How do I replace all occurrences of a string in JavaScript?
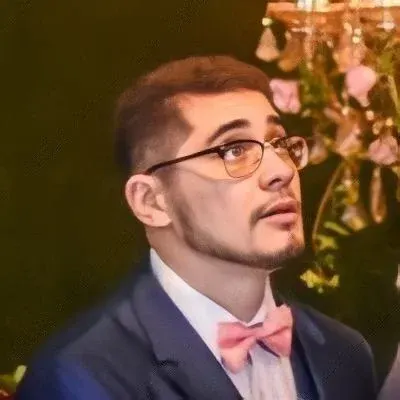
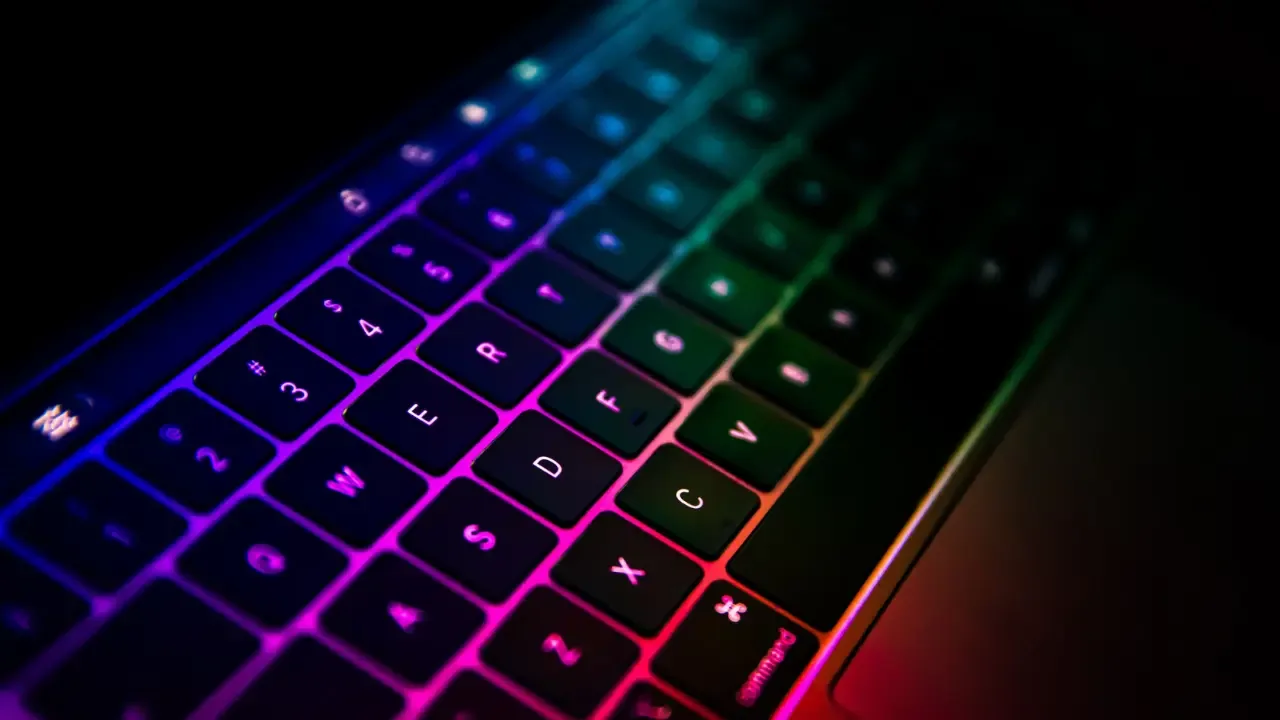
🎉How to Replace All Occurrences of a String in JavaScript?🎉
So, you want to replace all occurrences of a string in JavaScript? You've come to the right place! 🙌
The Problem 😩
Let's say you have a string:
const s = "Test abc test test abc test test test abc test test abc";
And, you want to replace all occurrences of the string 'abc'
. 👀
The first solution that may come to mind is using the replace()
method:
s = s.replace('abc', '');
But wait, this only replaces the first occurrence of 'abc'
! 😱
The Solution 💡
To replace all occurrences of a string, you can use a regular expression with the replace()
method. 🚀
s = s.replace(/abc/g, '');
In the regular expression /abc/g
:
/abc/
is the pattern you want to match, which in this case is'abc'
.g
stands for "global", indicating that you want to match all occurrences of the pattern in the string. 🌍
By including the g
flag in the regular expression, you can replace all occurrences of 'abc'
with an empty string. And voila! 🎉
Final Thoughts 🤔
Replacing all occurrences of a string in JavaScript is as simple as using a regular expression with the replace()
method and the g
flag. 😎
Now that you've mastered this technique, go forth and conquer those string replacements! 💪
If you have any further questions or found this guide helpful, please let us know in the comments below. We would love to hear from you! 📝
👉 Happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
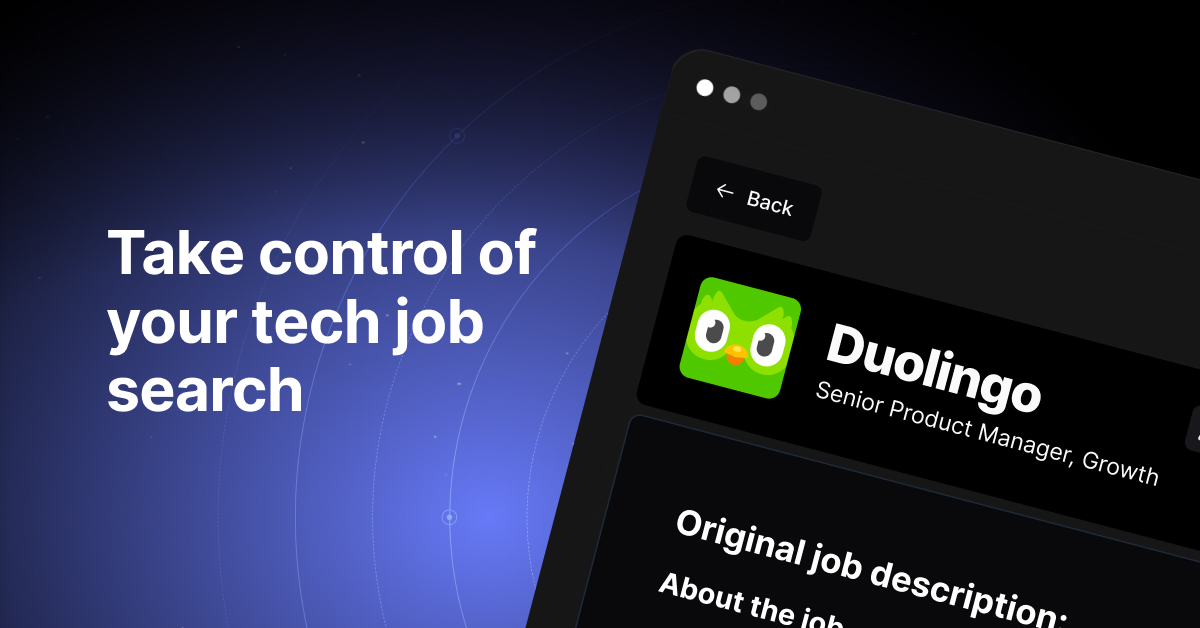