How do I debug Node.js applications?
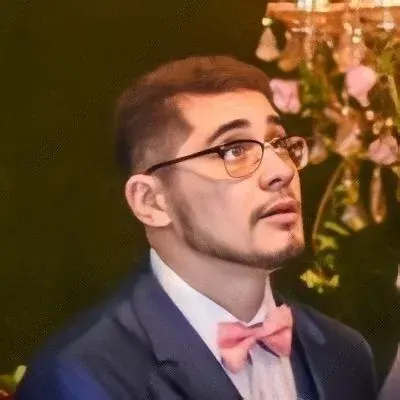
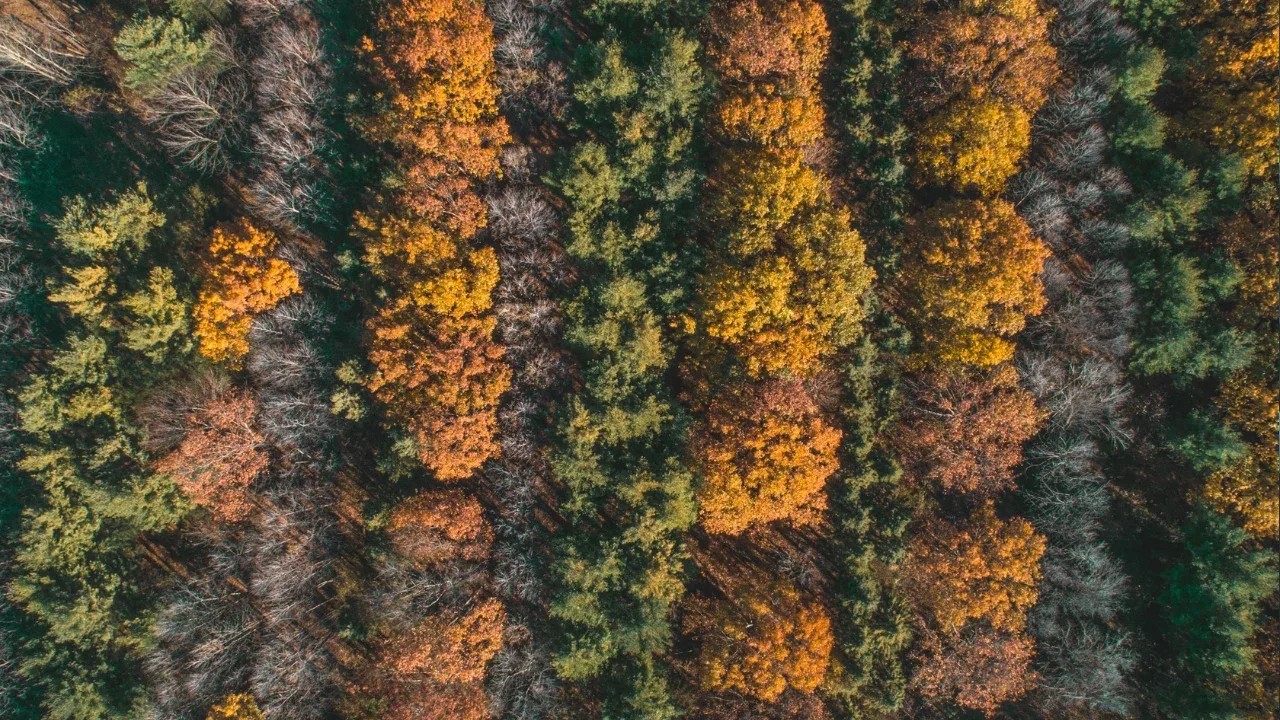
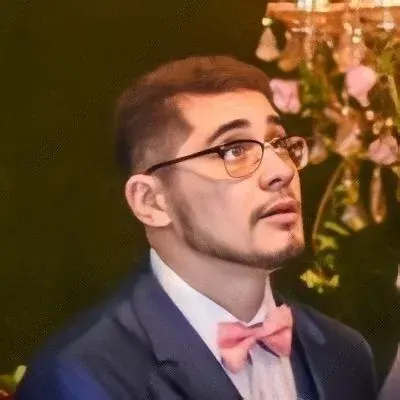
Debugging Node.js Applications: A Beginner's Guide 👨💻
Are you tired of using primitive alert debugging techniques to chase down bugs in your Node.js applications? Well, fret no more! 🥳 In this guide, we will explore the world of Node.js debugging and uncover the power of the developer's best friend - the debugger. 💪
The Struggles of Alert Debugging 😩
Using sys.puts
with sys.inspect
might have sufficed when you were starting out, but as your Node.js applications grow in complexity, so do the bugs. Printing out variables and object contents to the console becomes tedious, time-consuming, and downright frustrating. 😫
Enter the Chrome Debugger 🕵️♀️
Did you know that the Google Chrome debugger can help you debug your Node.js applications as well? That's right! The powerful tools you love for debugging web applications can also be used for troubleshooting Node.js servers. 🌐
Setting Up Chrome Debugger for Node.js 🚀
To get started with Chrome Debugger in Node.js, follow these steps:
Install the latest version of Node.js on your machine.
Update your Node.js application to include the
--inspect
flag when running it:
node --inspect server.js
Open your Chrome browser and type
chrome://inspect
in the address bar.Click on the "Open dedicated DevTools for Node" link.
In the DevTools window that opens, navigate to the "Sources" tab.
On the left-hand side, you should see a list of running Node.js processes. Click on your application to start debugging.
Debugging Like a Pro 🎯
Now that you have the Chrome Debugger set up, let's explore some of its powerful features:
Breakpoints ⛔️
Set breakpoints in your code by clicking on the line number in the source file. When your application hits a breakpoint, it pauses execution and allows you to inspect variables, call stack, and even execute code in the console.
Step-by-Step Execution ⏩
Step through your code line by line using the step buttons (Step over, Step into, and Step out). This helps you understand the flow of your application and pinpoint the exact moment where a bug occurs.
Watch Expressions 👁️🗨️
Enter expressions into the watch panel to keep an eye on specific variables or objects. As you step through your code, the watch panel will update in real-time, showing you the current values of those expressions.
Console Logging 📝
Instead of cluttering your code with sys.puts
statements, you can use the console in Chrome Debugger to log messages and inspect variables on the fly. It's much cleaner and more efficient!
📣 Get Involved: Share Your Experience! 📣
Now that you know the magic of the Chrome Debugger for Node.js, why not share your experiences with the developer community? Head over to our website and leave a comment. We'd love to hear your success stories, tips, and tricks for debugging Node.js applications. Let's debug together! 🤝
Happy debugging! 🐛✨