How do I check if an object has a specific property in JavaScript?
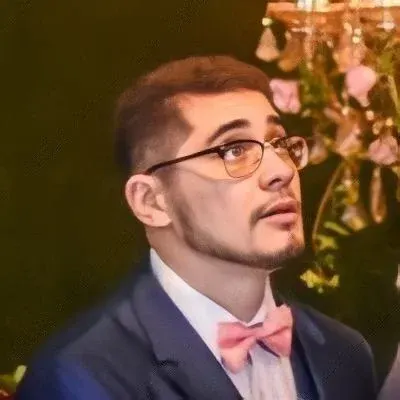
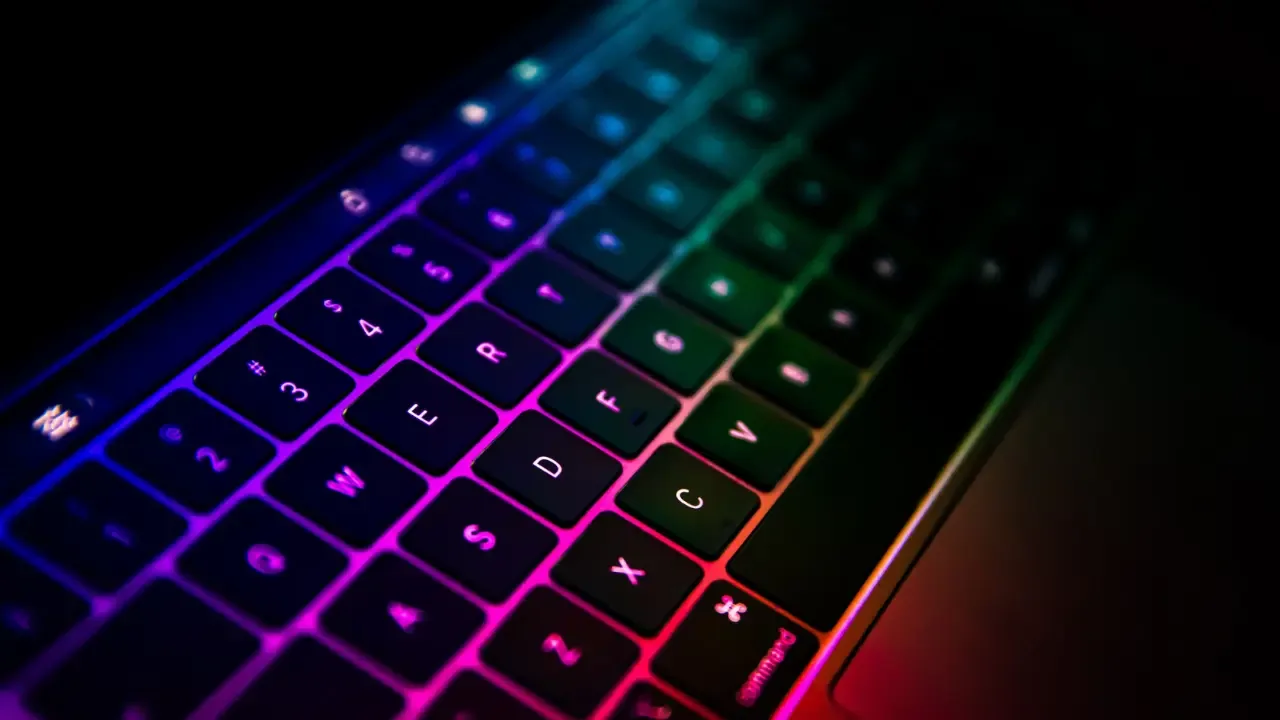
🕵️♀️ How to Check if an Object has a Specific Property in JavaScript
If you've ever found yourself wondering how to check if an object has a specific property in JavaScript, you're in the right place! 🤔 In this blog post, we'll explore a common issue faced by JavaScript developers and provide you with easy solutions. By the end, you'll have a clear understanding of how to tackle this problem. Let's dive in! 💪
🧠 Understanding the Challenge
Imagine you have an object and you want to check if it contains a particular property. Seems simple enough, right? However, JavaScript provides several ways to approach this problem, and it can be easy to get lost in the options. 🤯
💡 Easy Solution 1: Using the "hasOwnProperty()" Method
One common way to check for a specific property is by using the hasOwnProperty()
method. This method checks if the object itself has the specified property, rather than its prototype chain. Here's an example:
const obj = {
key: 1,
};
if (obj.hasOwnProperty('key')) {
// Do something
}
In this case, the hasOwnProperty()
method returns true
if the object obj
has the property 'key'
, allowing you to perform the desired actions within the if
statement. 🎉
💡 Easy Solution 2: Using the "in" Operator
Another option is to use the in
operator, which checks if a property exists in an object, including its prototype chain. Here's an example:
const obj = {
key: 1,
};
if ('key' in obj) {
// Do something
}
In this case, the in
operator returns true
if the property 'key'
is found in the object obj
. Feel free to perform your desired actions within the if
statement. 🎊
⚠️ A Word of Caution
While both solutions mentioned above work perfectly fine in most scenarios, be aware of potential limitations with the hasOwnProperty()
method and the in
operator.
