How do I POST urlencoded form data with $http without jQuery?
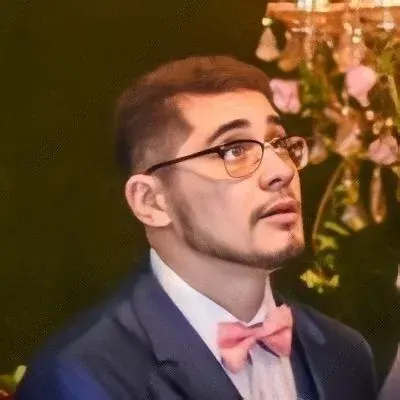
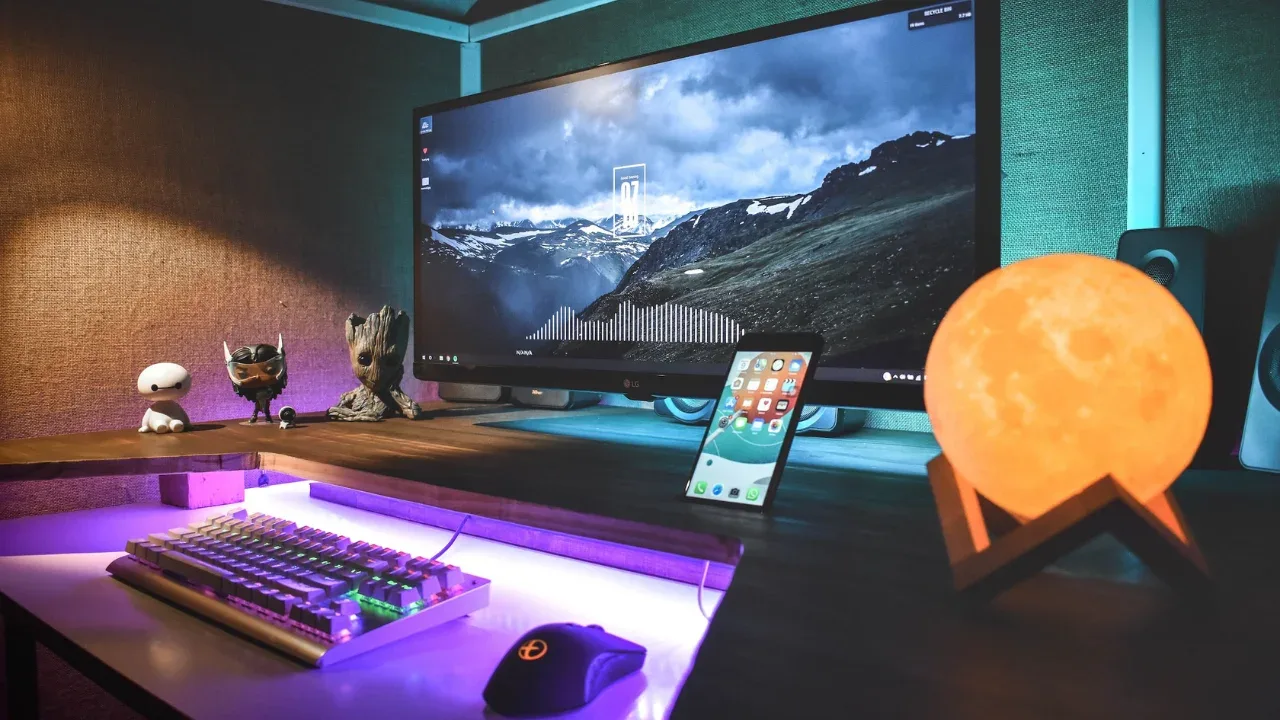
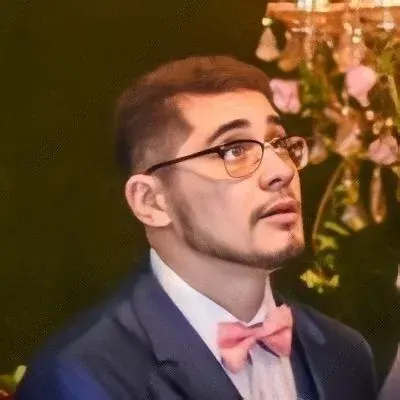
How to POST urlencoded form data with $http without jQuery?
š Hey there! So, you're new to AngularJS and eager to develop an application using just AngularJS. That's awesome! š
One common problem you might encounter is making an AJAX call to the server side using $http
from your Angular app. In particular, you want to send parameters in a urlencoded form data format. Let's walk through some possible solutions to this problem.
š Understanding the Issue
In your code snippet, you've successfully made the $http
POST request, but you relied on jQuery's $.param
function to urlencode the data. However, you want to avoid using jQuery and instead find a pure AngularJS solution.
š Solution 1: URLSearchParams API
One way to achieve urlencoding without jQuery is by using the URLSearchParams
API, which is built into modern browsers. Here's how you can modify your code:
var params = new URLSearchParams();
params.set('username', $scope.userName);
params.set('password', $scope.password);
$http({
method: "post",
url: URL,
headers: {'Content-Type': 'application/x-www-form-urlencoded'},
data: params.toString()
}).then(function(response){
console.log(response.data);
});
Using the URLSearchParams
API, you can easily set the parameters and then convert them to a string using the toString()
method. This way, you achieve the desired urlencoded form data without depending on jQuery.
š Solution 2: Manual serialization
If your target browser doesn't support the URLSearchParams
API, don't worry! You can still accomplish your goal by manually serializing the data. Here's how:
var data = 'username=' + encodeURIComponent($scope.userName) +
'&password=' + encodeURIComponent($scope.password);
$http({
method: "post",
url: URL,
headers: {'Content-Type': 'application/x-www-form-urlencoded'},
data: data
}).then(function(response){
console.log(response.data);
});
Here, encodeURIComponent()
is used to ensure that the parameter values are properly escaped for URL transmission.
š” Understanding the Differences and Choosing the Right Solution
Both solutions effectively send urlencoded form data with $http
without relying on jQuery. However, the first solution using the URLSearchParams
API is preferred when supported by the target browser, as it provides a cleaner and more elegant approach.
š£ Call-to-Action
Now that you have these solutions in your arsenal, feel free to experiment and find which one works best for your project. Remember, mastering AngularJS and its features will unlock numerous possibilities for your applications. If you have any other questions or face any challenges, leave a comment below, and let's continue this exciting AngularJS journey together! ššŖ
š References
Stay updated with more exciting tech blogs and insights by subscribing to our newsletter! š§š
Keep coding, keep learning! Happy coding! šāØ