How do I check whether an array contains a string in TypeScript?
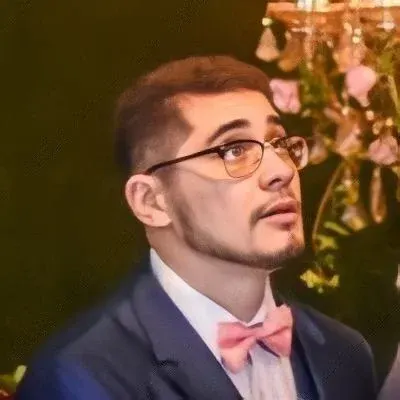
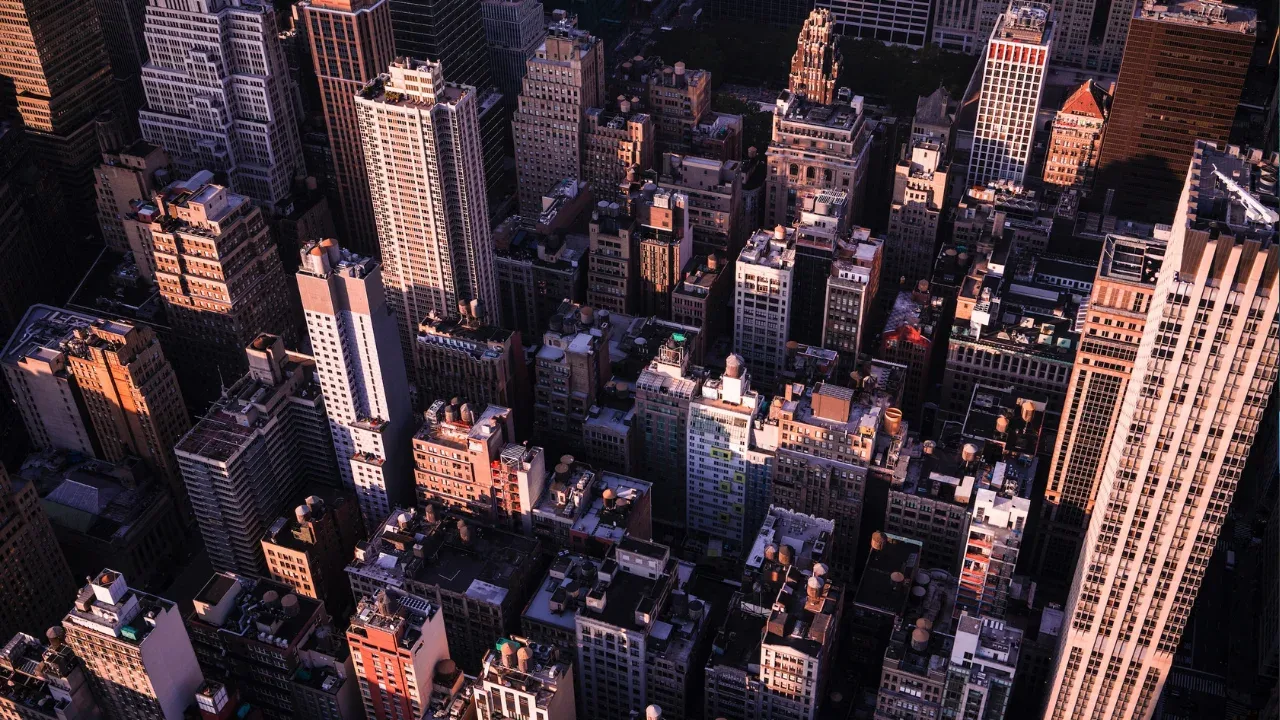
How to Check Whether an Array Contains a String in TypeScript? 😎📚
Hello, fellow TypeScript enthusiasts! Today, we are going to tackle a common issue in TypeScript: how to check if an array contains a specific string. This is a question that often pops up when working with arrays in TypeScript, particularly in scenarios involving Angular applications.
Let's dive right in and explore some easy solutions to this problem. 💪
Solution 1: Using the Array.includes() Method 🧰
One straightforward way to check if an array contains a string in TypeScript is by utilizing the includes()
method provided by the Array object. This method returns a boolean value indicating whether the specified string is found in the array.
In the given example, we have an array called channelArray
:
var channelArray: Array<string> = ['one', 'two', 'three'];
To check if this array contains the string 'three', we can use the includes()
method like this:
if (channelArray.includes('three')) {
console.log("The array contains the string 'three'!");
} else {
console.log("The array does not contain the string 'three'.");
}
🔍 Explanation:
The includes()
method performs a simple linear search in the array, returning true
if it finds a match and false
otherwise. It is a clean and concise way to solve our problem.
