How do I check if string contains substring?
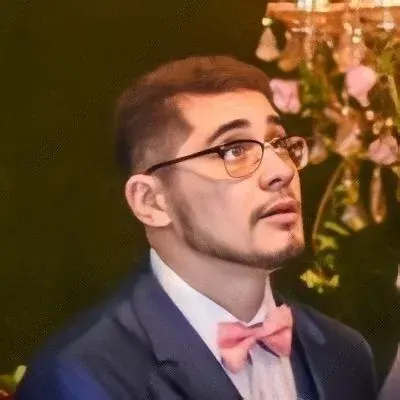
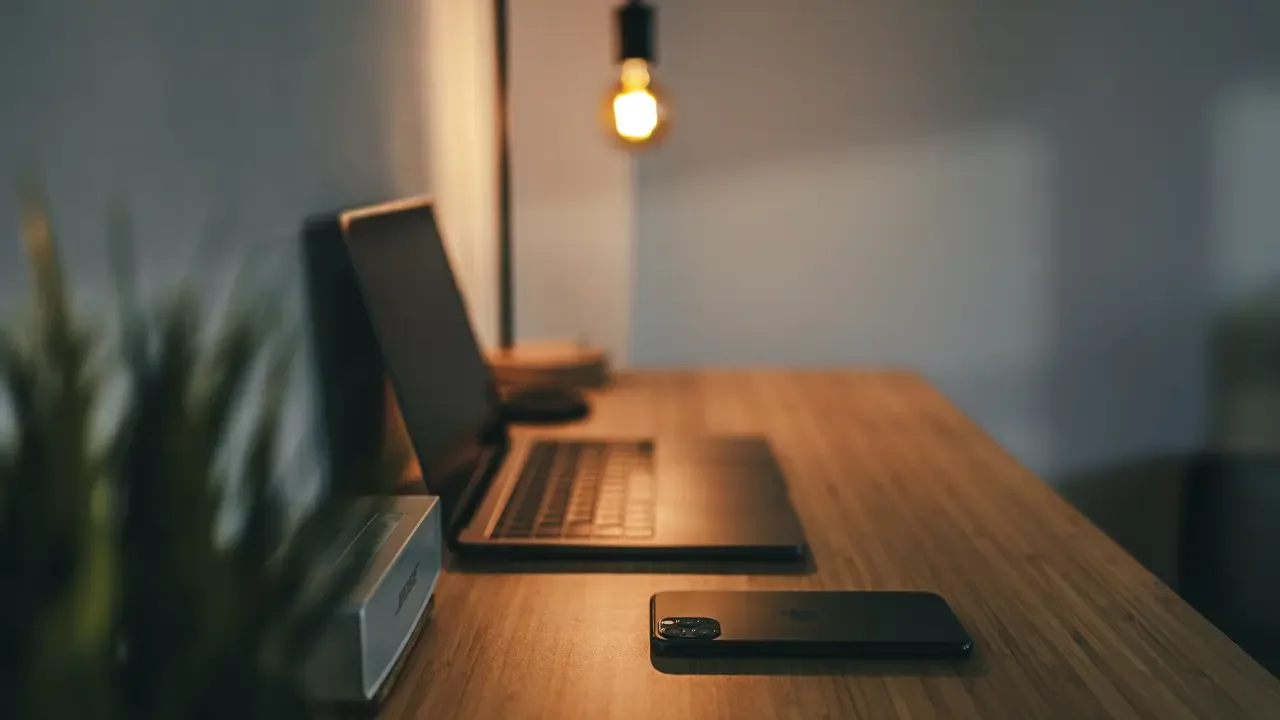
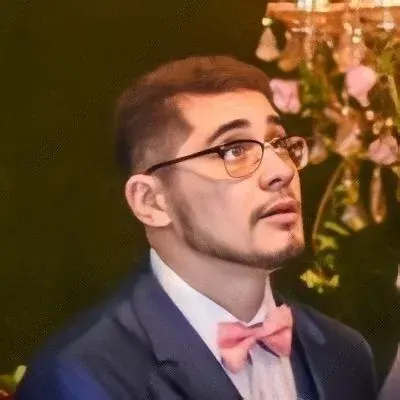
How do I check if a string contains a substring? 😕
Are you struggling with checking if a string contains a specific word or phrase? 🤔 Don't worry, you're not alone! Many developers face this challenge when working with user inputs, data extraction, or conditional statements. In this blog post, we'll tackle this issue head-on and provide you with easy solutions to meet your coding needs. Let's dive in! 🚀
The Problem: Selecting options in a shopping cart 🛒
To illustrate the problem, let's imagine that you have a shopping cart that displays product options in a dropdown menu. When a user selects "Yes" for a specific option, you want to make some additional fields on the page visible. However, there's a catch - the shopping cart includes the price modifier in the text as well, which can be different for each product. 😬
In the provided code example, you can see two different approaches used to check if the selected option is "Yes". The first one works as expected, while the second one doesn't quite hit the mark. Let's break it down and find a better solution! 💡
Solution 1: Using the ==
equality operator 🎯
The code snippet that works uses the equality operator ==
to compare the selected option with the desired value. By extracting the text from the selected option and comparing it directly to "Yes (+ $6.95)", the code determines whether to show or hide the additional fields based on the user's selection. 👍
$(document).ready(function() {
$('select[id="Engraving"]').change(function() {
var str = $('select[id="Engraving"] option:selected').text();
if (str == "Yes (+ $6.95)") {
$('.engraving').show();
} else {
$('.engraving').hide();
}
});
});
This approach works because the ==
operator checks for exact equality. If the selected option's text exactly matches "Yes (+ $6.95)", the required action is performed. However, if the text includes any deviations or additional characters, the condition fails, and the action is skipped. 🤷♂️
Solution 2: Using the includes()
method ✨
In the code that doesn't work, the intent is to use the *=
operator to check if the selected option text contains the word "Yes". Unfortunately, this operator doesn't exist in JavaScript, which is why the code fails. 😓 But fret not, we have another solution up our sleeves!
To efficiently check if a string contains a substring, you can use the includes()
method. This method returns a boolean value based on whether the substring is found in the given string. Let's take a look at how we can refactor the code using includes()
. 🔧
$(document).ready(function() {
$('select[id="Engraving"]').change(function() {
var str = $('select[id="Engraving"] option:selected').text();
if (str.includes("Yes")) {
$('.engraving').show();
} else {
$('.engraving').hide();
}
});
});
By replacing the non-existent *=
operator with includes("Yes")
, we achieve our desired result. The code will now perform the action whenever the selected option text contains the word "Yes", regardless of the price modifier or any additional characters. Awesome, right? 😎
A Word of Caution ⚠️
While using includes()
provides a simple and effective solution for most cases, it's crucial to be mindful of potential edge cases. For example, if the selected option has leading or trailing spaces, or if the capitalization of "Yes" varies, the comparison might fail. To handle such scenarios, you might consider trimming the text or converting everything to lowercase before performing the check. Adapt and personalize the solution according to your specific requirements! 👌
Time to Level Up! ⏫
You've conquered the challenge of checking if a string contains a substring! 🎉 Armed with the knowledge of equality operators and the includes()
method, you can apply this technique whenever you encounter similar situations in your code. Keep experimenting, exploring, and expanding your coding skills! 💪
If you found this article helpful, feel free to share it with your fellow developers and spread the wisdom. We love to hear your thoughts and experiences, so don't hesitate to leave a comment down below! Together, let's code better and make the tech world a little bit brighter. ✨ Happy coding! 💻