How do I make the first letter of a string uppercase in JavaScript?
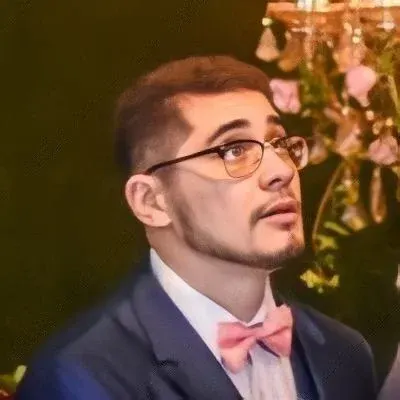
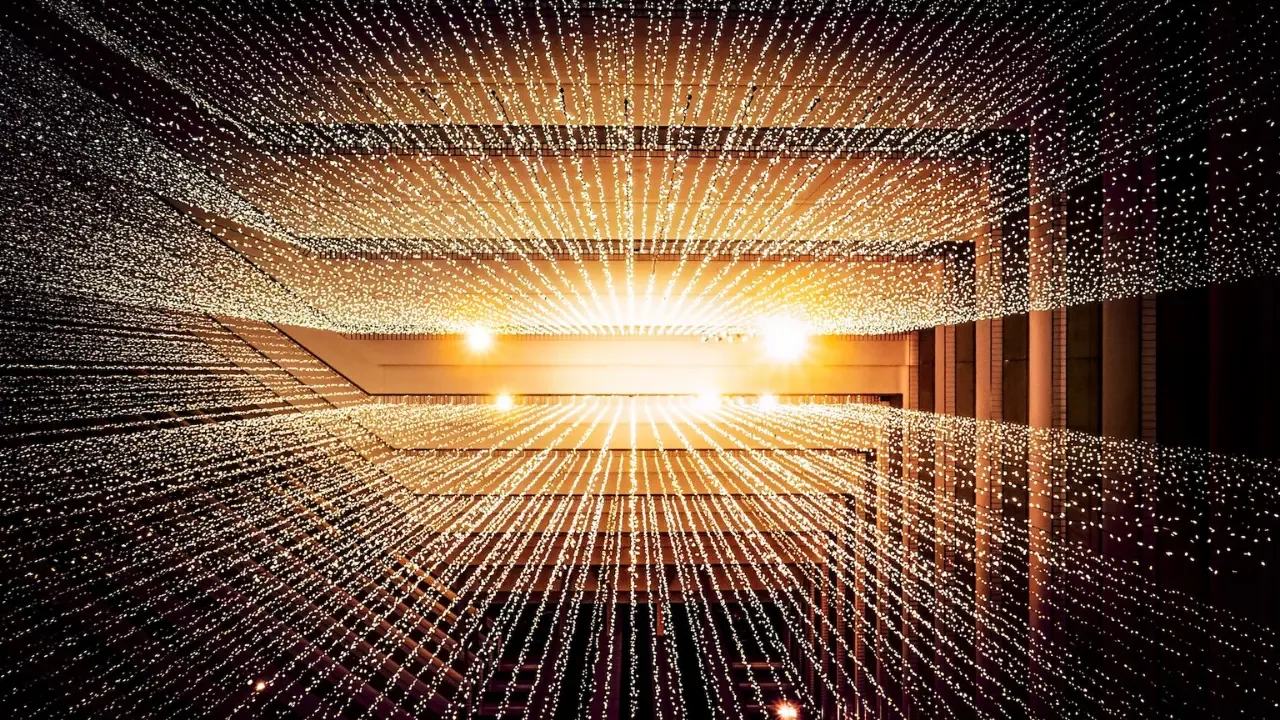
How to Make the First Letter of a String Uppercase in JavaScript
π Welcome to our tech blog! Today, we have an interesting question that many developers often come across: "How do I make the first letter of a string uppercase in JavaScript?" π€
The Challenge: Only Capitalize the First Letter
So, here's the problem: you have a string, but you want to capitalize only the first letter of that string. But wait, there's a catch! You don't want to change the case of any of the other letters in the string. Sounds tricky, right? Let's dig into some easy solutions. π‘
1. Using JavaScriptβs String Methods
JavaScript provides us with some handy string methods that can help us achieve this task without pulling our hair out. One such method is charAt()
which returns the character at a specified index in a string.
