How do I find out which DOM element has the focus?
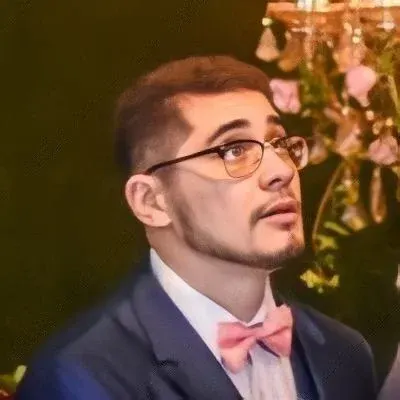
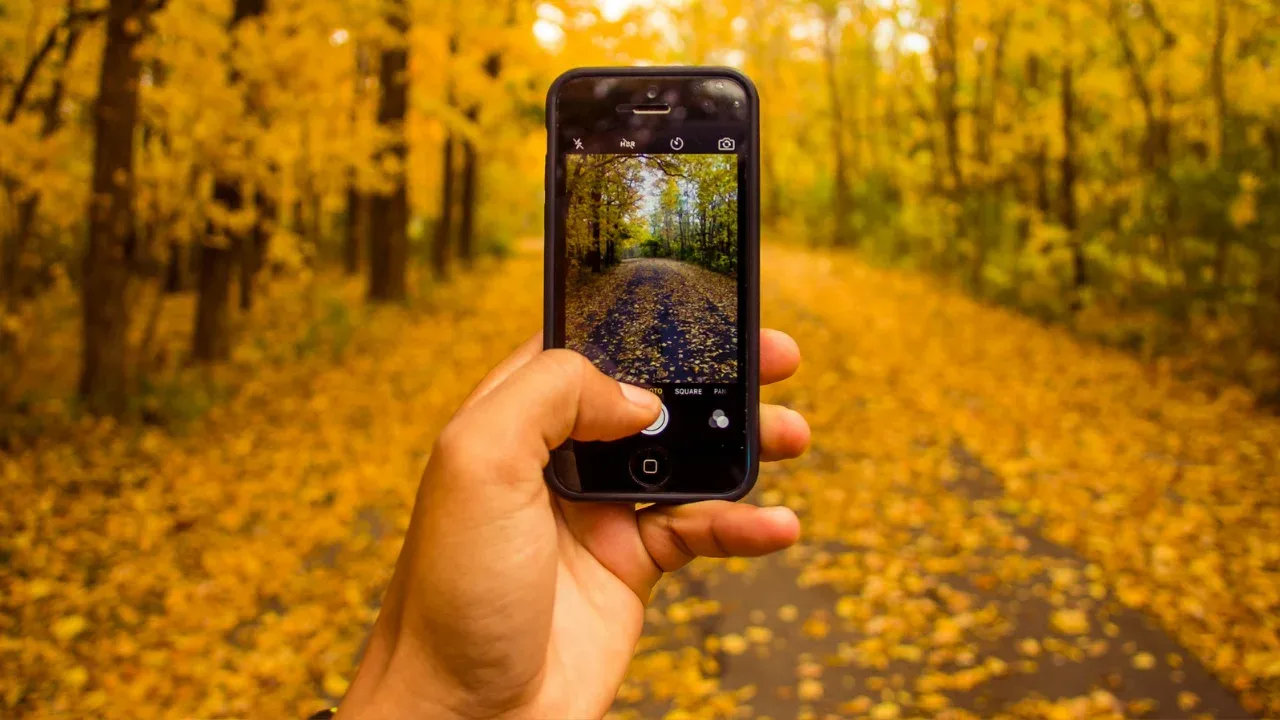
How to Find Out Which DOM Element Has the Focus
š Hey there tech enthusiasts! Are you having trouble determining which DOM element currently has the focus in your JavaScript code? š¤ No worries! I'm here to help you out with this common issue.
The Problem
The original question was posted by a curious developer who wanted to know how to identify the focused element in the DOM. š§ Their specific use case involved navigating through a table of input elements using arrow keys and the Enter key.
