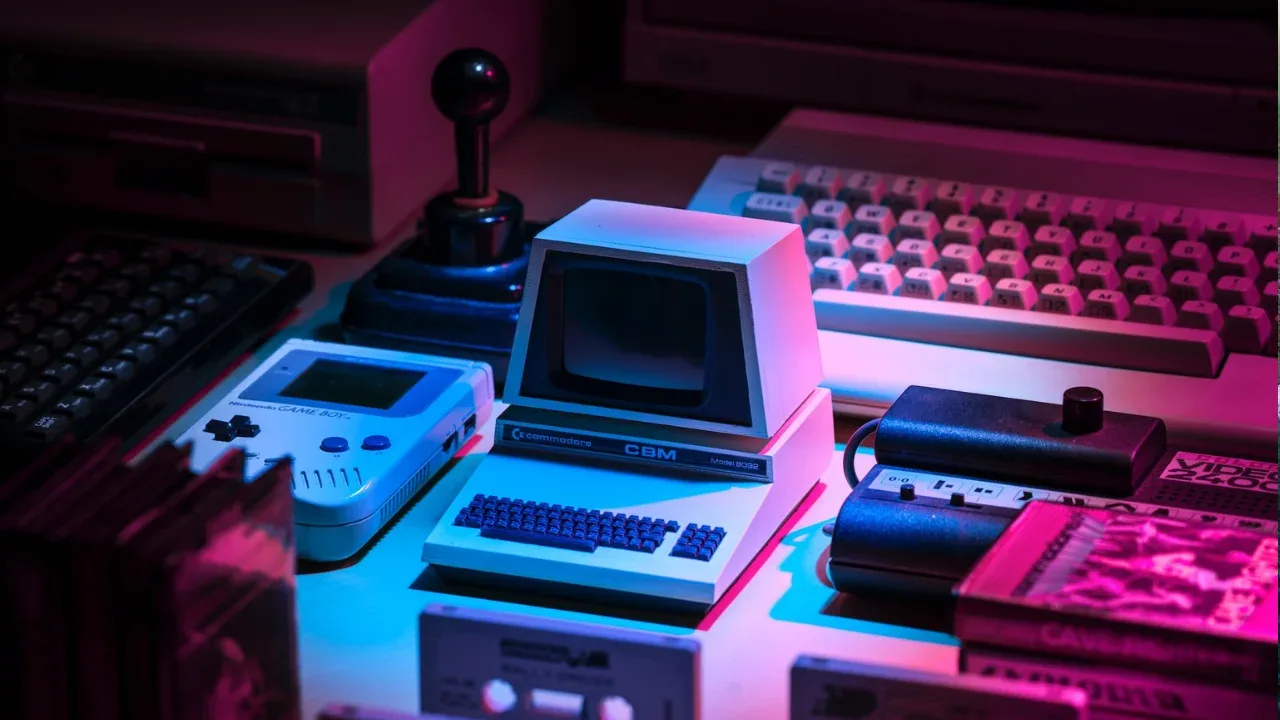
📝 Title: How to Get the Response from an Asynchronous Call in JavaScript
Are you struggling to return the response from an asynchronous call in JavaScript? 🤔 Don't worry, you're not alone! Many developers face this common issue when handling asynchronous operations. In this blog post, we will explore various scenarios and provide easy solutions to help you tackle this problem effectively. So let's dive in! 💪💻
⚠️ The Common Dilemma
Let's start by examining a common scenario where you encounter difficulties while returning the response from an asynchronous call. For instance, you have a function called foo
that makes an asynchronous request, but you're unsure about how to obtain and return the response. 😕 Here's an example of such a situation using different libraries:
function foo() {
var result;
// Using jQuery's ajax function
$.ajax({
url: '...',
success: function(response) {
result = response;
}
});
return result; // Always returns `undefined`
}
function foo() {
var result;
// Using Node.js
fs.readFile("path/to/file", function(err, data) {
result = data;
});
return result; // Always returns `undefined`
}
function foo() {
var result;
// Using the `then` block of a Promise
fetch(url).then(function(response) {
result = response;
});
return result; // Always returns `undefined`
}
🤷♂️ The Reason Behind Undefined Results
In all of the above examples, the issue arises because JavaScript executes the asynchronous code separately from the synchronous code. So when the return result
statement is encountered, the asynchronous call hasn't had a chance to complete yet, resulting in the result
variable still being undefined
.
