How do I get the YouTube video ID from a URL?
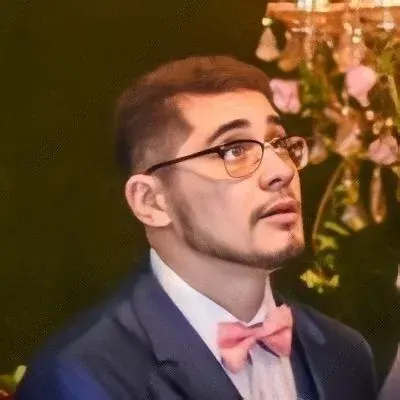
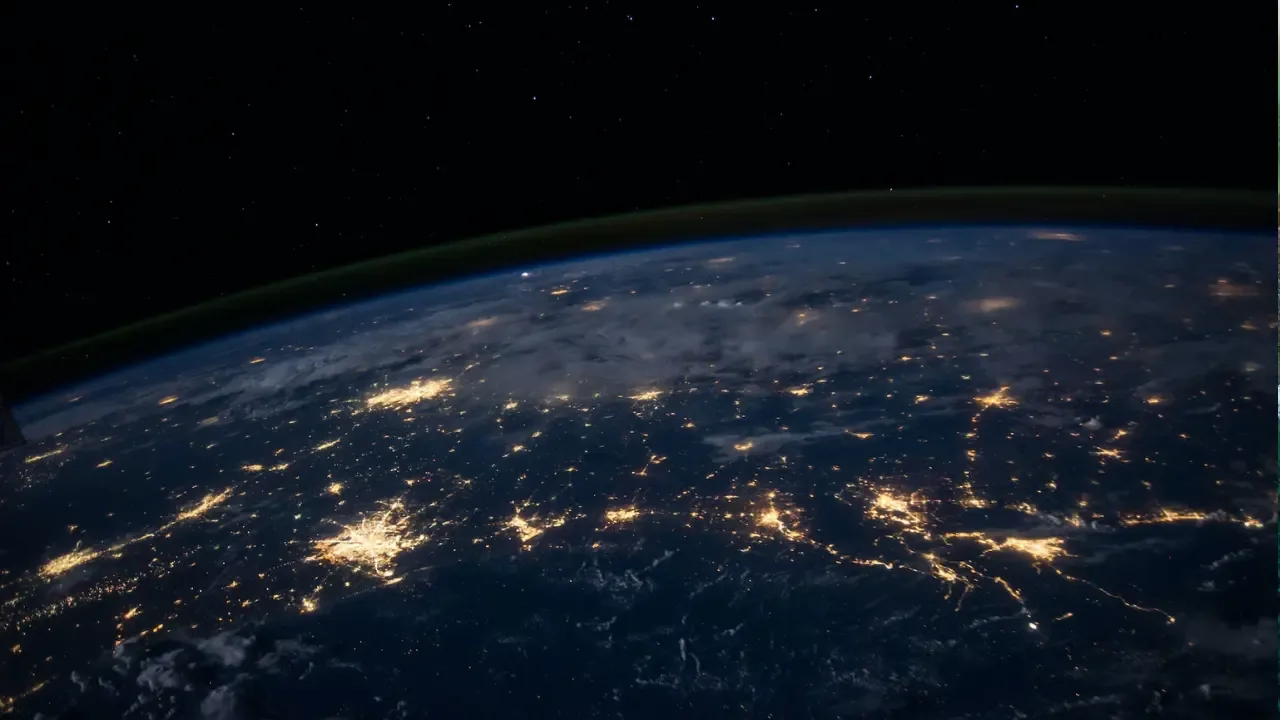
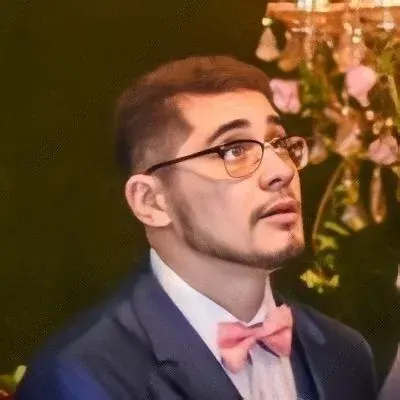
How to Get the YouTube Video ID from a URL 📹
Are you looking to extract the YouTube video ID from a URL using JavaScript? Look no further! In this easy-to-follow guide, we'll walk you through the process step-by-step, addressing common issues and providing solutions.
Understanding YouTube URL Formats 🌐
YouTube URLs can be in different formats, but they all contain the video ID that we're after. Here are a few examples:
http://www.youtube.com/watch?v=u8nQa1cJyX8&a=GxdCwVVULXctT2lYDEPllDR0LRTutYfW
http://www.youtube.com/watch?v=u8nQa1cJyX8
Regardless of the format, our goal is to extract the video ID, which in this case is u8nQa1cJyX8
. Let's dive into the solutions!
Solution 1: Using Regular Expressions 🎯
One way to get the YouTube video ID from a URL is to use regular expressions in JavaScript. Regular expressions are powerful tools for pattern matching. Here's an example of how you can extract the video ID using this method:
function getVideoId(url) {
const regex = /(?:\?v=|&v=|youtu\.be\/|\/embed\/|\/v\/|\.be\/)([^#\&\?]{11})/;
const match = url.match(regex);
if (match && match[1]) {
return match[1];
} else {
// Video ID not found
return null;
}
}
const url = "http://www.youtube.com/watch?v=u8nQa1cJyX8";
const videoId = getVideoId(url);
console.log(videoId); // Output: u8nQa1cJyX8
This solution works for most YouTube URL formats. However, keep in mind that YouTube might change their URL format in the future, which could break this regular expression. Regular expressions can also be challenging to understand and maintain.
Solution 2: Using URLSearchParams 🌐
If you prefer a simpler and more modern approach, you can use the URLSearchParams
API available in most modern browsers. Here's an example:
function getVideoId(url) {
const searchParams = new URLSearchParams(new URL(url).search);
return searchParams.get("v");
}
const url = "http://www.youtube.com/watch?v=u8nQa1cJyX8";
const videoId = getVideoId(url);
console.log(videoId); // Output: u8nQa1cJyX8
The URLSearchParams
API allows you to parse the URL's query string easily and retrieve the v
parameter, which corresponds to the video ID.
Conclusion and Call-to-Action 🚀
Now that you know how to extract the YouTube video ID from a URL, you can incorporate this functionality into your web projects with ease. Whether you choose the regular expression approach or the URLSearchParams
API method, these solutions will help you trustfully retrieve the video ID.
Try implementing these solutions in your next project and share your experience with us! If you have any other questions or need further assistance, feel free to leave a comment below. Happy coding!
👉 Follow us on Twitter for more helpful tech tips and guides! 👈