How do I convert a float number to a whole number in JavaScript?
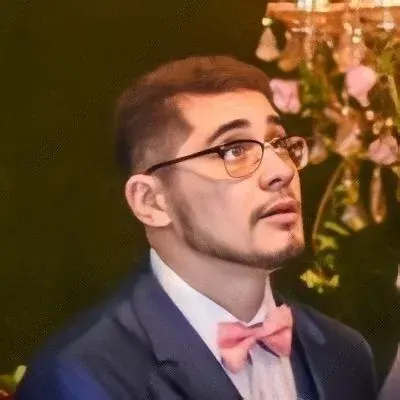
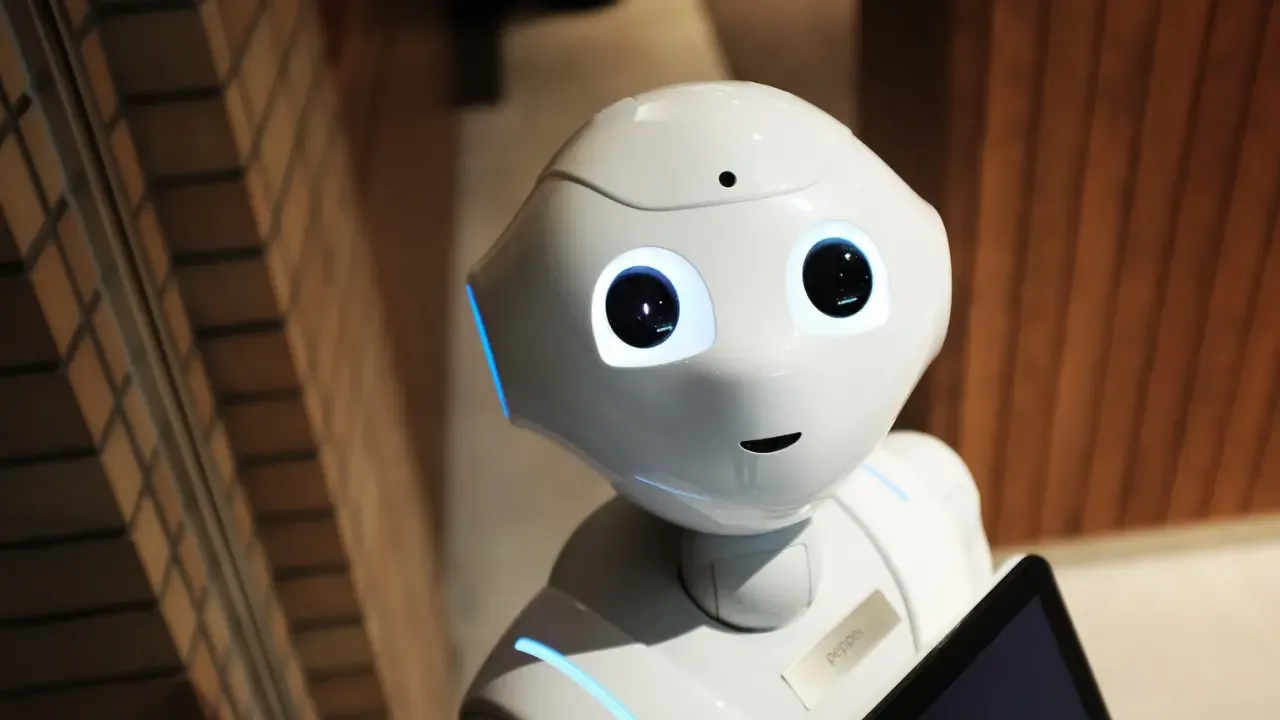
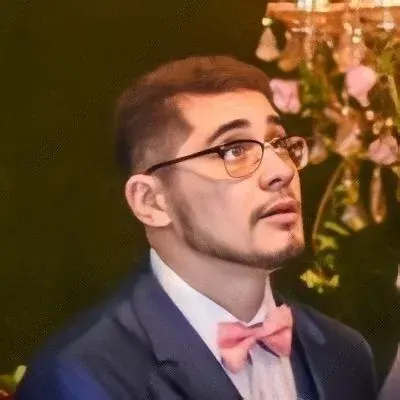
How to Convert a Float Number to a Whole Number in JavaScript 🤔💭
Are you trying to convert a float (decimal) number to a whole number (integer) in JavaScript? Look no further! In this guide, we'll walk you through two standard methods: truncating and rounding. And the best part? We'll show you efficient ways to achieve this without resorting to converting to a string and parsing. Let's dive in! 💻🚀
The Challenge 🌊
You may be wondering: why can't we simply convert a float to an integer in JavaScript? Well, it's because JavaScript treats numbers with decimal places as floating-point numbers by default. However, there are cases where we need to work with whole numbers only, such as when dealing with integers in mathematical operations or when working with certain APIs that require whole numbers.
Solution 1: Truncating ✔️
Truncating a float number essentially means removing its decimal part without rounding up or down. This method is often used when exact precision is required. Here's how you can achieve this in JavaScript:
let floatNumber = 3.75;
let wholeNumber = Math.trunc(floatNumber);
console.log(wholeNumber); // Output: 3
By using the Math.trunc()
function, we discard everything after the decimal point and return the integer part of the number.
Solution 2: Rounding 🔄
If you need to round the float number to the nearest whole number, JavaScript provides the Math.round()
function. It rounds up or down based on the decimal part. Here's an example:
let floatNumber = 3.75;
let wholeNumber = Math.round(floatNumber);
console.log(wholeNumber); // Output: 4
In this case, the decimal part of 3.75
is greater than or equal to 0.5
, so the number is rounded up to the nearest whole number, which is 4
.
Efficiency Matters! 💪
Converting a float number to a whole number without converting to a string can improve performance and efficiency. The methods we've discussed, Math.trunc()
and Math.round()
, are both powerful and efficient. By using these built-in functions, you can save time and computational resources.
Moreover, when using these methods, it's important to keep in mind how JavaScript handles numbers internally. Due to the limitations of floating-point arithmetic, some calculations may result in unexpected outcomes. Always test your code thoroughly to ensure accurate conversions.
Conclusion and Your Turn! 🎉
Converting float numbers to whole numbers is a common task in JavaScript, and now you have two efficient methods in your toolkit: truncating and rounding. Remember, Math.trunc()
is great when you want to discard the decimal part, while Math.round()
rounds the number up or down based on the decimal part.
Now it's your turn to try it out! ✨ What other JavaScript topics would you like us to cover in future blog posts? Let us know in the comments below, and don't forget to share this post with your fellow developers! 📢💌
Happy coding! 💻😄