How do I handle newlines in JSON?
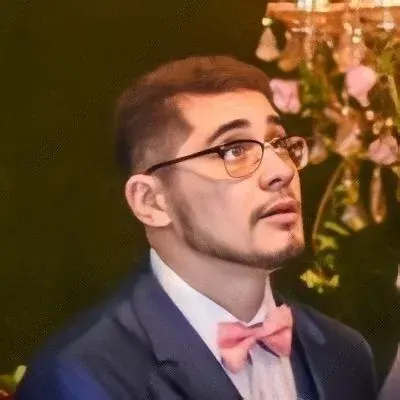
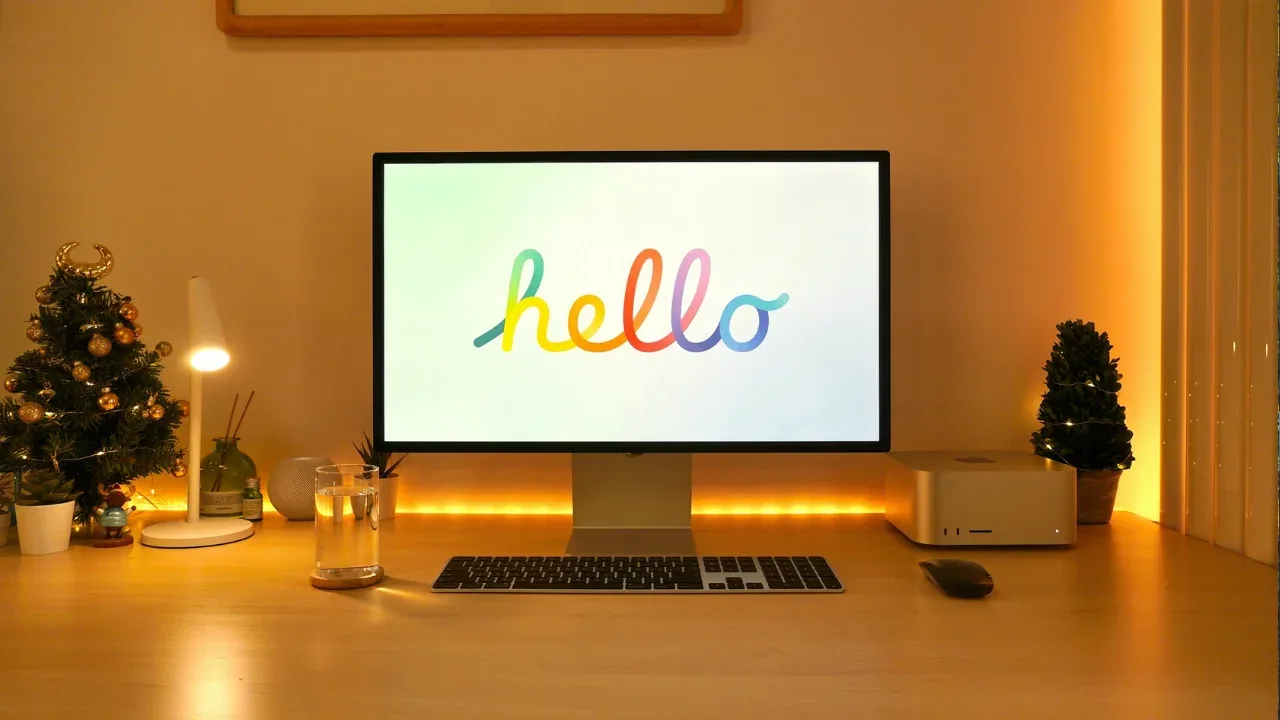
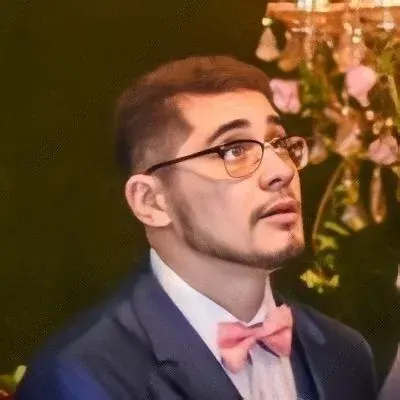
How to Handle Newlines in JSON: A Simple Guide ๐๐
So, you're working with JSON and you've encountered a little problem with newlines. Don't worry, you're not alone! It's a common issue that can lead to frustrating errors. But fear not, we've got you covered with some easy solutions. Let's dive in! ๐โโ๏ธ
The Problem: Newlines Wreaking Havoc ๐คฏ
Let's take a look at the code snippet you provided:
var data = '{"count" : 1, "stack" : "sometext\n\n"}';
var dataObj = eval('('+data+')');
This code generates a JSON string with newlines and attempts to convert it into a JavaScript object using eval()
. However, you're facing an "unterminated string literal" error. The same error pops up when using JSON.parse(data)
with the additional issue of "Unexpected token โต" in Chrome and "unterminated string literal" in Firefox and IE. So, what's going on? ๐ค
Explaining the Issue: Escaping Characters! ๐ฉโ๐ซ๐
The problem lies in how characters are escaped in JSON strings. In JSON, the backslash \
is used to escape special characters like newline (\n
), tab (\t
), and double quotes (\"
). However, when using eval()
or JSON.parse()
, the backslash is treated as an escape character by JavaScript itself, not just JSON.
In our case, the newline character \n
is causing trouble. JavaScript interprets it as an actual newline, resulting in an "unterminated string literal" error. This is why removing the \n
resolves the issue.
Solution 1: Removing Newlines Manually ๐
The simplest solution is to remove the offending newline characters from your JSON string. Here's an updated version of your code:
var data = '{"count" : 1, "stack" : "sometext"}'; // Removed the \n after "sometext"
var dataObj = eval('('+data+')');
By manually removing the \n
, you avoid the error and successfully convert your JSON into a JavaScript object. Problem solved! ๐
Solution 2: Escaping the Backslashes ๐งโจ
If removing newlines is not an option for some reason, you can escape the backslashes themselves. Here's how it looks:
var data = '{"count" : 1, "stack" : "sometext\\n\\n"}'; // Escaped the \n with double backslashes
var dataObj = JSON.parse(data);
By using double backslashes \\
before the newline \n
, you ensure that JavaScript treats them as literal characters rather than escape sequences.
Try It Yourself! ๐ป
To put these solutions into practice, copy the code snippets provided and give them a try in your own development environment. Play around with different scenarios and understand how newlines affect your JSON parsing.
Join the Conversation! ๐ฌ๐ข
We hope this guide helped you solve your newline issue in JSON! If you have any questions, comments, or alternative solutions, we'd love to hear from you. Leave a comment below to share your thoughts and engage with fellow developers. Let's make handling newlines in JSON a breeze for everyone! ๐
Happy Coding! ๐๐ป
Now that you're equipped to handle newlines in JSON like a pro, go forth and code with confidence! Don't let those pesky newlines stand in your way. Happy coding and may your JSON parsing adventures be trouble-free! ๐๐ฅ