How do I include a JavaScript file in another JavaScript file?
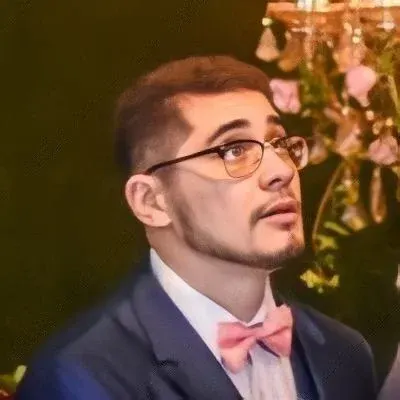
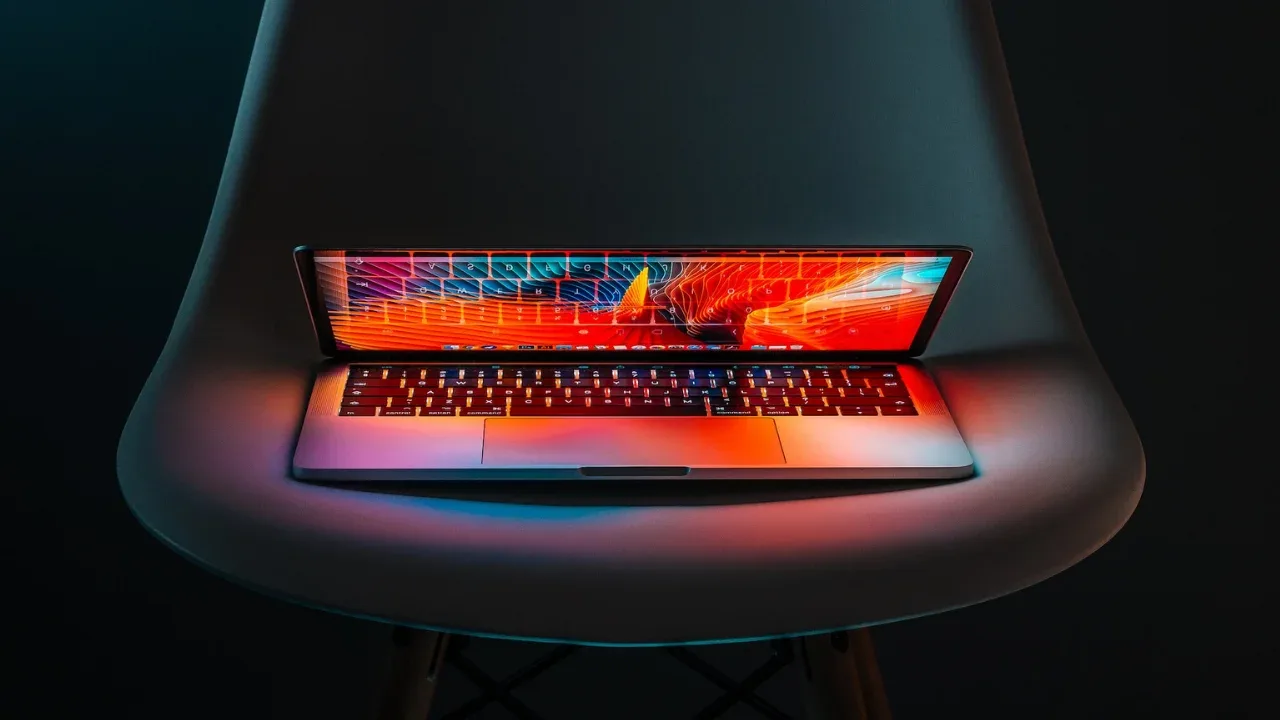
How to Include a JavaScript File in Another JavaScript File
š Hey there tech-savvy peeps! So, you've got a burning desire to include a JavaScript file inside another JavaScript file, just like how we do it with @import
in CSS? I've got your back! Let's dive into this tech dilemma and find an easy solution together. šŖ
Understanding the Issue
š¤ Before we jump into the solution, let's make sure we understand the problem. You probably want to use some functions, variables, or objects defined in one JavaScript file in another one, right? We've all been there! Unfortunately, JavaScript does not have a built-in @import
feature like CSS. But fear not, fellow developer, because we have some awesome alternatives. š
Solution 1: The Good Ol' Script Tag
š·ļø The simplest and most widely-used solution is to use the <script>
tag to include the desired JavaScript file inside the HTML file that references the main JavaScript file. Here's an example:
<!DOCTYPE html>
<html>
<head>
<title>My Awesome Website</title>
<script src="main.js"></script> <!-- Include your main JavaScript file -->
</head>
<body>
<!-- The rest of your HTML content -->
<script src="other.js"></script> <!-- Include the JavaScript file you want to use -->
</body>
</html>
š In the example code above, we include the other.js
file after the main.js
file inside the HTML file. This allows the functions, variables, or objects defined in other.js
to be accessible from within main.js
. Easy peasy, right? š