The Solution
To find out which element has the focus in JavaScript, we can leverage the document.activeElement
property. š This property returns the currently focused element within the DOM.
const focusedElement = document.activeElement;
By accessing this property, you'll obtain a reference to the element that currently holds the focus. Pretty cool, right? š
An Example
Let's look at an example that demonstrates how to use document.activeElement
to solve the specific use case mentioned earlier.
<table>
<tr>
<td><input type="text"></td>
<td><input type="text"></td>
</tr>
<tr>
<td><input type="text"></td>
<td><input type="text"></td>
</tr>
</table>
<script>
document.addEventListener('keydown', (event) => {
const focusedElement = document.activeElement;
if (event.key === 'ArrowUp') {
// Navigate to the input above the current one
} else if (event.key === 'ArrowDown') {
// Navigate to the input below the current one
} else if (event.key === 'Enter') {
// Perform some action when the Enter key is pressed
}
});
</script>
In this example, we're adding an event listener to the document that listens for keydown
events. Inside the event listener, we obtain the current focused element using document.activeElement
. Depending on the key pressed, we can perform the desired navigation or action logic.
Your Turn
Now that you know how to find out which DOM element has the focus, why not try implementing it in your own project? š Remember to consider the context and use case of your application to provide relevant functionality to the user.
If you have any questions or need further assistance, feel free to leave a comment below. Let's dive into this fascinating world of DOM manipulation together! šŖ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
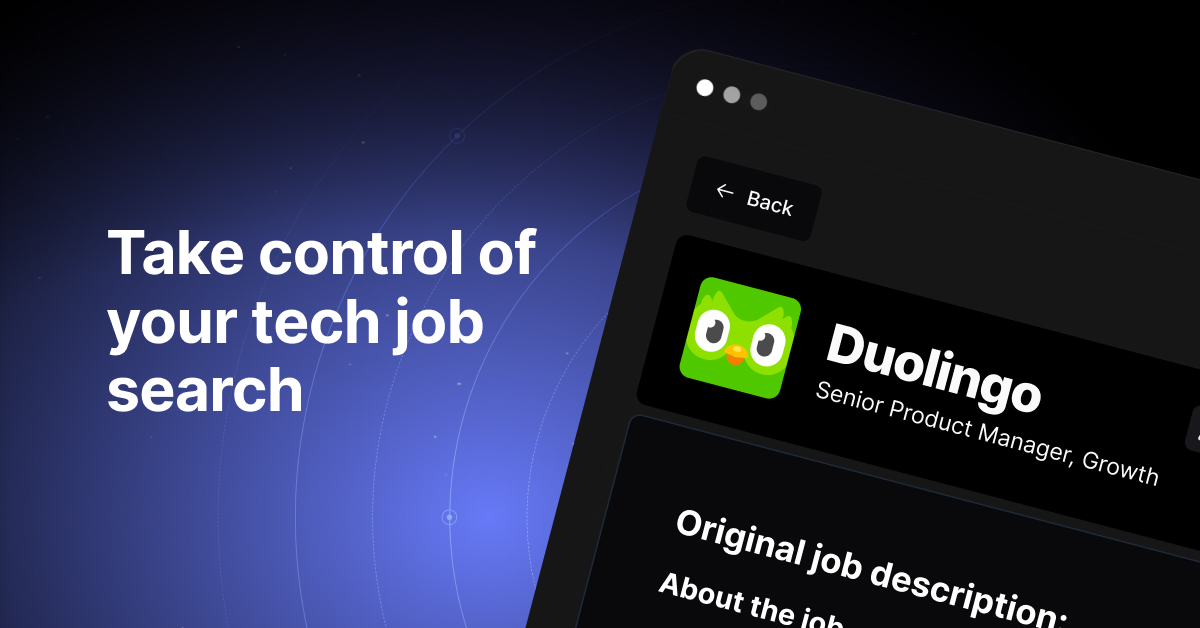