How do I conditionally add attributes to React components?
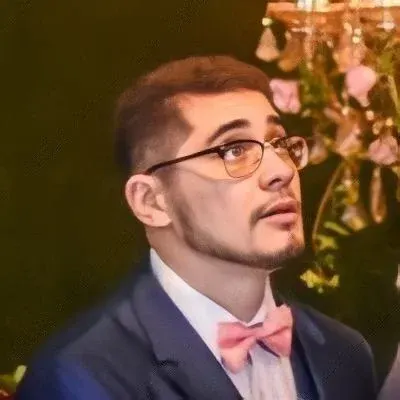
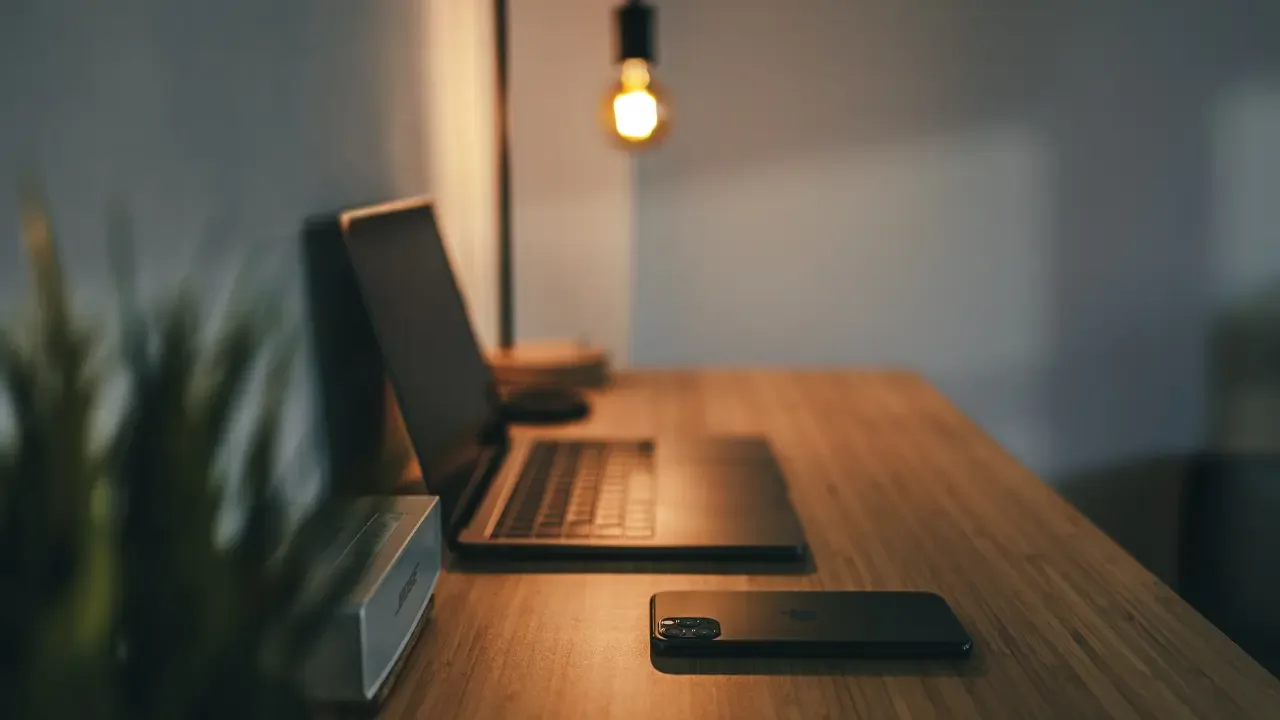
How to Conditionally Add Attributes to React Components! 😎🚀
Are you struggling to add attributes to your React components based on certain conditions? 😫 Don't worry, we've got your back! In this blog post, we'll address this common issue and provide easy solutions to help you ace your React game! 💪🔥
The Problem 🤔
A fellow developer posed this question on our forums:
"Is there a way to only add attributes to a React component if a certain condition is met? I need to add
required
andreadOnly
attributes to form elements based on an Ajax call after render. But somehow,readOnly="false"
doesn't work, and omitting the attribute completely is not the same. Help!"
