How do I format a date in JavaScript?
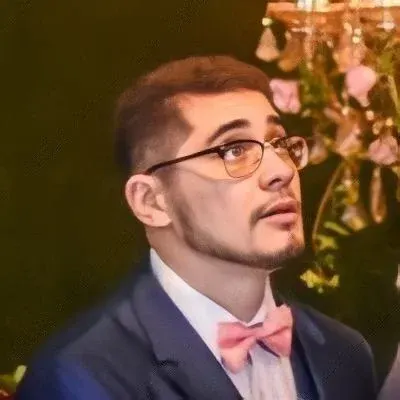
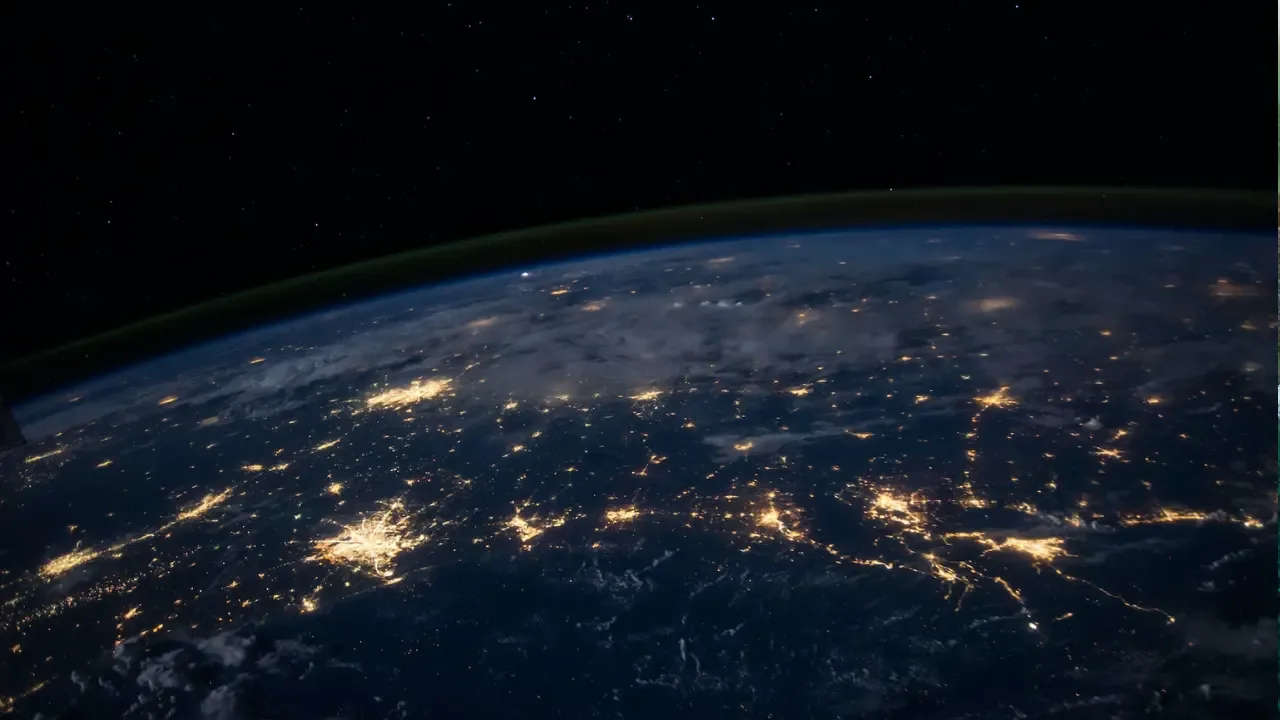
How to Format a Date in JavaScript 📅
So you want to format a JavaScript Date
object as a string? Preferably in the format: 10-Aug-2010
? No worries, we got you covered! In this guide, we'll walk you through the common issues, provide easy solutions, and empower you to impress your friends with your date formatting skills. Let's get started! 🚀
The Problem 🤔
Formatting dates in JavaScript can be a bit tricky, especially if you're new to the language. The built-in Date
object provides a variety of methods to manipulate and display dates, but formatting them to a specific format may not be straightforward.
The Solution 💡
To format a Date
object as a string, we can follow a few simple steps:
Create a new
Date
object:
const myDate = new Date();
Define the desired format:
const dateFormat = "dd-MMM-yyyy";
In this example, we're using the format "dd" for the day, "MMM" for the month abbreviation, and "yyyy" for the four-digit year. Feel free to customize the format according to your needs.
