How do I check if an object has a key in JavaScript?
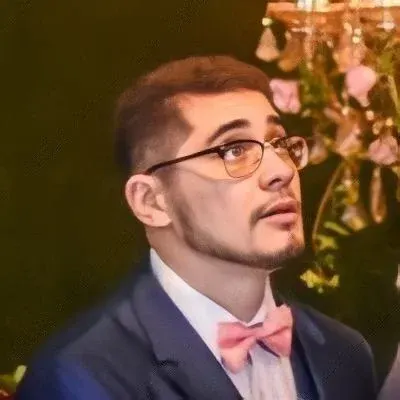
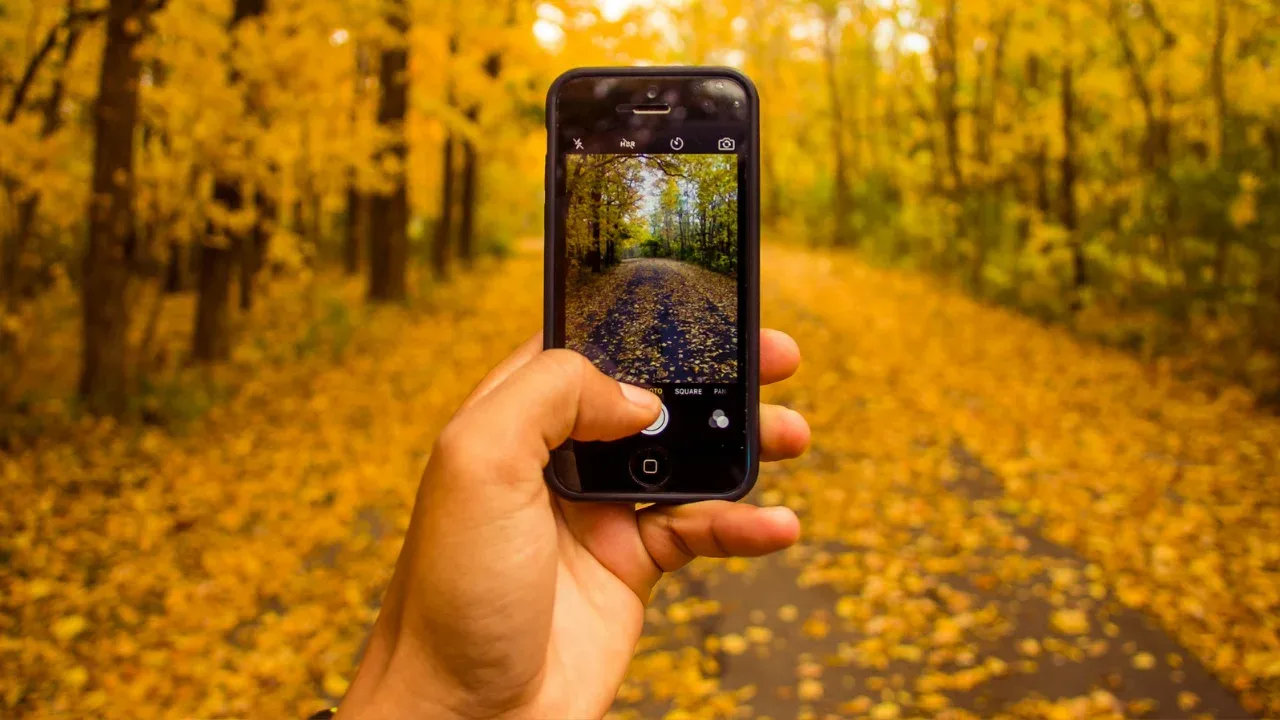
How to Check if an Object Has a Key in JavaScript 🕵️♂️
Have you ever encountered a situation where you need to check if an object has a specific key in JavaScript? It's a common problem that can be easily solved with a few lines of code. In this blog post, we'll explore different methods to tackle this issue and provide you with clear and concise solutions. Let's dive in! 💻
The Problem 😕
To understand the problem, let's consider an example:
const myObj = {
key: "value",
anotherKey: "another value"
};
Now we want to check if the object myObj
has a specific key, let's say the key is "key"
. There are multiple ways to do this, but which one is the right way? Let's examine the options presented in the context:
if (myObj['key'] == undefined)
if (myObj['key'] == null)
if (myObj['key'])
The Solutions 🛠️
Solution 1: Using the in
Operator 🎯
The in
operator can be used to check if an object has a specific key. It returns true
if the key exists in the object and false
otherwise. Here's how you can use it:
if ('key' in myObj) {
// Do something if the key exists
} else {
// Do something else if the key doesn't exist
}
This solution is concise and widely supported across different browsers and JavaScript versions. It clearly conveys the intention of checking for the existence of a key in an object.
