How do I get a timestamp in JavaScript?
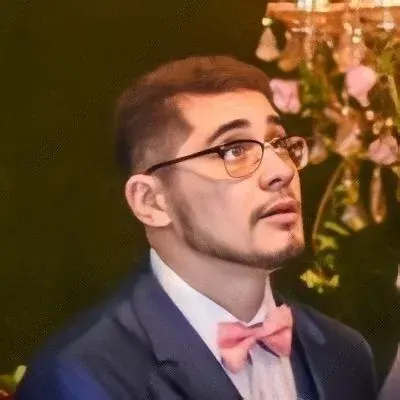
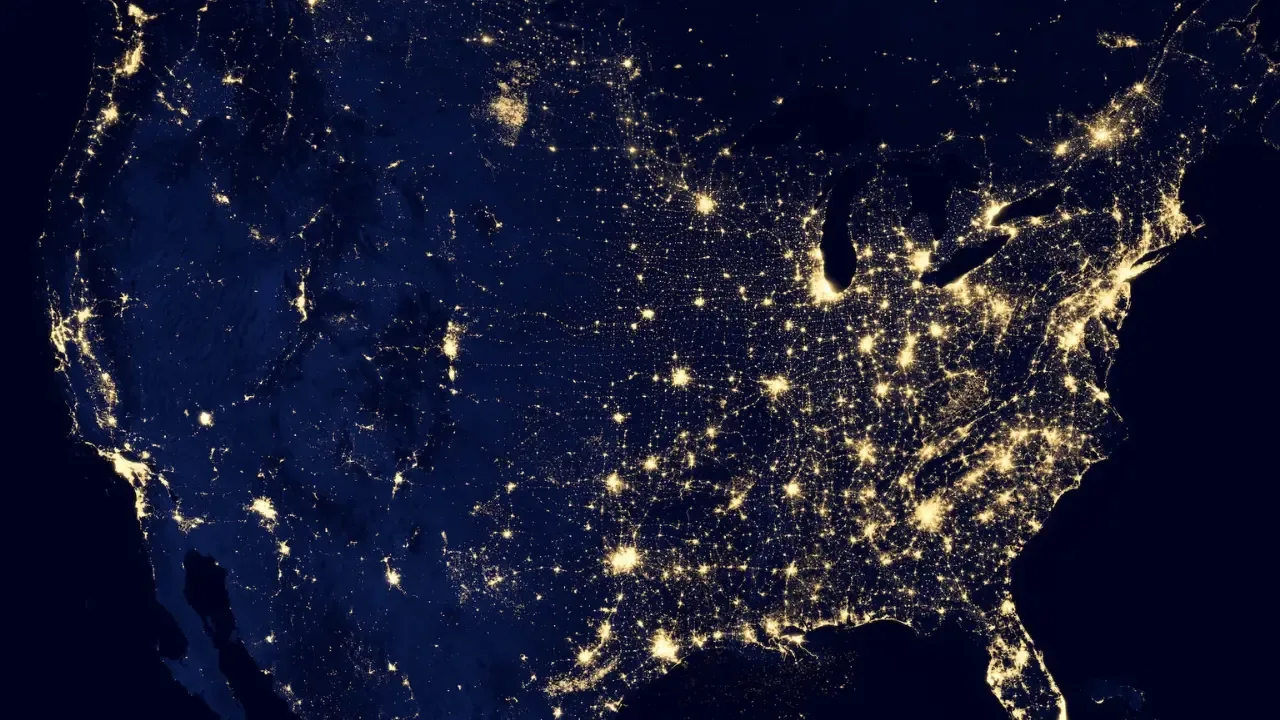
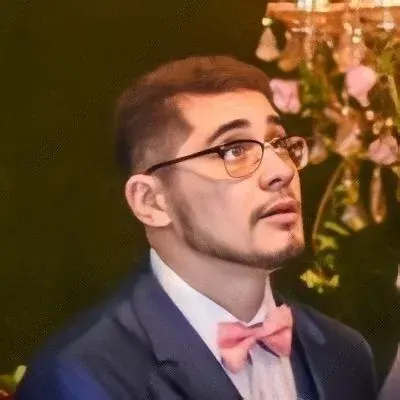
How to Get a Timestamp in JavaScript: Your Ultimate Guide! ⏱️📅
Hey there, coding enthusiasts! Have you ever wondered how to get a timestamp in JavaScript? 🤔 Well, you've come to the right place!
Whether you're building a sleek countdown timer ⏳ or tracking the time of a game event 🎮, having a timestamp can be incredibly useful. But fear not, we're here to help you master this trick! Let's dive right in 🏊♀️:
What is a Timestamp and Why Do You Need It?
A timestamp is a unique identifier that represents a specific point in time. It's typically used to measure the time elapsed between events or accurately display when something occurred. 💡
Imagine you want to record when a user signed up on your website 📝. Instead of displaying the date and time in a human-readable format, you can convert it into a timestamp. This allows you to perform calculations and manipulate the time effortlessly. Pretty neat, huh? ✨
Getting the Current Timestamp
So how do we obtain this mystical timestamp in JavaScript? Fear not, for we have a few options up our sleeves! 🎩
Option 1: Using the Date
Object
The Date
object in JavaScript comes to the rescue! 🚀 By default, it provides the current date and time. To get the timestamp, we can use the getTime()
method like this:
const timestamp = new Date().getTime();
console.log(timestamp);
Here, we create a new Date
object and call the getTime()
method on it. This returns the number of milliseconds that have passed since January 1, 1970, also known as the Unix epoch 🌍. Voila! Your timestamp is ready for action! 💪
Option 2: Using the Date.now()
Method
If you're a fan of concise code, we have a treat for you! 🍬 JavaScript provides a handy-dandy shortcut with the Date.now()
method. It returns the same timestamp as our previous example, but with fewer lines of code:
const timestamp = Date.now();
console.log(timestamp);
Isn't it splendid? 😎
A Word of Caution: UTC vs. Local Time
Before you go off and timestamp all the things, we must address an important point ⚠️: timezones. The timestamp we've obtained using the methods above represents the number of milliseconds elapsed since the Unix epoch IN COORDINATED UNIVERSAL TIME (UTC) ⏰.
If you want to display the time in your local timezone, you'll need to handle some conversions. Thankfully, JavaScript has built-in methods like toLocaleString()
that can assist you in this endeavor. Don't forget to take this into account when using timestamps! 🌐
Now It's Your Turn to Code! 🚀👩💻👨💻
Congratulations, fellow coder! You've just learned how to obtain a timestamp in JavaScript. Now it's time to put your skills to the test and create something awesome! ⚡
Leave us a comment below and let us know how you plan to use timestamps in your projects. Are you building a real-time chat app? A sports event tracker? We'd love to hear about it! 😄
Keep coding, stay curious, and remember: timestamps are timeless! ⏳✨