How do I get the current date in JavaScript?
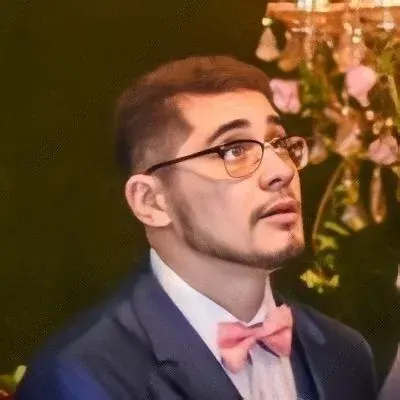
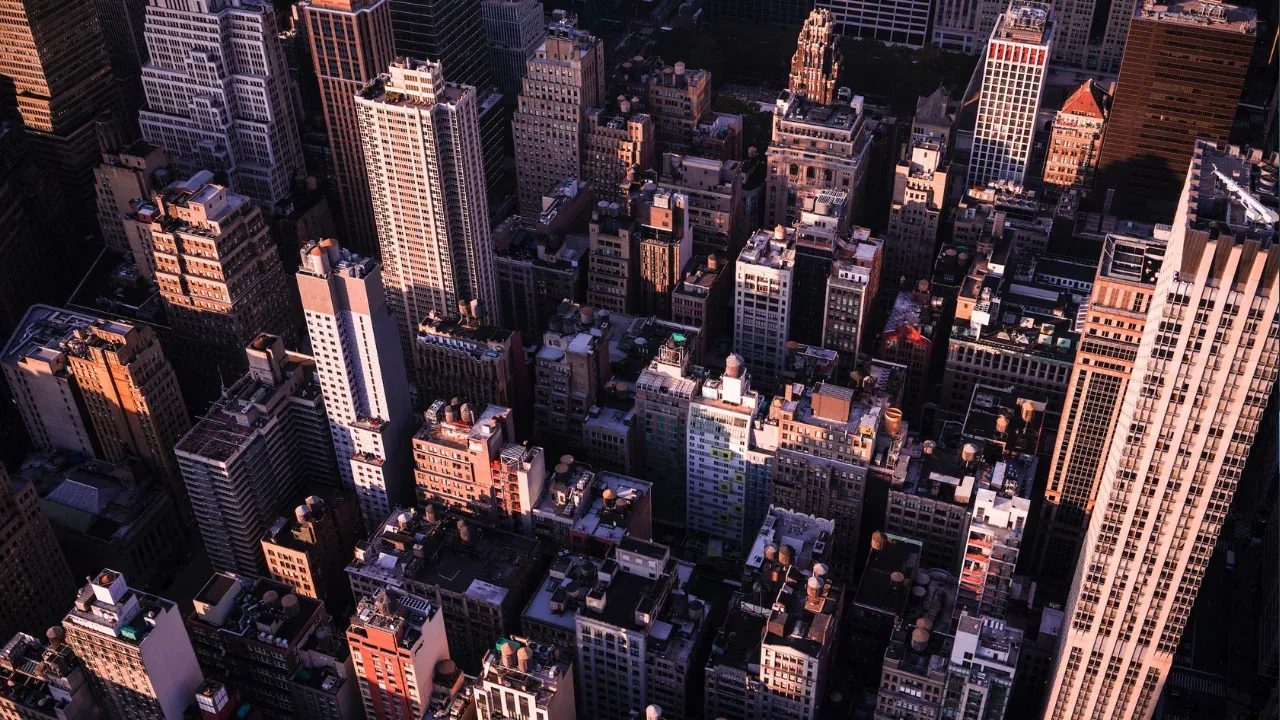
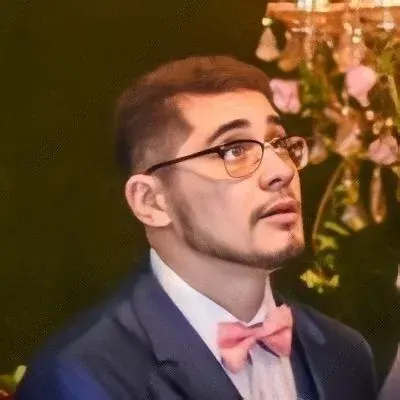
How to Get the Current Date in JavaScript: A Handy Guide! 📅🚀
So, you want to know how to get the current date in JavaScript? 🤔 You've come to the right place! JavaScript provides various methods to obtain the current date and time, and in this guide, we'll dive into a few different approaches. By the end, you'll be a JavaScript date expert! 💪
Option 1: The Date Object 🗓️
The simplest way to get the current date in JavaScript is by using the Date
object. Here's an example:
const currentDate = new Date();
console.log(currentDate);
Running this code snippet will output the current date and time in your browser's console. This method allows you to work with the date object further, extract specific elements such as year, month, day, hours, minutes, and seconds, and perform various operations on them.
Option 2: Getting the Date as a String 📅🔤
Sometimes, you might need the current date in a specific format as a string. JavaScript provides several methods to achieve this. Let's take a look at a couple of them:
Method 1: Using toLocaleDateString()
const options = { year: 'numeric', month: 'long', day: 'numeric' };
const currentDate = new Date().toLocaleDateString(undefined, options);
console.log(currentDate);
This code snippet would yield a result like "September 25, 2022".
Method 2: Using toISOString()
const currentDate = new Date().toISOString().split('T')[0];
console.log(currentDate);
With this code, you'll get a result in the format "2022-09-25".
Feel free to experiment with these methods and tweak the options to suit your desired format!
Common Issues and Troubleshooting ✋🐛
1. Wrong Timezone
It's important to note that the methods discussed above will return the current date and time according to your device's timezone. If you need to display the date in a different timezone, you can use libraries like Moment.js or Moment Timezone to handle those conversions easily.
2. Date Formatting
Sometimes, you might find yourself struggling to achieve the exact date format you desire. In such cases, take a look at the MDN documentation on the Date object or explore popular libraries like Luxon or Day.js, which offer powerful and flexible date formatting options.
Your Turn to Shine! ✨🎉
Congratulations! 🎉 You've learned how to obtain the current date in JavaScript using both the Date
object and different formatting methods. Now, it's time to put your knowledge into action!
Exercise: Building a Countdown Timer ⏳
To practice what you've learned, why not challenge yourself to build a countdown timer using JavaScript? Implement a webpage that displays the number of days, hours, minutes, and seconds until an upcoming event using the current date functionality we covered. 🗓️⏱️
Feel free to get creative with the design, add personalized features, and most importantly, have fun learning and improving your JavaScript skills! Remember to share your creations and tag us on social media - we can't wait to see what you come up with! 😉
Happy coding! 💻✨