Solution 2: Using the hasOwnProperty
Method 👀
Every object in JavaScript has a built-in method called hasOwnProperty
. This method returns true
if the object has a property with the specified key, and false
otherwise. Here's how you can utilize it:
if (myObj.hasOwnProperty('key')) {
// Do something if the key exists
} else {
// Do something else if the key doesn't exist
}
This solution is also widely supported and explicitly states the usage of hasOwnProperty
for checking object keys.
Choosing the Right Solution 🤔
Now that we have explored two solutions to check if an object has a key, you might be wondering which one is the best approach. Both solutions are viable and have their own advantages. The in
operator is more concise and readable, while the hasOwnProperty
method provides a clear indication of checking object keys.
In most cases, using the in
operator should suffice. However, if you have specific requirements or are working with objects that might inherit properties from their prototypes, the hasOwnProperty
method is a safer choice.
Conclusion and Call-to-Action ✅
Checking if an object has a key in JavaScript is an essential skill for any developer. In this blog post, we explored two easy and effective solutions to tackle this problem.
🎯 Remember, use the in
operator when you want a concise and readable solution, and opt for the hasOwnProperty
method when you require a more precise check.
Now it's your turn! Try out these solutions in your own code and let us know your thoughts in the comments. Do you have any other methods for checking object keys? We'd love to hear from you! 💬👇
Keep coding and stay curious! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
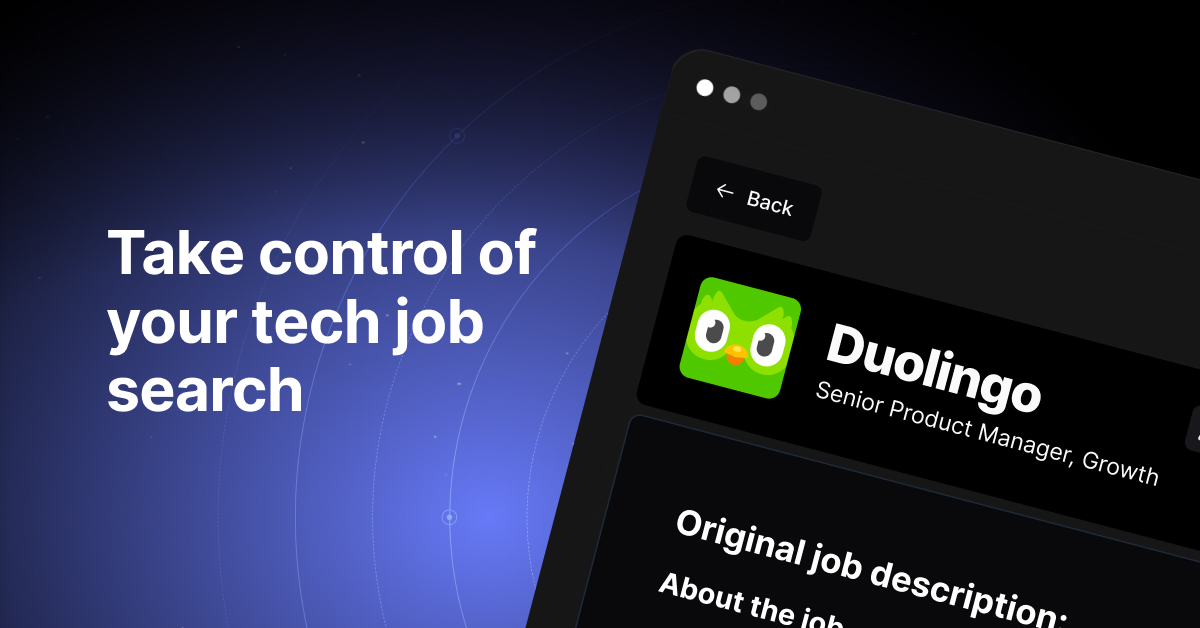