How do I convert an existing callback API to promises?
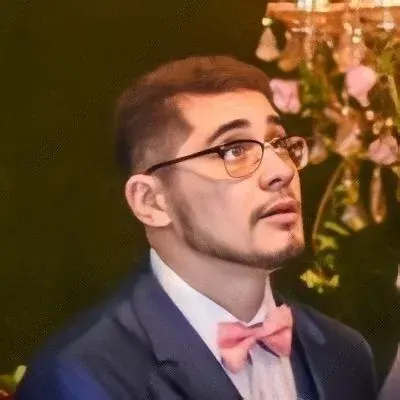
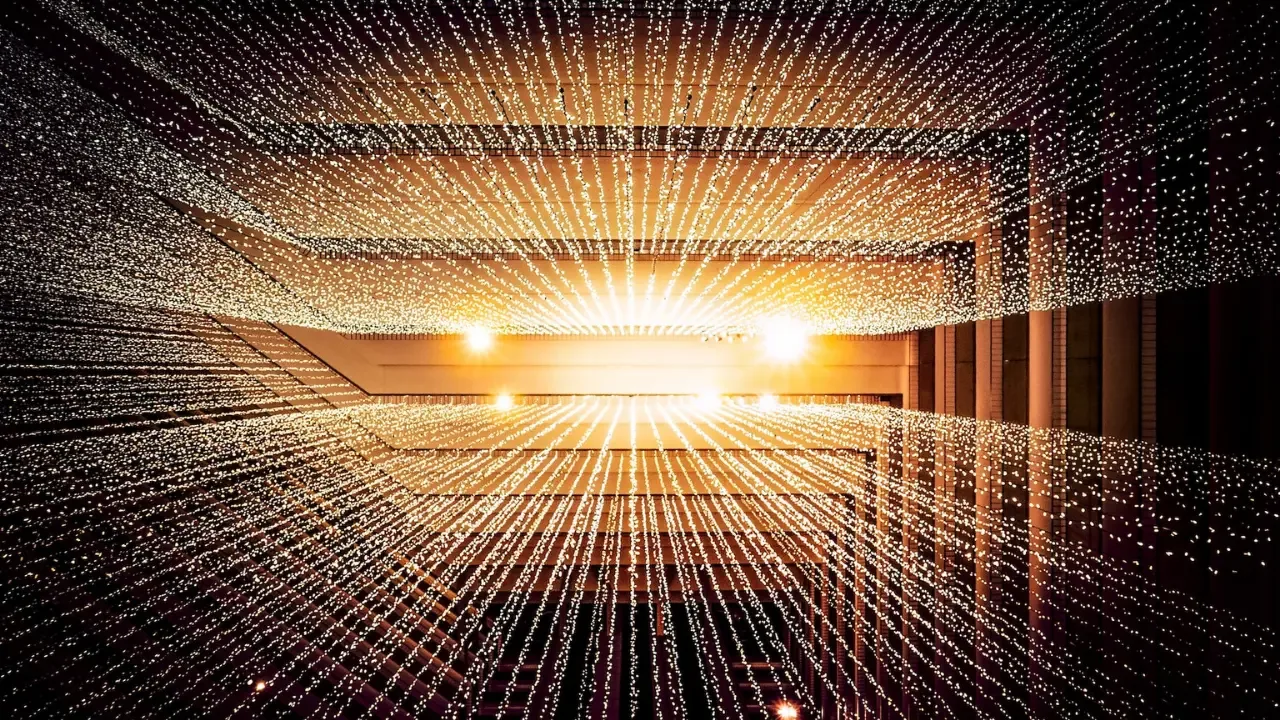
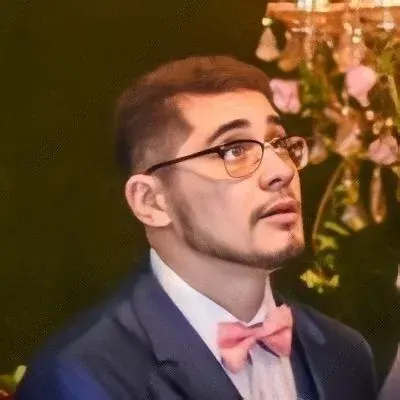
Converting Callback API to Promises: A Comprehensive Guide 🔄✨
Are you tired of working with callback APIs and longing for the simplicity and elegance of promises? Fear not! In this guide, we will explore how to convert an existing callback API to promises, making your code more readable, maintainable, and downright enjoyable. 😊
The Challenge: Callback APIs in Various Formats
Callback APIs come in different flavors, ranging from simple one-time events to complex libraries with nested callbacks. Let's take a look at some common scenarios:
1️⃣ DOM Load or One-Time Event
In this scenario, we often set a callback to be executed when a certain event occurs, such as the window.onload
event. Here's an example:
window.onload = function() {
// Handle the event
};
2️⃣ Plain Callback
A plain callback is a function that gets invoked when a specific action or change happens. It can be used in various contexts. Here's an example:
function request(onChangeHandler) {
// Perform some action
}
request(function() {
// Handle the change
});
3️⃣ Node-style Callback ("Nodeback")
Node.js introduced a convention for callbacks where the first argument is an error object (if any), followed by the data. This convention is often referred to as "nodeback." Here's an example:
function getStuff(data, callback) {
// Do some asynchronous work
}
getStuff("dataParam", function(err, data) {
// Handle the result
});
4️⃣ Libraries with Nested Callbacks
Some libraries have a chain of callbacks that need to be executed in a specific order. Here's an example:
API.one(function(err, data) {
API.two(function(err, data2) {
API.three(function(err, data3) {
// Handle the final result
});
});
});
The Solution: Promisify to the Rescue! 🚀✨
To convert a callback API to promises, we can use a technique called "promisify." The idea is to wrap the callback-based function in a promise so that we can work with promises seamlessly. Let's explore how to do this for each of our scenarios:
