How do I remove a property from a JavaScript object?
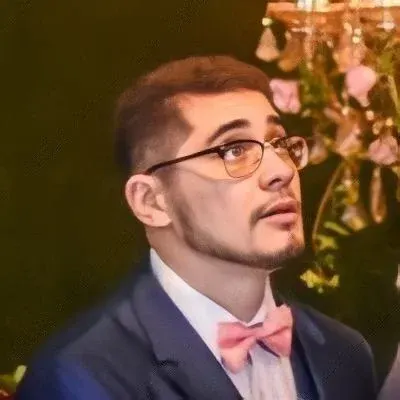
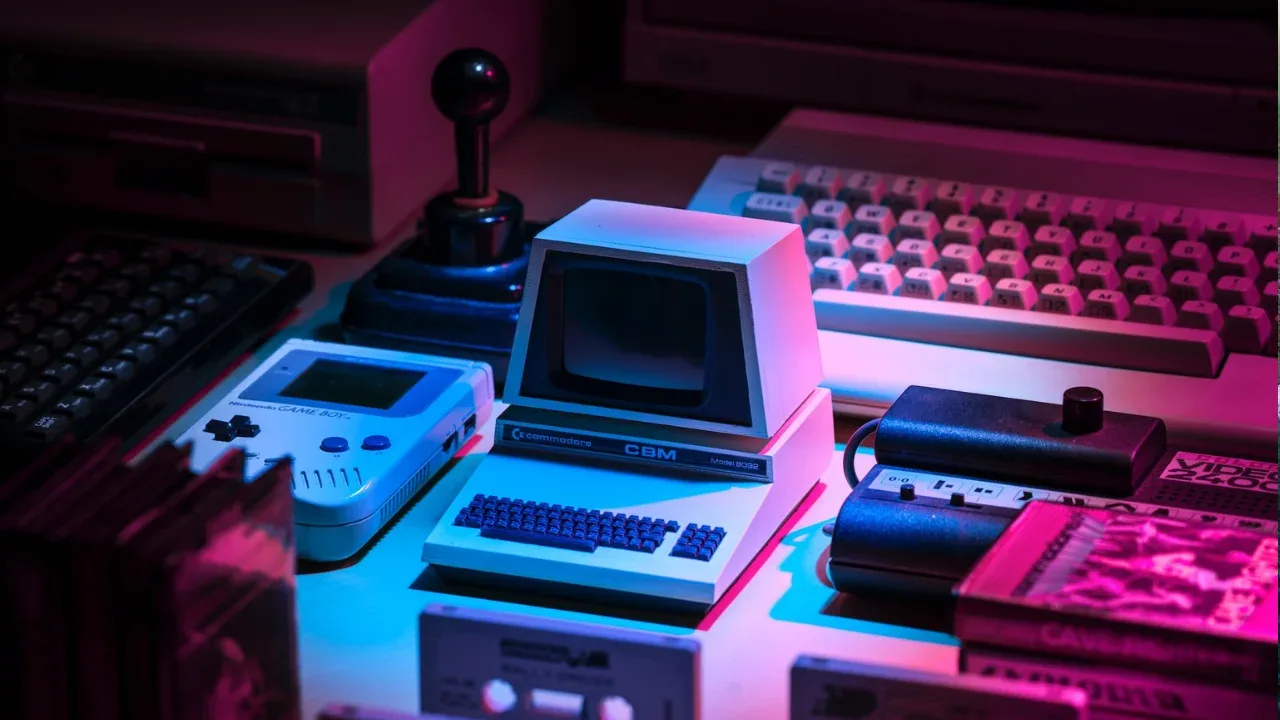
🚀 Removing a Property from a JavaScript Object: Easy Guide! 🚀
Have you ever found yourself in a situation where you need to remove a property from a JavaScript object? It can be confusing at first, but fear not! In this blog post, we'll walk you through common issues and provide easy solutions to help you achieve your goal.
