How do I loop through or enumerate a JavaScript object?
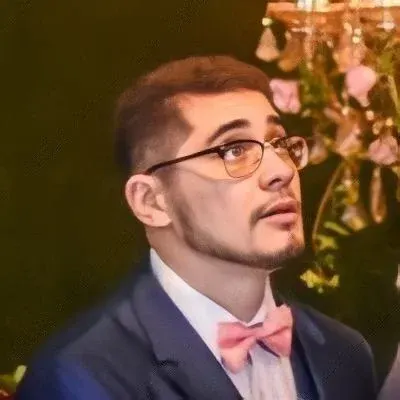
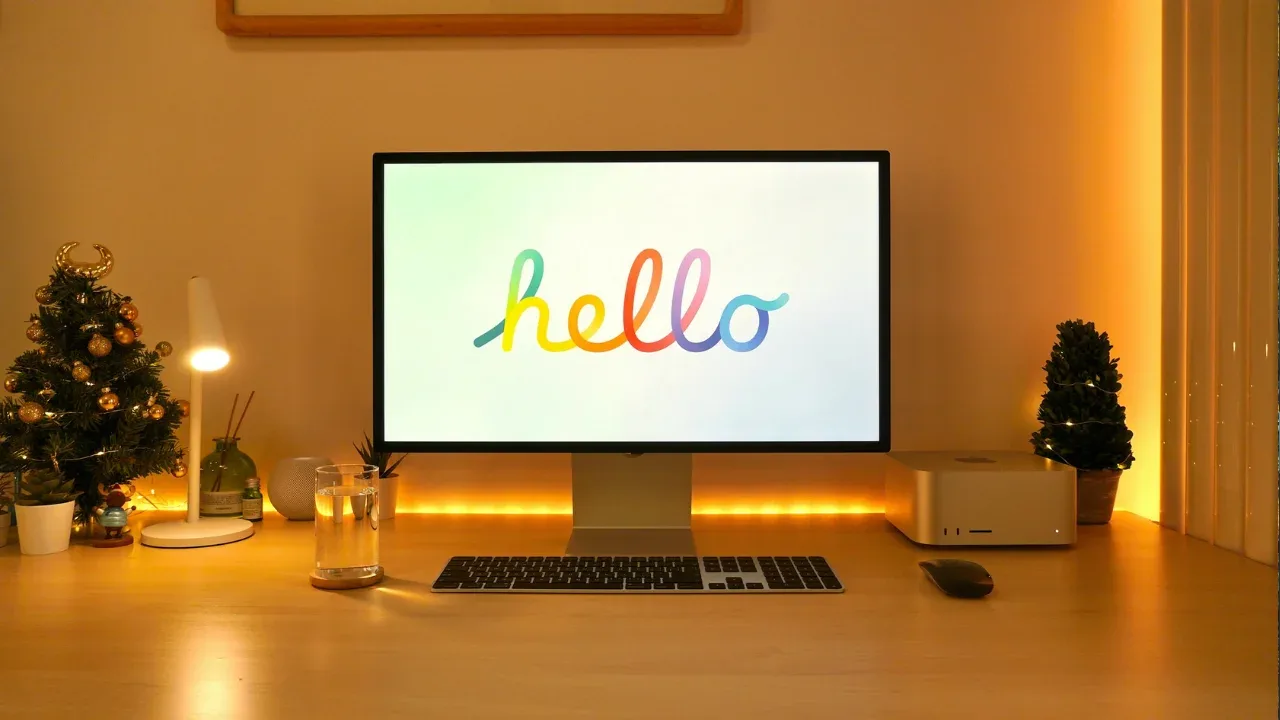
How to Loop Through or Enumerate a JavaScript Object 🔄🔢
Are you struggling with looping through or enumerating a JavaScript object? 🤔 Don't worry, we've got you covered! In this guide, we'll address this common problem and provide you with easy solutions so you can conquer it like a pro. Let's get started! 💪
The Scenario 📖
Imagine you have a JavaScript object like this:
var p = {
"p1": "value1",
"p2": "value2",
"p3": "value3"
};
And you want to loop through all of p
's elements (p1
, p2
, p3
) to get their keys and values. So, let's dive into the solutions for this challenge! 🚀
Solution 1: Using a for...in Loop 💫
One way to loop through the properties of an object is by using a for...in
loop. This loop will iterate over each key in the object, allowing you to access the corresponding value. Here's how you can accomplish this:
for (var key in p) {
if (p.hasOwnProperty(key)) {
var value = p[key];
console.log("Key: ", key);
console.log("Value: ", value);
}
}
In this code snippet, we're using the hasOwnProperty
method to filter out inherited properties. This ensures that we only loop through the object's own properties.