🔍 The Solution: Promises and Async/Await
To overcome this challenge, you can utilize Promises or the newer ES8 feature, async/await. Let's explore both approaches.
🌟 Promises Promises are a powerful tool for handling asynchronous operations in JavaScript. They allow you to chain methods and handle success or failure cases. Here's how you can modify the previous code to return the response using Promises:
function foo() {
return new Promise(function(resolve, reject) {
// Using jQuery's ajax function
$.ajax({
url: '...',
success: function(response) {
resolve(response);
},
error: function(error) {
reject(error);
}
});
});
}
function foo() {
return new Promise(function(resolve, reject) {
// Using Node.js
fs.readFile("path/to/file", function(err, data) {
if (err) {
reject(err);
} else {
resolve(data);
}
});
});
}
function foo() {
return fetch(url);
}
✨ Async/Await
Async/Await is a more recent addition to JavaScript and provides a cleaner syntax for handling asynchronous code. By using the async
keyword in a function and await
before calling any asynchronous functions, you can simplify your code. Here's an example:
async function foo() {
// Using jQuery's ajax function
try {
const response = await $.ajax({ url: '...' });
return response;
} catch (error) {
throw new Error(error);
}
}
async function foo() {
// Using Node.js
try {
const data = await fs.promises.readFile("path/to/file");
return data;
} catch (error) {
throw new Error(error);
}
}
async function foo() {
// Using fetch API
const response = await fetch(url);
return response;
}
🎉 Wrap Up
Now you have two powerful methods at your disposal to retrieve the response from an asynchronous call: Promises and async/await. It's up to you to choose the one that fits your coding style and project requirements best.
📢 Make It Share-worthy
Did you find this blog post helpful? Share it with your fellow developers who might be facing the same challenge! Let's spread the knowledge and save other developers from the confusion around returning responses from asynchronous calls in JavaScript.
👇 Start Engaging!
Have you encountered any other struggles while handling asynchronous code? Share your experiences and questions in the comments below. Let's discuss and find solutions together! 💬🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
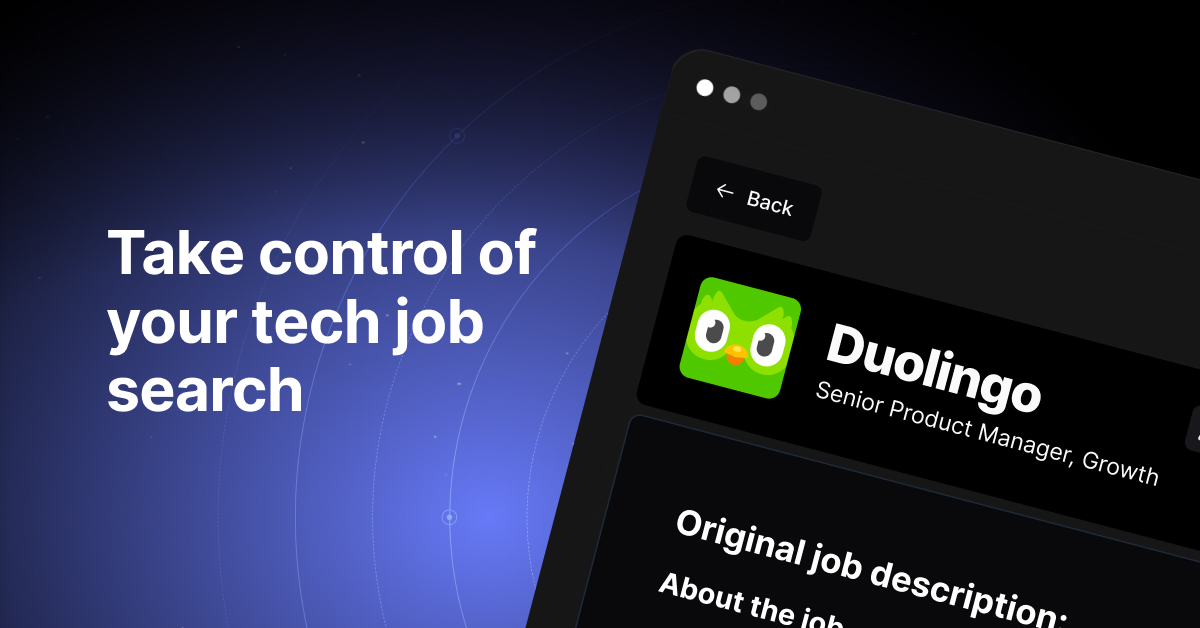