Solution 2: Using the Module System
š If you're already using modern JavaScript features, like ES6 modules, then you're in luck! The module system provides a much cleaner and modular approach to including JavaScript files. Here's how you can use it:
<!DOCTYPE html>
<html>
<head>
<title>My Awesome Website</title>
<script type="module">
import { someFunction } from './main.js'; // Import the desired function from main.js
import { anotherFunction } from './other.js'; // Import the desired function from other.js
// Start using the imported functions
someFunction();
anotherFunction();
</script>
</head>
<body>
<!-- The rest of your HTML content -->
</body>
</html>
š In the example code above, we use the import
statement to include the desired functions from the respective JavaScript files. Just make sure you have defined those functions as exports in their respective files. Modules make it easy to manage dependencies and keep your code organized. š
Conclusion and Call-to-Action
š And there you have it, my tech-savvy amigos! You now know not just one, but two awesome ways to include a JavaScript file inside another JavaScript file. Whether you go for the traditional script tag or embrace the modern module system, you're bound to find a solution that suits your coding style. š»
š” If this guide helped you in any way, share it with your fellow developers who might be facing the same conundrum. And don't forget to leave a comment below! I'd love to hear your thoughts and experiences with including JavaScript files. Let's geek out together! š¤
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
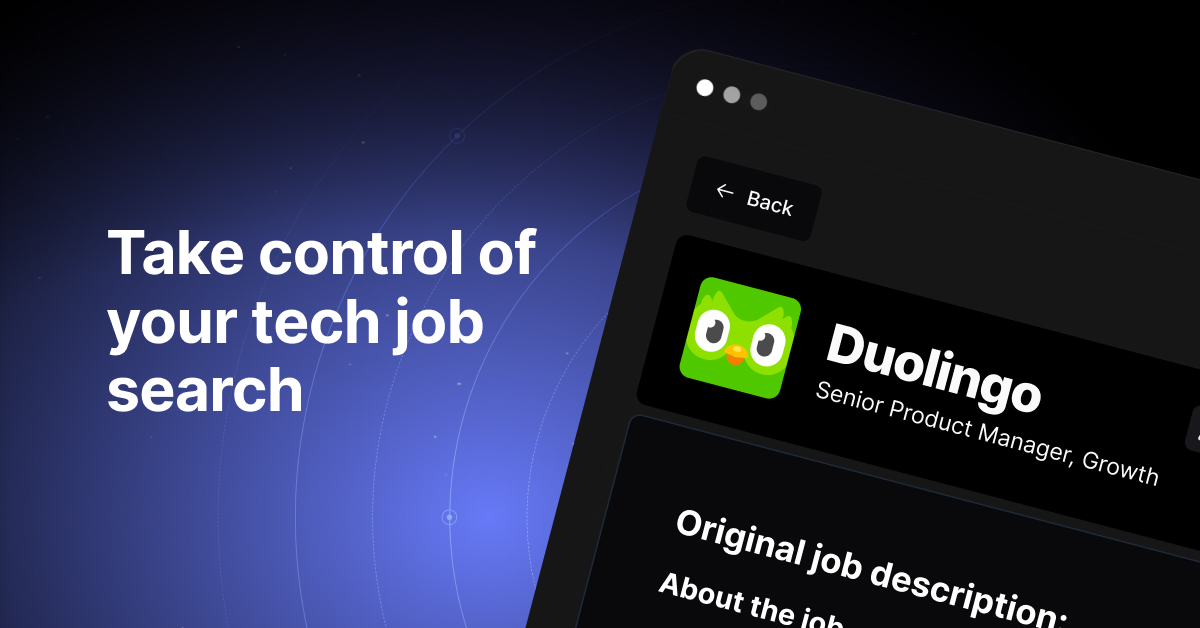