How do I check whether a checkbox is checked in jQuery?
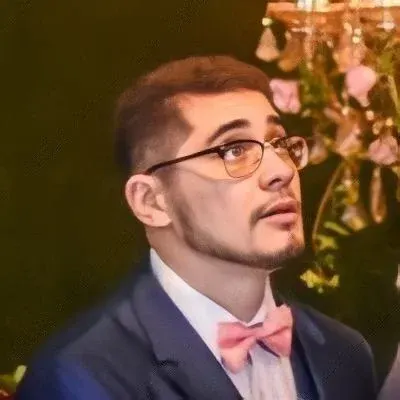

📝 Easy Guide: How to Check Whether a Checkbox is Checked in jQuery 📝
Are you struggling to check the checked
property of a checkbox using jQuery? Don't worry, we've got your back! 🤝 In this article, we'll address common issues related to this problem and provide you with easy-to-follow solutions. Let's dive in! 💪
The Problem:
You need to perform an action based on the checked
property of a checkbox using jQuery. For instance, you want to show a textbox to enter the age if the checkbox is checked, otherwise, hide it.
The code provided in the context section seems promising but returns false
by default. Let's see how we can fix this. 🔍
Solution 1: Using the prop()
Method:
Instead of using the attr()
method, you can use the prop()
method to check the checked
property. This method is more reliable when it comes to working with boolean attributes such as checked
. 🎯
if ($('#isAgeSelected').prop('checked')) {
$("#txtAge").show();
} else {
$("#txtAge").hide();
}
With this approach, you can accurately check whether the checkbox is checked and perform your desired action accordingly. ✔️
Solution 2: Utilizing the :checked
Selector:
jQuery provides a handy selector called :checked
, which allows you to select all checked checkboxes in your document. By combining this selector with jQuery's functions, you can easily manipulate the elements. 🕺
if ($('#isAgeSelected:checked').length > 0) {
$("#txtAge").show();
} else {
$("#txtAge").hide();
}
This code snippet checks if the checkbox with the ID isAgeSelected
is checked. If it is, the txtAge
element is shown; otherwise, it is hidden. 🎉
Your Turn to Shine! ✨
Now that you know how to check whether a checkbox is checked in jQuery, it's time to put your skills to the test! 🚀 Try integrating this code into your project and see the magic happen. If you face any issues or have additional questions, feel free to leave a comment below. We're here to help! 💪
Was this article helpful in solving your checkbox dilemma? If so, don't forget to share it with your fellow developers and spread the knowledge! Let's make coding easier for everyone! 🌟
Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
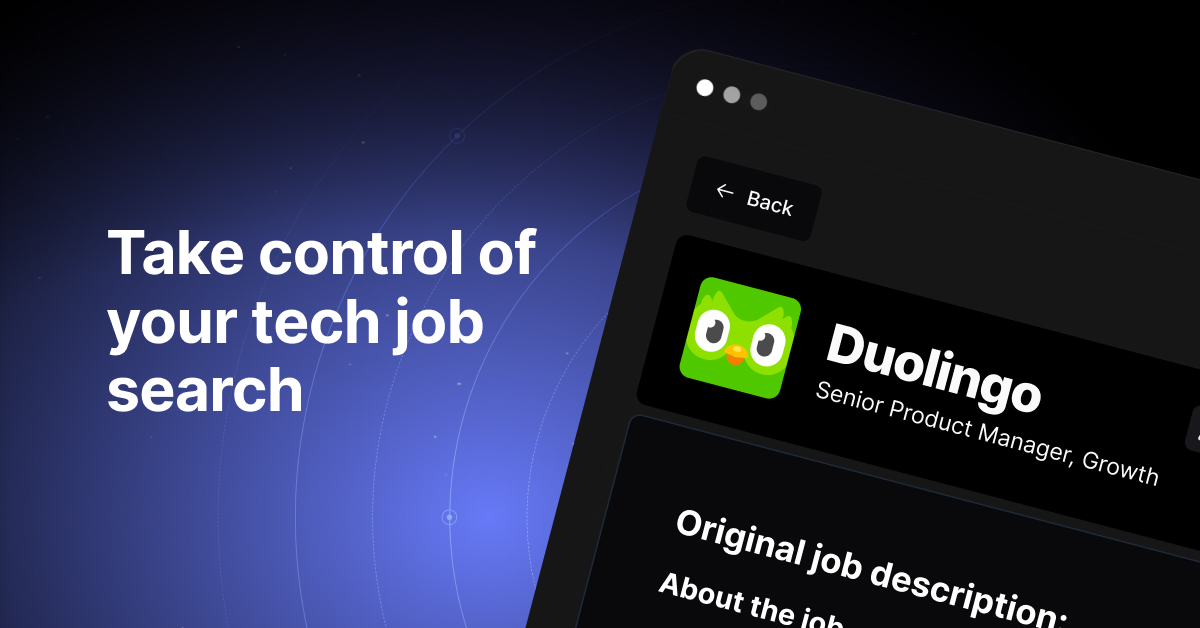