How do I pass command line arguments to a Node.js program and receive them?
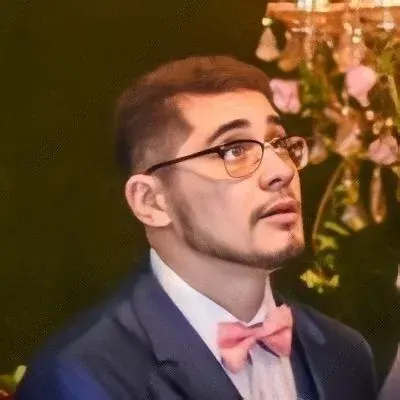
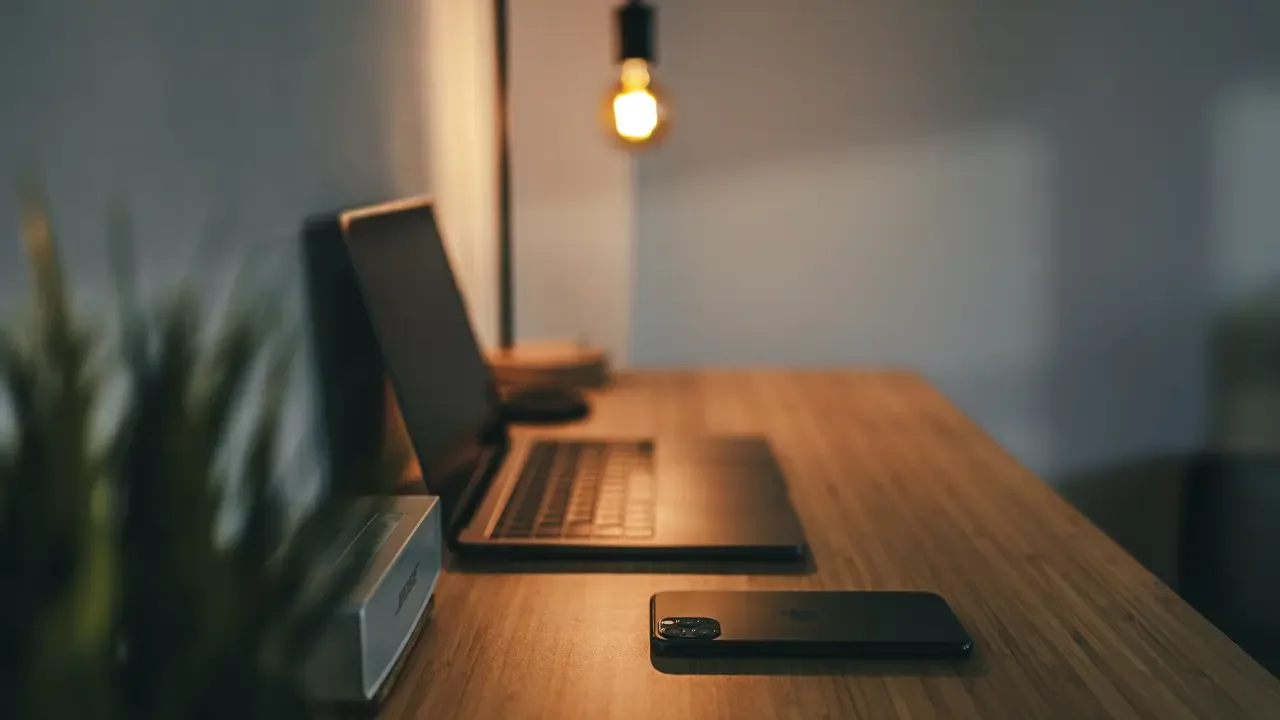
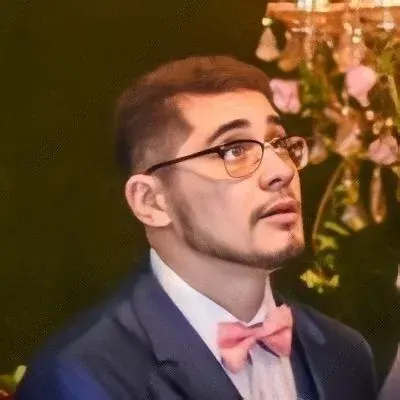
How to Pass Command Line Arguments to a Node.js Program 🚀
So, you're building a web server in Node.js and you want to be able to launch it with specific arguments from the command line? No worries, I've got you covered! 🎉
Understanding the Problem 🤔
The user here wants to pass a folder argument when launching their Node.js server using the command node server.js folder
. However, they're not sure how to access these arguments in their JavaScript code. Don't fret, because it's actually quite simple! 💪
Easy Solution 🙌
To access command line arguments in a Node.js program, you can leverage the process.argv
array. This array contains the command line arguments provided when launching your Node.js script. Here's how you can make use of it:
// server.js
// The first two elements of process.argv are the Node.js executable and the name of your script respectively.
// The third element onwards contain the command line arguments.
const folder = process.argv[2];
console.log(`Launching server with folder: ${folder}`);
// Do whatever you want with the folder argument here
Now, when you run node server.js folder
, the value of folder
will be set to "folder"
. You can use it as needed within your server code. Easy peasy! 😎
Dealing with Edge Cases 👀
It's important to handle scenarios where the user forgets to provide the required argument. In our case, if no folder argument is provided, accessing process.argv[2]
will result in undefined
. You can easily address this by adding a check:
// server.js
const folder = process.argv[2];
if (folder) {
console.log(`Launching server with folder: ${folder}`);
// Do whatever you want with the folder argument here
} else {
console.error("Please provide the required folder argument!");
}
🚀 Time to Launch Your Awesome Server!
Now that you know how to pass command line arguments to your Node.js server, it's time to take your project to the next level! 🚀 Whether you want to customize the behavior of your server or dynamically configure settings, command line arguments can help you achieve that flexibility.
So go ahead, experiment with different arguments, and make your server even cooler! And if you have any other questions or tips to share, don't forget to drop them in the comments below. Let's learn and grow together! 💡🌱
Keep coding, keep exploring! 👩💻👨💻