If the property you're checking for happens to be named 'hasOwnProperty'
or exists in the prototype chain, unexpected results may occur. It's recommended to be cautious and consider using other approaches, such as using built-in functions like Object.keys()
or Object.getOwnPropertyNames()
.
🌞 Level Up: Using Built-in Functions
If you want to explore more advanced options, JavaScript offers built-in functions that can help you determine if an object has a specific property. Let's take a quick look at two of them:
1. Object.keys()
The Object.keys()
function returns an array containing all the enumerable properties of an object. By checking if a specific property exists in this array, you can determine if the object contains that property. Here's an example:
const obj = {
key: 1,
};
if (Object.keys(obj).includes('key')) {
// Do something
}
By using the Object.keys()
function, we can create an array of all the properties in the object obj
. Then, with the help of the includes()
method, we can check if the property 'key'
exists in that array.
2. Object.getOwnPropertyNames()
Similar to Object.keys()
, the Object.getOwnPropertyNames()
function returns an array containing all the properties of an object, including non-enumerable ones. Here's an example:
const obj = {
key: 1,
};
if (Object.getOwnPropertyNames(obj).includes('key')) {
// Do something
}
By utilizing Object.getOwnPropertyNames()
, you can check for the existence of a specific property in the resulting array.
📣 Engage with Us!
Congratulations on reaching the end of this informative blog post! We hope this guide has provided you with clear and easy solutions to check if an object has a specific property in JavaScript. 🎉
Now it's your turn to take action! Let us know which solution worked best for you and share your experiences in the comments below. If you have any further questions or ideas for future blog posts, we'd love to hear them! 😊
Stay curious, keep coding, and see you in the next blog post! Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
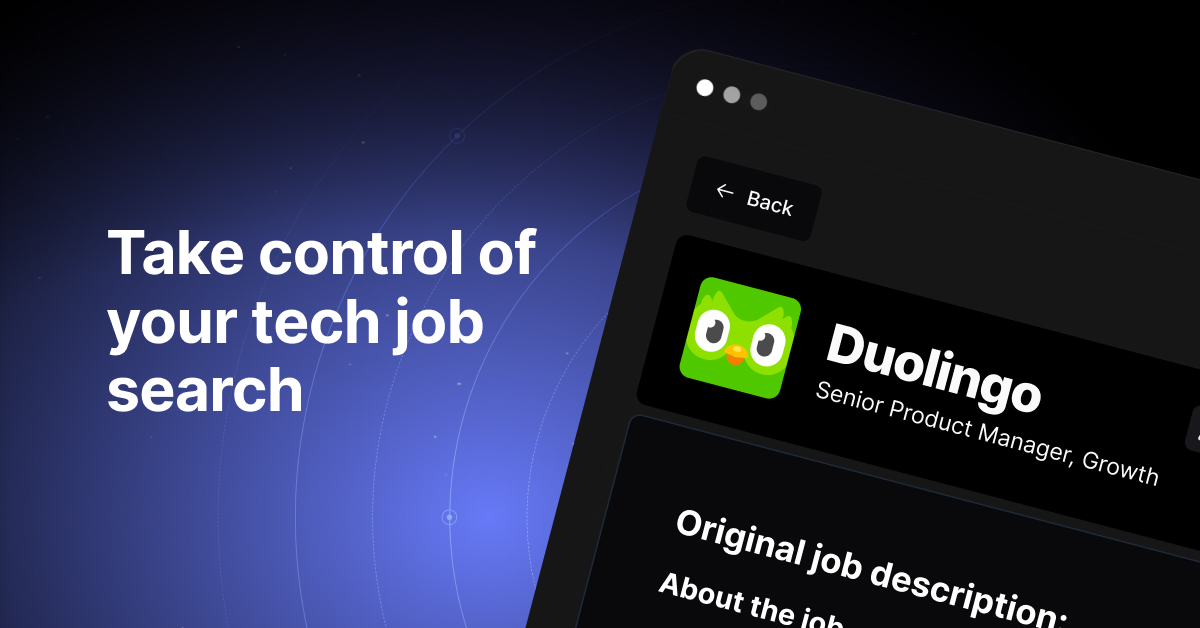