Our developer friend shared the following code snippet, which showcases the desired behavior, but unfortunately doesn't work as expected:
function MyInput({isRequired}) {
return <input className="foo" {isRequired ? "required" : ""} />
}
Let's dive in and uncover the easy solutions to this problem! 💡💻
Solution 1: Using String Concatenation 🧩
One way to conditionally add attributes to your React components is by using string concatenation. Here's how you can modify the MyInput
component using this approach:
function MyInput({isRequired}) {
const requiredAttribute = isRequired ? "required" : "";
return <input className="foo" {...(requiredAttribute && {required: true})} />;
}
In this solution, we create a requiredAttribute
variable that stores either the value "required"
or an empty string, based on the condition isRequired
. Using the JavaScript spread operator ({...}
), we can dynamically add the required
attribute to the input element if requiredAttribute
is truthy.
Solution 2: Using Ternary Operator and Spread Operator 🎯
Another approach is to combine the ternary operator and the spread operator to conditionally add attributes. Let's update the MyInput
component to leverage this technique:
function MyInput({isRequired}) {
return (
<input
className="foo"
{...(isRequired ? {required: true} : {})}
/>
);
}
In this solution, we use the ternary operator ?:
to determine if isRequired
is true
. If it is, we use the spread operator to add the required
attribute with a value of true
. If not, we pass an empty object {}
so that no additional attributes are added.
Solution 3: Using JavaScript Conditional Statements 🌈
If you prefer a more explicit approach, you can use JavaScript conditional statements to dynamically render different components with the desired attributes. Let's take a look:
function MyInput({isRequired}) {
if (isRequired) {
return <input className="foo" required />;
} else {
return <input className="foo" />;
}
}
By leveraging the if
statement, we can conditionally render different components based on the value of isRequired
. In this case, if isRequired
is true
, we render the input element with the required
attribute; otherwise, we render it without the required
attribute.
Conclusion and Call-to-Action 🏁📣
Adding attributes conditionally to React components doesn't have to be a headache! You can easily tackle this problem using string concatenation, the ternary operator, spread operators, or JavaScript conditional statements. Choose the solution that suits your coding style and project requirements.
Now it's your turn! Have you encountered other challenges in React that you'd like us to solve in a future blog post? Let us know in the comments section below! 👇
Remember, sharing is caring! If you found this blog post helpful, sharing it with your fellow developers can help them overcome similar challenges. Happy coding! 🙌💻
This blog post was brought to you by the React Nerds team. Stay tuned for more React-ivating content!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
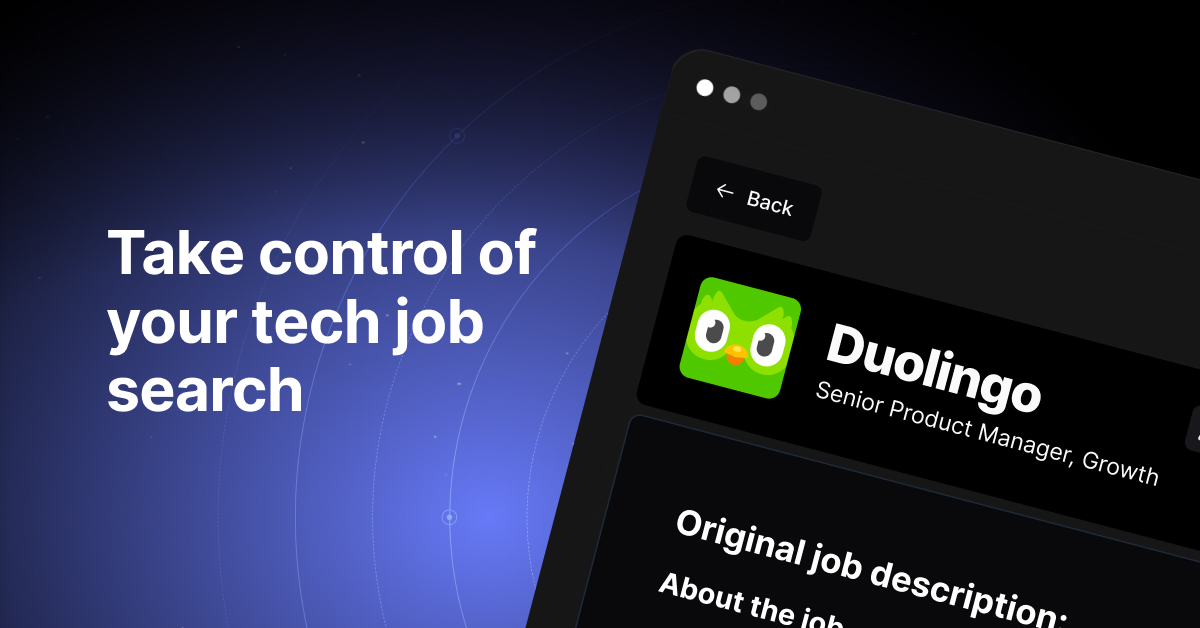