How do I empty an array in JavaScript?
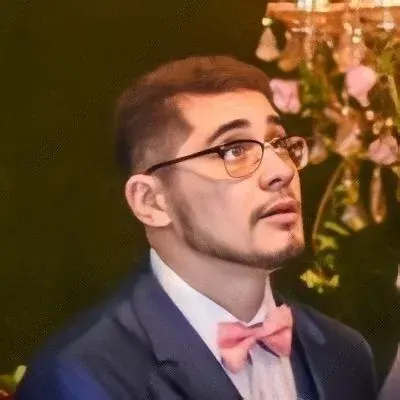
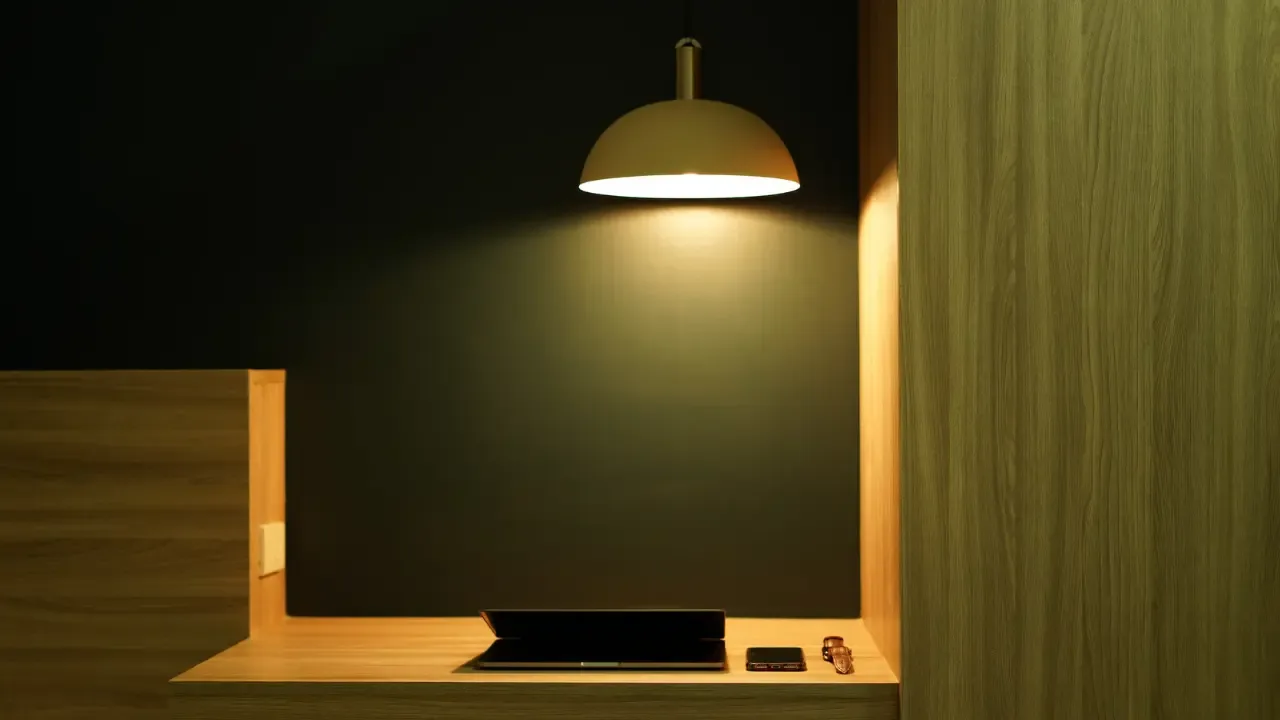
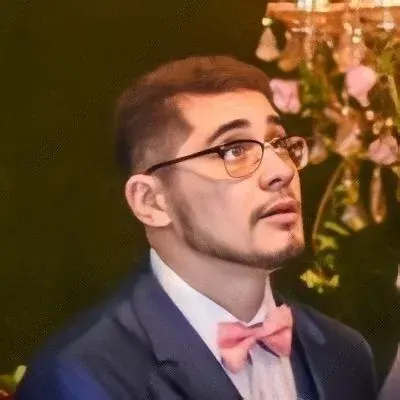
How to Empty an Array in JavaScript ποΈ
So you have an array in JavaScript and you want to clear out all its elements, but you're not quite sure how to do it. Fear not! π¦ΈββοΈ In this blog post, I'll explain various methods to help you empty an array in JavaScript without breaking a sweat! πͺ
The Problem π€
Let's consider the following array:
A = [1, 2, 3, 4];
Now, you want to completely empty this array, meaning you want to remove all its elements and start fresh.
The Solutions π‘
Method 1: Assigning an Empty Array
The simplest way to empty an array is by assigning it an empty array.
A = []; // This assigns an empty array to A
Using this method, the previous array will be completely cleared, and you will have an empty array ready for use.
Method 2: Using the splice() Method
Another way to empty an array is by using the splice()
method. This method allows you to remove elements from an array, starting from a specified index.
A.splice(0, A.length);
The splice()
method takes two arguments: the starting index and the number of items to remove. By setting the starting index to 0 and the number of items to A.length
, we can effectively remove all elements from the array A
.
Choosing the Right Method π€·ββοΈ
Both methods will empty your array, so which one should you choose? It depends on your specific use case.
If you want to preserve the reference of the array (i.e., if other variables are referencing the same array), using the
splice()
method might be more appropriate.On the other hand, if you want to completely replace the array with a new empty one, assigning an empty array is simpler and cleaner.
Consider your needs and choose the method that suits your situation best.
Conclusion π
Emptying an array in JavaScript is a common task, but it doesn't have to be complicated. By following the methods I've outlined in this blog post, you can confidently clear out arrays without any hassle.
So go ahead, give these methods a try, and keep coding! π»
Have any other JavaScript questions or tips for emptying an array? Let us know in the comments below! Let's keep the conversation going! π¬