How do I remove a key from a JavaScript object?
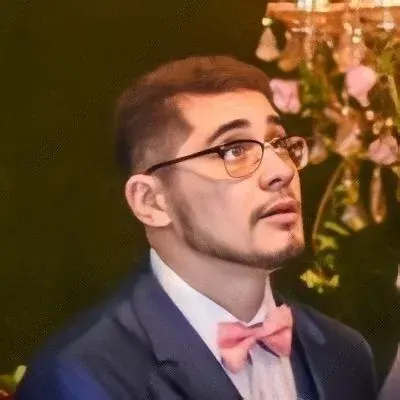
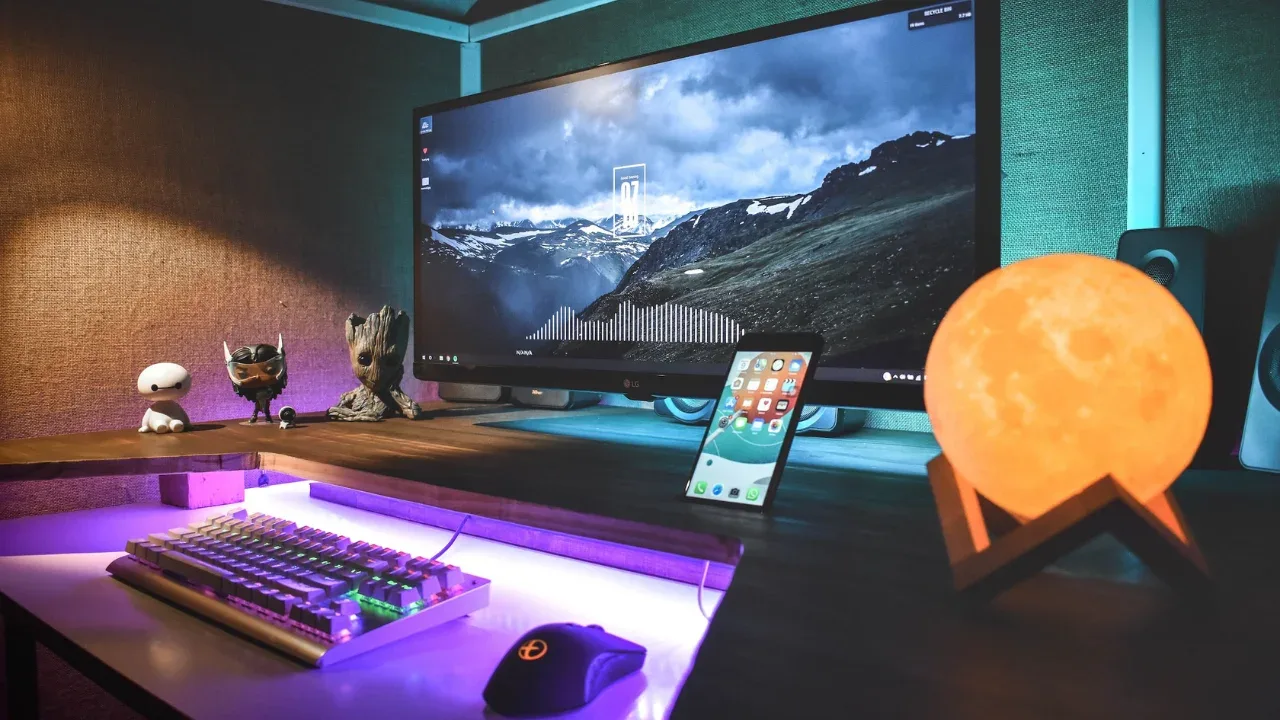
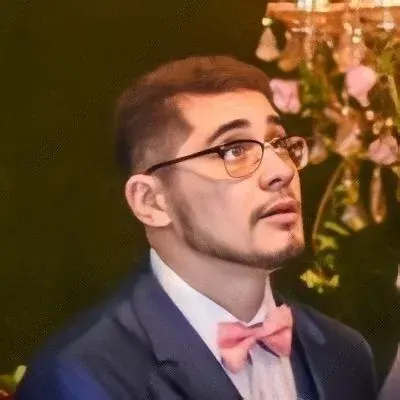
Easy Ways to Remove a Key from a JavaScript Object 🗝️
So, you want to remove a key from a JavaScript object? No worries, I've got you covered! 🤓
Understanding the Situation 💡
Let's start by understanding the problem. You have an object with some key-value pairs, and you need to remove a specific key. In your case, you want to remove the key 'Cow'
from the object:
var thisIsObject = {
'Cow' : 'Moo',
'Cat' : 'Meow',
'Dog' : 'Bark'
};
The Approach 🏃
We can achieve this in multiple ways, but I'll explain two easy and effective methods for removing a key from a JavaScript object.
Method 1: Using the delete
Operator 🗑️
The simplest way to remove a key is by using the delete
operator. Here's how you can do it:
delete thisIsObject['Cow'];
And just like that, the key 'Cow'
and its corresponding value 'Moo'
will be removed from the thisIsObject
object.
Method 2: Using the Object
method .assign()
📋
Another approach is to use the Object.assign()
method to create a new object without the specific key you want to remove. Here's how it works:
var updatedObject = Object.assign({}, thisIsObject);
delete updatedObject['Cow'];
In this approach, we create a new object updatedObject
by copying all the properties of thisIsObject
using Object.assign()
. Then, we use the delete
operator to remove the key 'Cow'
from updatedObject
. This way, the original thisIsObject
remains untouched, and you can work with the updated object instead.
The Call-to-Action 📣
Now that you know two methods to remove a key from a JavaScript object, it's time to put your new knowledge into practice! Try them out in your code and see which one works best for your particular situation. Feel free to experiment and have fun! 😄
If you have any other JavaScript related questions or need further assistance, don't hesitate to leave a comment below. I'm here to help! 👇
Happy coding! 💻🚀