1️⃣ DOM Load or One-Time Event
In this case, we can create a promise that resolves when the event occurs. Here's how you can do it:
const loadPromise = new Promise((resolve, reject) => {
window.onload = function() {
resolve();
};
});
// Usage
loadPromise.then(() => {
// Handle the event
});
2️⃣ Plain Callback
To convert a plain callback function to a promise, we can use the util.promisify
method available in Node.js:
const util = require('util');
const requestPromise = util.promisify(request);
// Usage
requestPromise().then(() => {
// Handle the change
});
3️⃣ Node-style Callback ("Nodeback")
For a function with a nodeback-style callback, we can manually wrap it in a promise. Here's how:
function getStuffAsync(data) {
return new Promise((resolve, reject) => {
getStuff(data, function(err, result) {
if (err) {
reject(err);
} else {
resolve(result);
}
});
});
}
// Usage
getStuffAsync("dataParam").then((result) => {
// Handle the result
}).catch((err) => {
// Handle the error
});
4️⃣ Libraries with Nested Callbacks
To handle libraries with nested callbacks, we can use the power of promises and create a sequence of promises using Promise.all
or async/await
. Here's an example:
const promisifiedAPI = {
one: util.promisify(API.one),
two: util.promisify(API.two),
three: util.promisify(API.three)
};
// Usage with async/await
async function handleAPICalls() {
try {
const data1 = await promisifiedAPI.one();
const data2 = await promisifiedAPI.two();
const data3 = await promisifiedAPI.three();
// Handle the final result
} catch (err) {
// Handle any errors
}
}
handleAPICalls();
Embrace the Power of Promises! 💪🌟
By converting your existing callback APIs to promises, you can unlock the full potential of asynchronous programming. Promises allow for cleaner, more readable code that is easier to maintain and debug. So why wait? Start converting your callback APIs to promises today and level up your coding game! ✨🚀
Are you ready to take the plunge into the world of promises? Share your thoughts, experiences, and any challenges you faced in the comments below. Let's engage in a conversation and help each other harness the power of promises! 👇💬