Solution 2: Object.entries() Method 🗂
Another modern approach to looping through an object is by using the Object.entries()
method. This method returns an array of arrays, where each sub-array contains a key-value pair from the object. Here's how you can utilize this method:
Object.entries(p).forEach(([key, value]) => {
console.log("Key: ", key);
console.log("Value: ", value);
});
Using the destructuring assignment in the forEach
callback allows us to directly access the key
and value
from the sub-array.
Solution 3: Object.keys() Method 🗝
If you're only interested in the keys of the object, you can use the Object.keys()
method. This method returns an array containing all the keys of the object, which you can then loop through or manipulate as needed. Here's an example:
Object.keys(p).forEach((key) => {
console.log("Key: ", key);
console.log("Value: ", p[key]);
});
This solution is especially useful if you don't need to access the values directly but want to perform operations solely on the keys.
Conclusion and Call-to-Action 🎉💬
You've reached the end of our guide to looping through or enumerating a JavaScript object! 🙌 We hope you found these solutions helpful and can now tackle this challenge with ease.
Remember, the for...in
loop, Object.entries()
, and Object.keys()
are powerful tools in your JavaScript arsenal. Depending on your specific use case, choose the solution that best suits your needs.
Now it's your turn to try them out! Share your experience or any other tips and tricks you've discovered in the comments section below. Let's keep the conversation going! 💬💡
Happy coding! 🚀🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
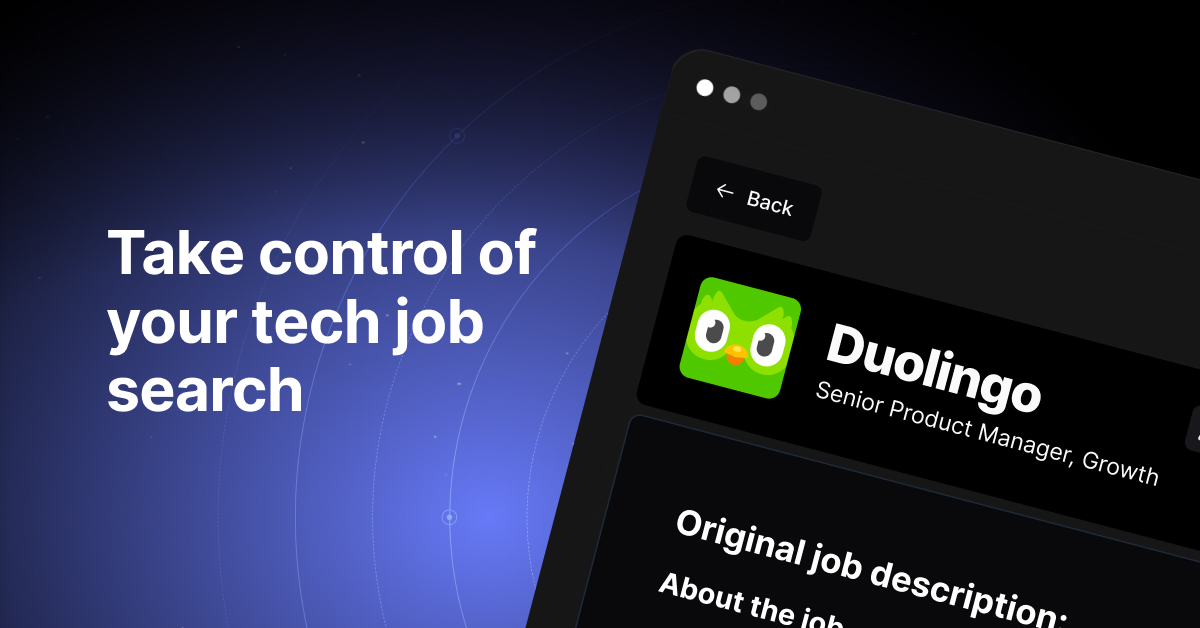