Implement a formatting function:
function formatDate(date, format) {
const day = String(date.getDate()).padStart(2, '0');
const month = date.toLocaleString('default', { month: 'short' });
const year = date.getFullYear();
return format
.replace('dd', day)
.replace('MMM', month)
.replace('yyyy', year);
}
const formattedDate = formatDate(myDate, dateFormat);
console.log(formattedDate); // Output: "10-Aug-2010"
The formatDate
function takes the Date
object and the desired format as arguments. It then extracts the day, month, and year components using the appropriate methods provided by the Date
object. Finally, it replaces the placeholders in the format string with the actual values.
And voilà! You've successfully formatted a date in JavaScript! 🎉
Going Beyond 😎
Now that you know the basics, you can unleash your creativity and format dates in any way you like. Here are a few examples to get you inspired:
Displaying the full month name:
To display the full month name instead of the abbreviation, simply change the MMM
string in the dateFormat
variable to MMMM
:
const dateFormat = "dd-MMMM-yyyy";
const formattedDate = formatDate(myDate, dateFormat);
console.log(formattedDate); // Output: "10-August-2010"
Including the day of the week:
If you want to include the day of the week in your date format, you can use the toLocaleDateString
method:
const dateFormat = "dddd, dd-MMM-yyyy";
const formattedDate = myDate.toLocaleDateString('default', { weekday: 'long' }) + ', ' + formatDate(myDate, dateFormat);
console.log(formattedDate); // Output: "Wednesday, 10-Aug-2010"
Great job! You're now a date formatting ninja! 🥷 But remember, JavaScript's Date
object offers many more methods and options for manipulating dates, so don't hesitate to explore the official documentation for further customization.
Your Turn! ✍️
Now it's time for you to put your new skills into action. Try formatting dates with different styles and share your results in the comments below. We'd love to see what you come up with!
If you found this guide helpful, don't forget to share it with your fellow developers. Happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
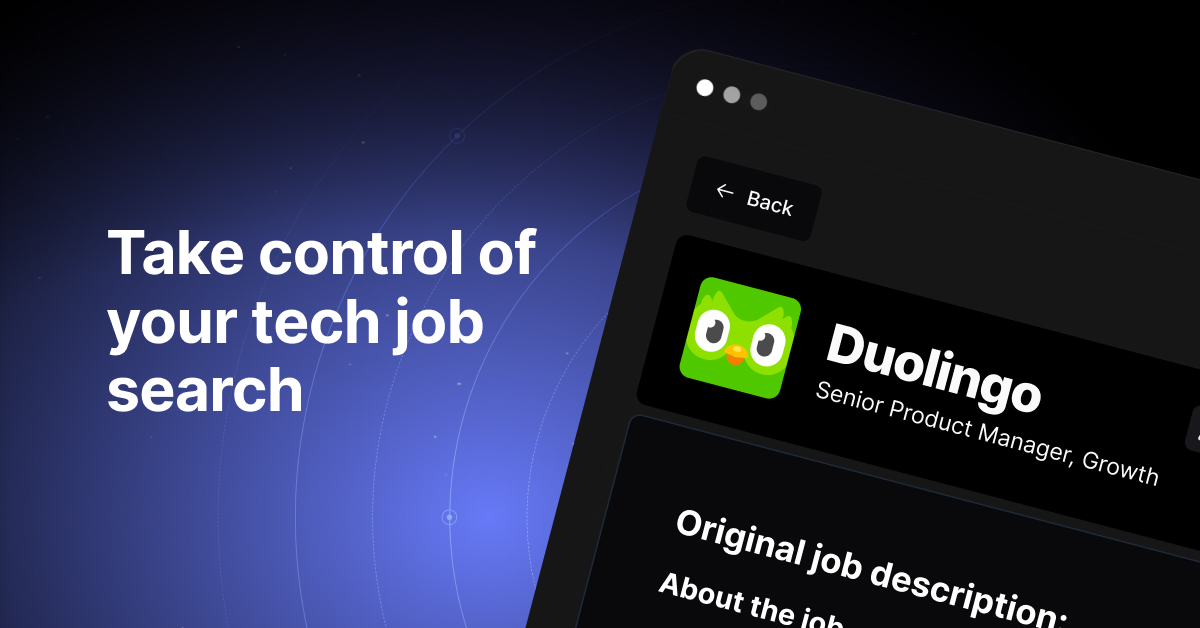