Solution 2: Using the Array.indexOf() Method 📌
An alternative approach to check if an array contains a string is by employing the indexOf()
method. This method searches the array for the specified string and returns its index if found, or -1 if not found.
Here's how you can use indexOf()
to check if the 'three' string is present in the channelArray
:
if (channelArray.indexOf('three') !== -1) {
console.log("The array contains the string 'three'!");
} else {
console.log("The array does not contain the string 'three'.");
}
🔍 Explanation:
By checking if the return value of indexOf()
is not equal to -1, we can determine whether the string is present in the array. If the return value is -1, it means the string was not found.
Solution 3: Using the Array.some() Method 👥
For more complex scenarios, where you need to perform more advanced checks on the array elements, the some()
method can come to the rescue. This method tests whether at least one element in the array passes the provided test function.
Consider the following example, where we want to check if the array contains any string with the letter 'o' in it:
if (channelArray.some((str) => str.includes('o'))) {
console.log("There's a string in the array containing the letter 'o'!");
} else {
console.log("No string in the array contains the letter 'o'.");
}
🔍 Explanation:
The some()
method iterates over each element in the array and executes the given test function. In this case, we're using an arrow function to check if each element (str
) contains the letter 'o'. If at least one element passes the test, the method returns true
.
Conclusion and Call-to-Action 🏁📢
Congratulations! Now you know a handful of ways to check whether an array contains a specific string in TypeScript. With the help of the includes()
, indexOf()
, and some()
methods, you can tackle this common problem effortlessly.
Next time you encounter a similar situation in your TypeScript application, feel free to refer back to this guide for quick and easy solutions!
If you found this blog post helpful, please share it with your fellow TypeScript developers. And if you have any questions or other TypeScript topics you'd like us to cover, leave a comment below. Let's keep the conversation going! 👇🤝
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
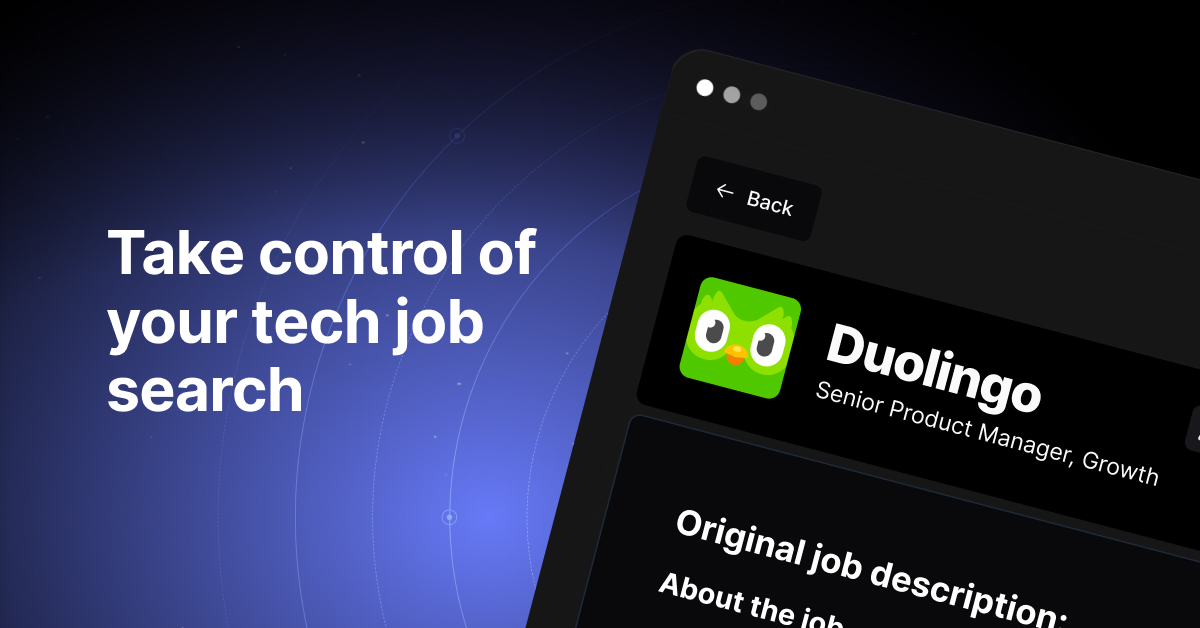