How do I create a GUID / UUID?
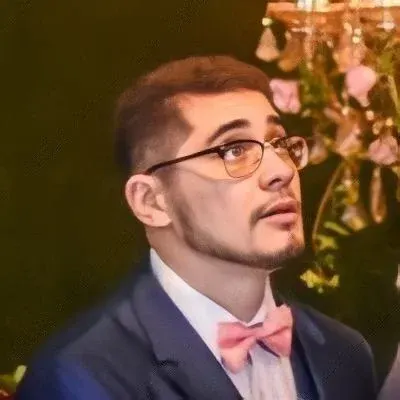
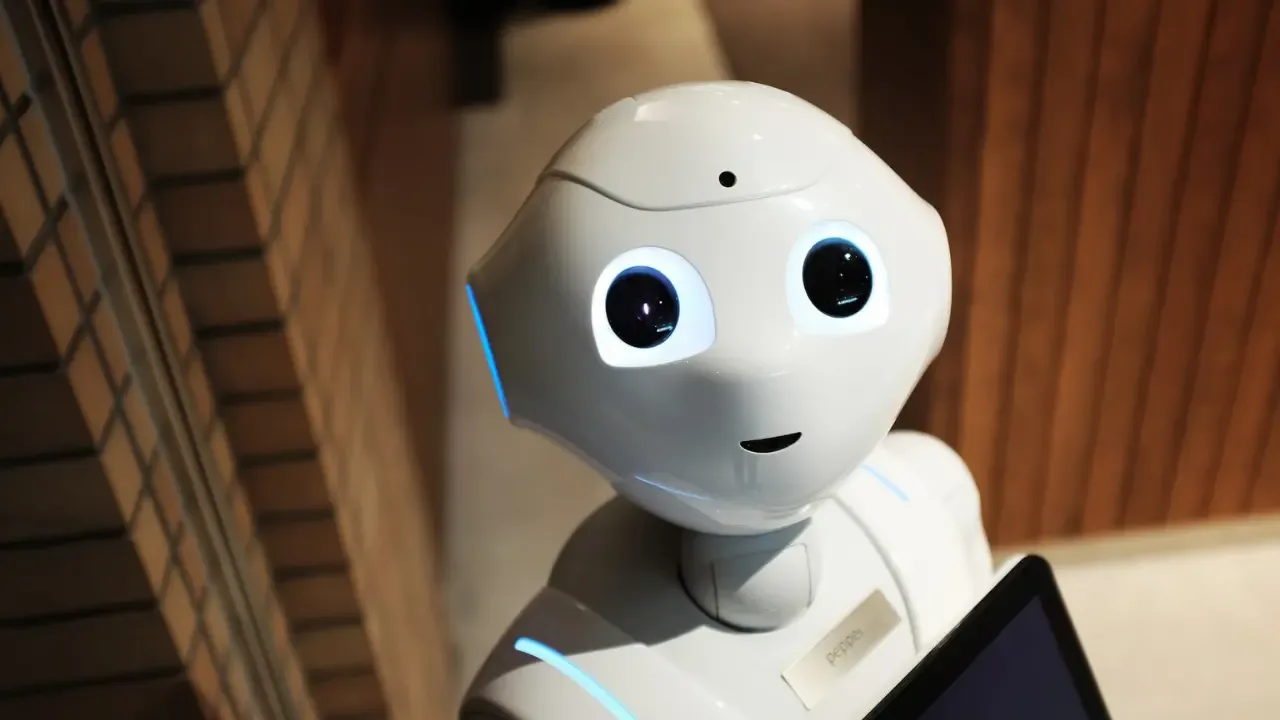
🆔 How to Create a GUID / UUID?
Have you ever wondered how to generate those unique identifiers that are used to identify objects across different systems? Well, look no further! In this blog post, we will dive into the world of GUIDs (globally unique identifiers) and explore how to create them using JavaScript. 🎉
Why Do We Need GUIDs / UUIDs?
GUIDs are essential in various fields, such as database management and software development, where unique identification of objects is crucial. They allow us to differentiate entities accurately and avoid conflicts when working with distributed systems or merging databases.