Here's a simple solution:
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
console.log(capitalizeFirstLetter("this is a test")); // Output: "This is a test"
console.log(capitalizeFirstLetter("the Eiffel Tower")); // Output: "The Eiffel Tower"
console.log(capitalizeFirstLetter("/index.html")); // Output: "/index.html"
In this solution, we use charAt(0)
to get the first character of the string. Then, we use toUpperCase()
to convert that first character to uppercase. Finally, we use slice(1)
to get the rest of the string starting from the second character and concatenate it with the uppercase first character.
Voila! Now you have the first letter capitalized.
2. Using Regular Expressions
If you're a fan of regular expressions (or want to impress your developer friends), we have another solution for you. π¦
function capitalizeFirstLetter(string) {
return string.replace(/^[a-z]/, (match) => match.toUpperCase());
}
In this solution, we use the replace()
method with a regular expression /^[a-z]/
, which matches the first lowercase letter in the string. Then, we pass an arrow function as the replacement parameter to the replace()
method. Inside this function, we use toUpperCase()
to convert the matched letter to uppercase.
You're just one line of code away from better strings! πͺ
Now that you have not one but two easy solutions to capitalize the first letter of a string, go ahead and try them out in your own JavaScript projects. Impress your colleagues, friends, and the developer community with your newfound knowledge! π
We hope you found this guide useful! If you have any questions or other JavaScript topics you'd like us to cover, feel free to drop a comment below. Happy coding! π»β¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
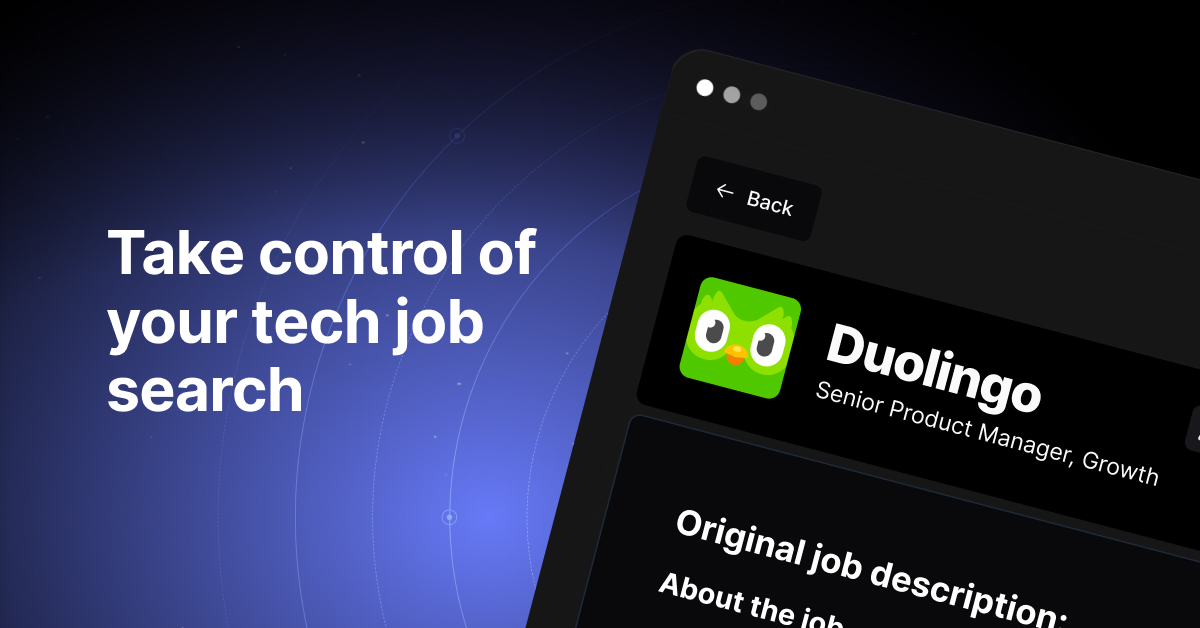