How do I check in JavaScript if a value exists at a certain array index?
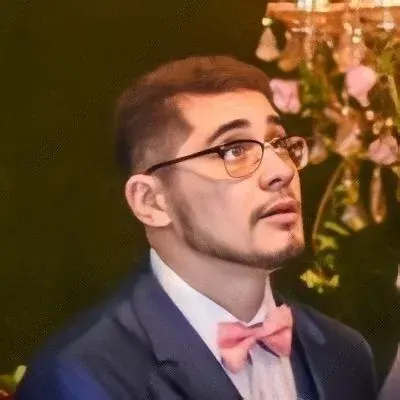
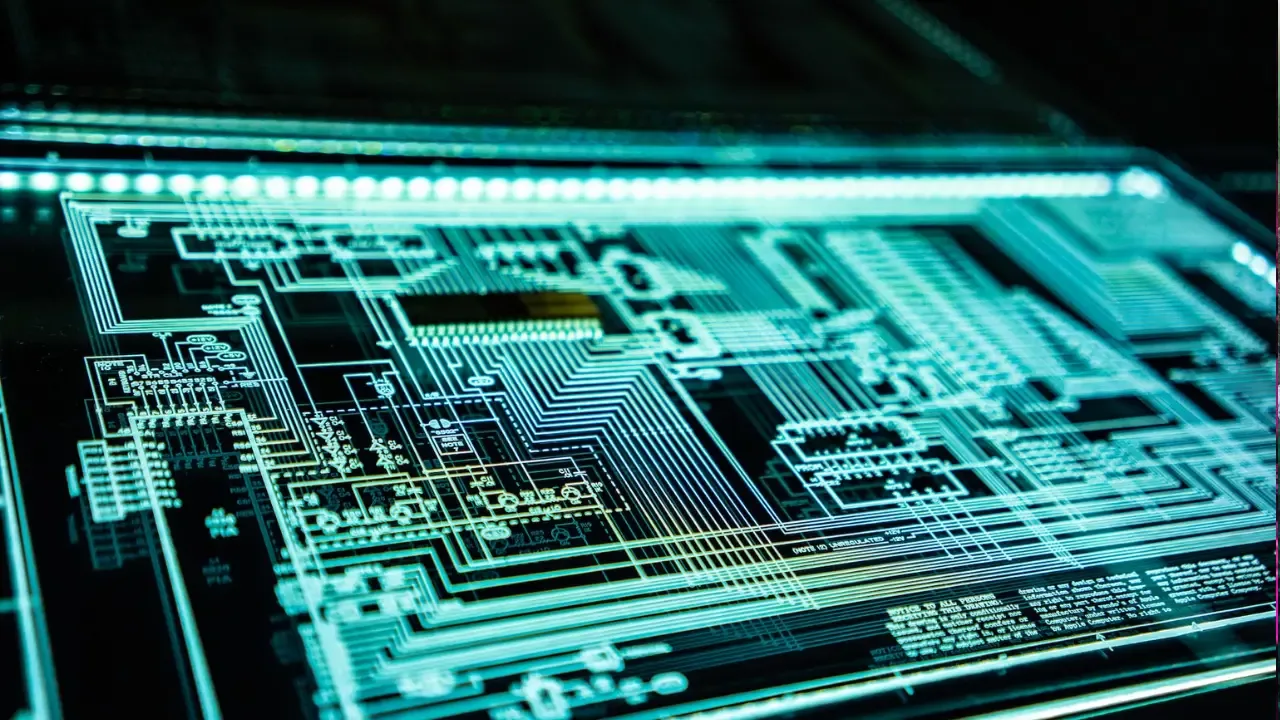
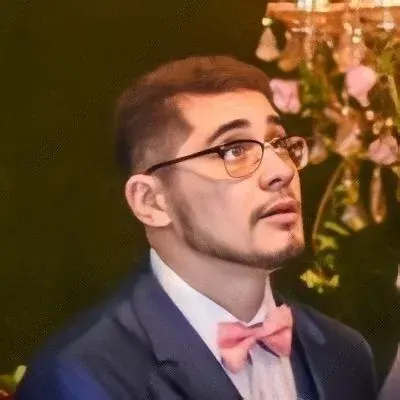
JavaScript Array: Checking if a Value Exists at a Certain Index ๐ต๏ธโโ๏ธ
Do you often find yourself wondering how to check if a value exists at a specific index in a JavaScript array? ๐ค Well, you've come to the right place! In this blog post, we'll explore the common issues surrounding this question, provide you with easy solutions, and empower you to write cleaner, more efficient code. ๐ช
The Problem ๐คจ
In the context you mentioned, the code snippet checks if the value at position index
in arrayName
is an empty string (""
). While this approach may work in some cases, it's not the best way to check if a value exists at a certain array index. ๐ฌ
The main issue with the code is that it assumes an empty string (""
) represents the absence of a value at index
. However, what if the value at index
is actually null
, undefined
, 0
, or false
? The current approach would miss those cases, leading to potential bugs and unexpected behavior.
Best Practice Solutions ๐ก
1. Check for undefined
or null
To ensure you're not missing any potential values, you can use strict equality (===
) to explicitly check for undefined
or null
at the specified index. Here's an example:
if (arrayName[index] === undefined) {
// Value is undefined
// Do stuff...
} else if (arrayName[index] === null) {
// Value is null
// Do stuff...
} else {
// There is a value at index
// Do stuff...
}
By incorporating this check, you cover both scenarios, and your code becomes more robust. ๐
2. Use the typeof
operator
Another approach is to use the typeof
operator, which allows you to determine the type of the value at index
. Here's how you can leverage it:
if (typeof arrayName[index] !== 'undefined') {
// Value is defined
// Do stuff...
} else {
// Value is undefined
// Do other stuff...
}
Using typeof
prevents potential errors and allows you to handle different scenarios based on the type of the value at index
. ๐
Call-To-Action: Engage and Learn More! ๐
Now that you have a deeper understanding of how to check if a value exists at a specific array index, why not put your newfound knowledge into practice? Try applying the suggested solutions in your own code and see the difference it makes.
Remember, writing clean and robust code is crucial for the success of any JavaScript project! If you want to learn even more awesome JavaScript tips and tricks, feel free to explore our blog for further insights.
If you have any questions or would like to share your thoughts, we'd love to hear from you! Leave a comment below and let's continue the conversation. Happy coding! ๐๐