How do I replace a character at a particular index in JavaScript?
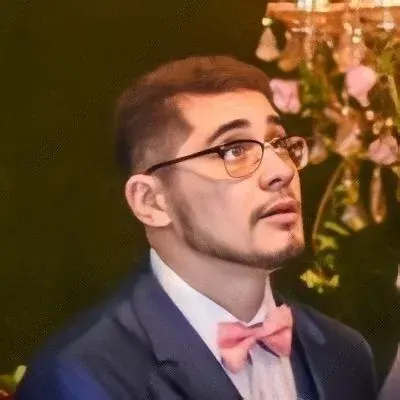
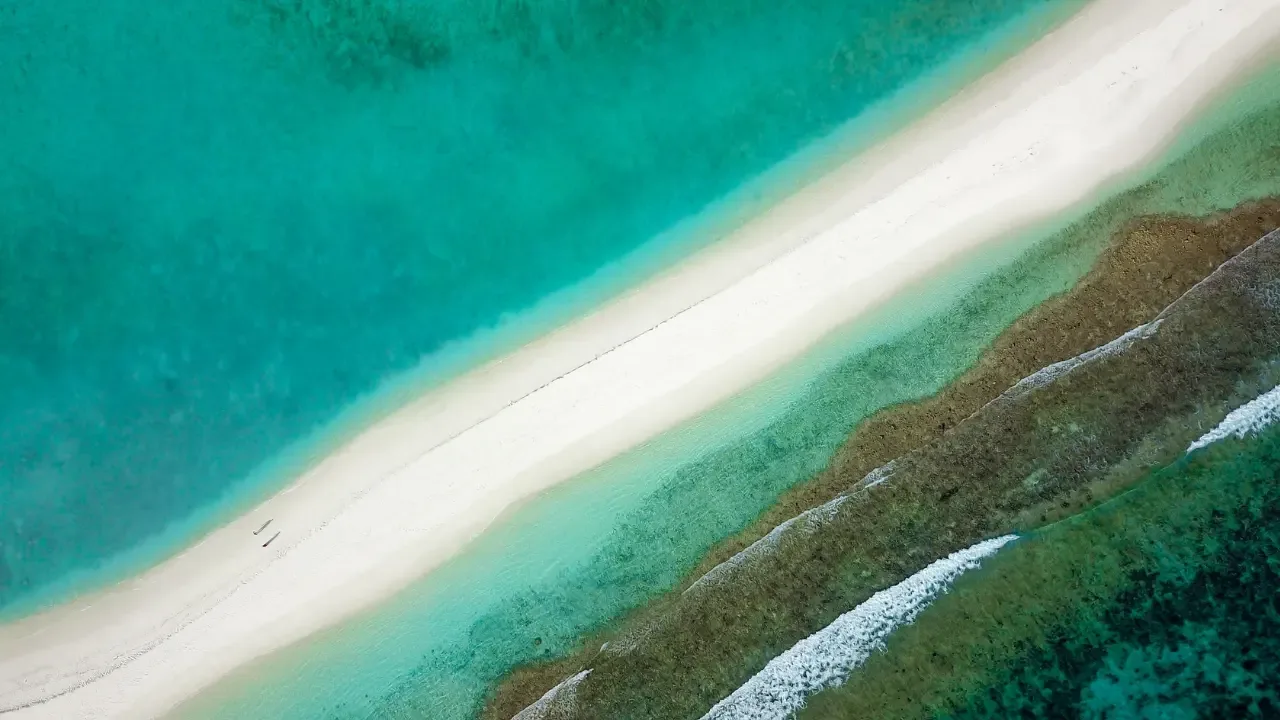
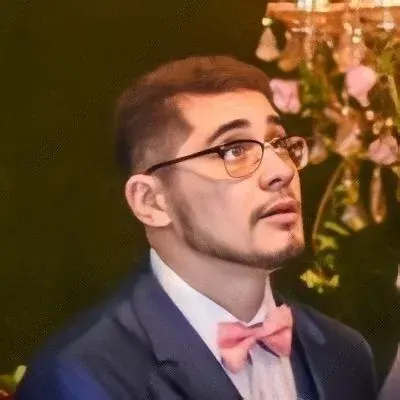
How to Replace a Character at a Specific Index in JavaScript? 😎
Are you stuck trying to figure out how to replace a character at a particular index in JavaScript? Don't worry, I've got you covered! 👍 In this guide, I'll walk you through the common issues people face and provide you with easy solutions to help you ace this task like a pro!
The Problem 🔍
Imagine you have a string like this: Hello world
, and you need to replace the character at index 3. In this case, the character is the letter "l" at index 3. How can you do this in JavaScript? Let's dive in!
The Solution 💡
JavaScript doesn't have a built-in replaceAt
function. However, you can easily achieve the desired result using a combination of string manipulation methods. Here's an example of how you can replace a character at a specific index:
var str = "hello world";
var newStr = str.substring(0, 3) + "X" + str.substring(4);
console.log(newStr);
In this solution, we're using the substring()
method to extract the parts of the string before and after the character we want to replace. We then add our new character (in this case, "X") in between and concatenate the resulting substrings. Voila! 🎉 You have successfully replaced the character at the desired index!
Handling Index Out of Bounds 🔒
It's important to note that when dealing with index-based operations, like replacing a character at a specific index, you should consider handling scenarios where the index is out of bounds.
Let's say you want to replace the character at index 10, but the string length is only 9 characters. You can easily handle this by checking if the index is within the valid range before performing the replacement. Here's an example:
function replaceAt(str, index, replacement) {
if (index < 0 || index >= str.length) {
return str;
}
return str.substring(0, index) + replacement + str.substring(index + 1);
}
var str = "hello world";
var newStr = replaceAt(str, 10, "X");
console.log(newStr);
By utilizing a custom replaceAt
function, we can now handle scenarios where the index is out of bounds gracefully, ensuring your application doesn't throw any unexpected errors.
Your Turn! ✨
Now that you know how to replace a character at a particular index in JavaScript, it's time to put your skills to the test! Try it out with different strings and varying index values to gain confidence and mastery over this technique. 💪
If you have any further questions or want to share your experience, I'd love to hear from you! Leave a comment below and let's keep the conversation going! Happy coding! 😄🚀