The Challenge: JavaScript and GUID Generation
Creating GUIDs in JavaScript might seem intimidating at first, as different browsers offer varying support for random number generators. Additionally, ensuring that the generated GUIDs stay within the ASCII character range is essential to prevent any complications during transmission.
The Solution: A Simple Approach
Fear not! We have a simple and effective solution for generating GUIDs in JavaScript. Let's break it down:
function generateGUID() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function (c) {
var r = Math.random() * 16 | 0,
v = c === 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
});
}
Here's how this code works:
We define a function called
generateGUID
that returns a GUID.The
replace
method is used to create a template for our GUID, consisting of both static and dynamic parts. The static parts are highlighted in single quotes, while the dynamic parts are represented byx
andy
.The
replace
method takes a regular expression and a callback function as arguments.Inside the callback function, we generate random hexadecimal digits for each occurrence of
x
andy
usingMath.random()
andtoString(16)
. The digit forx
can be any random number, while the digit fory
is slightly adjusted to match the required format.Finally, the generated digits replace the corresponding placeholders
x
andy
in the template, resulting in a valid GUID.
And that's it! 🚀 With a few lines of code, you can now create your own GUIDs in JavaScript!
The Call-to-Action: Share Your Experience!
Now that you have mastered the art of GUID generation, it's time to put your skills to the test. Share your experience with us and let us know how GUIDs have improved your development process. We always enjoy hearing from our readers, so leave a comment below! 😄
Remember, GUIDs play a vital role in various scenarios, so feel free to bookmark this post for future reference. Happy coding! 👩💻👨💻
Don't forget to follow us on Twitter and Facebook for more exciting tech tips and tricks!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
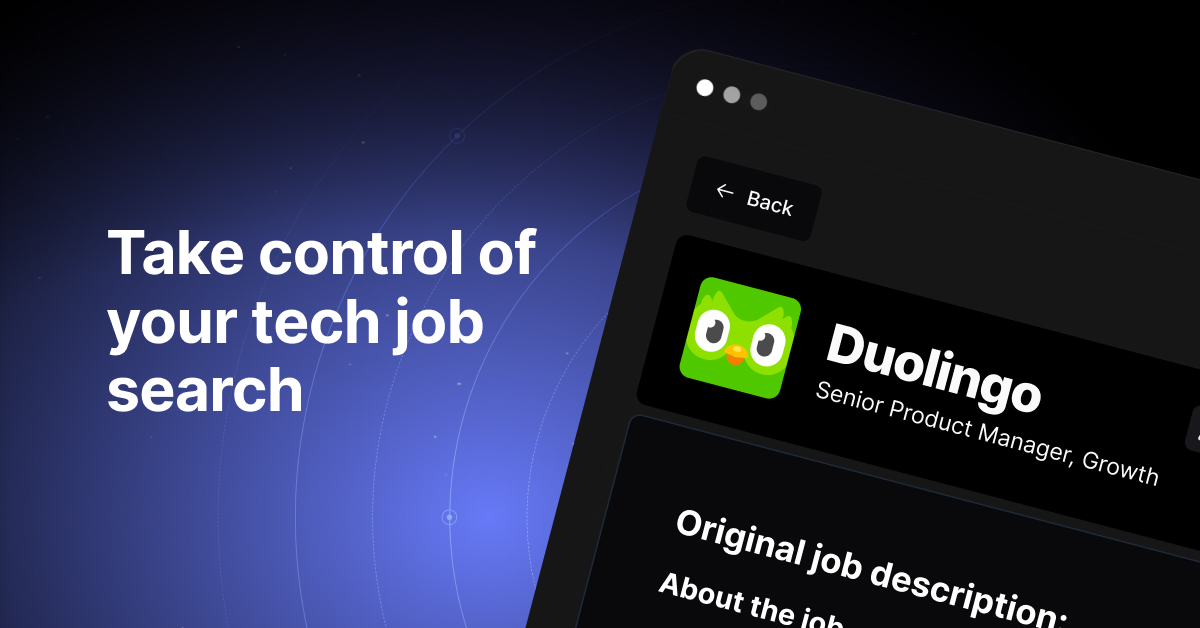