Understanding the Problem
Let's start by looking at the context of the question. We have an object called myObject
, which looks like this:
let myObject = {
"ircEvent": "PRIVMSG",
"method": "newURI",
"regex": "^http://.*"
};
The goal is to remove the property regex
from myObject
, so it matches the following structure:
let myObject = {
"ircEvent": "PRIVMSG",
"method": "newURI"
};
Solution 1: Using the delete
operator
One way to remove a property from a JavaScript object is by using the delete
operator. It's a simple and straightforward solution.
Here's how you can do it:
delete myObject.regex;
By executing this line of code, the property regex
will be removed from the myObject
object. Simple as that! 🎉
Solution 2: Using the Object.assign()
method
Another approach you can take is to use the Object.assign()
method. This method can be used to copy or merge objects, but it can also be used to remove properties.
Here's how you can remove the regex
property using Object.assign()
:
myObject = Object.assign({}, myObject, { regex: undefined });
In this code snippet, we're creating a new object by merging the properties from myObject
with an object that has the regex
property set to undefined
. By doing this, we effectively remove the regex
property from myObject
.
Conclusion
Removing a property from a JavaScript object is not as challenging as it may seem. By using the delete
operator or the Object.assign()
method, you can easily achieve the desired result.
Remember, understanding the problem and using the right technique is key! Now it's your turn to give it a try. 🤓
Let us know in the comments which solution worked best for you or if you have any other cool JavaScript tricks to share. Happy coding! 💻🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
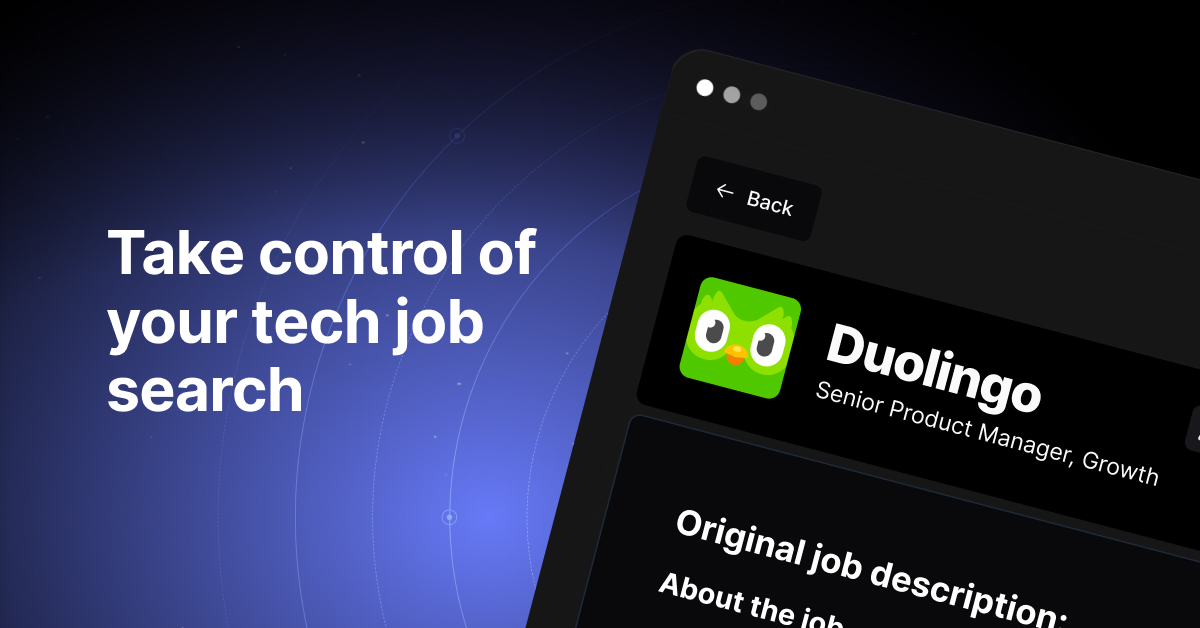