How do I check if an array includes a value in JavaScript?
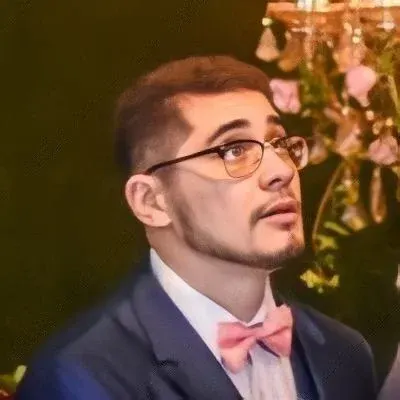
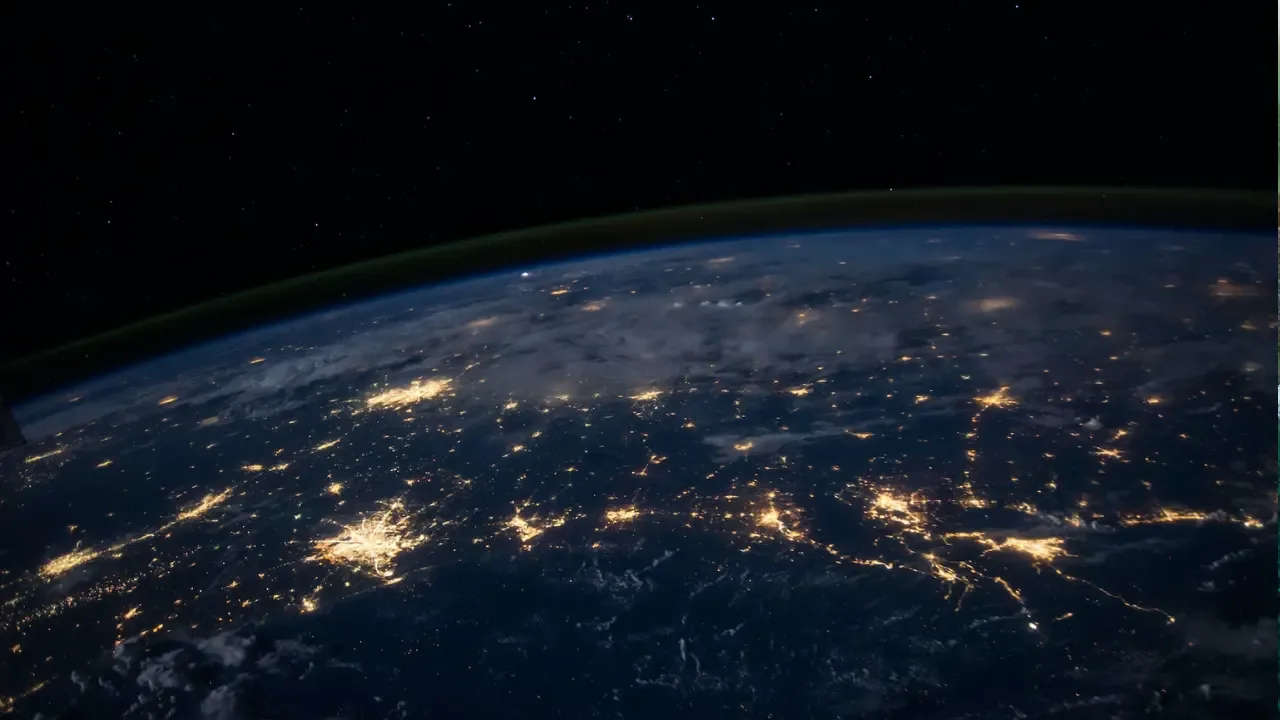
🕵️♀️ How to Check if an Array Includes a Value in JavaScript?
So, you want to spice up your JavaScript skills and learn how to check if an array contains a specific value? Look no further! In this guide, we'll dive into common issues, provide straightforward solutions, and have some fun along the way. Let's get started! 🚀
🤔 The Problem
To begin, let's take a look at the context that sparked this question. We have an existing function that checks if an array contains a value. However, there may be a better and more concise solution out there. Can we optimize this process and save some mental energy? Let's find out!
💡 The Solution
One popular method to check if an array includes a value in JavaScript is to use the Array.includes()
method. This method returns true
if the array contains the specified value, and false
otherwise.
const fruits = ['apple', 'banana', 'mango'];
console.log(fruits.includes('banana')); // Output: true
console.log(fruits.includes('orange')); // Output: false
Simple, right? The Array.includes()
method takes care of the loop and condition checks for you, making your life a whole lot easier! 🙌
🧠 Examples and Explanation
Let's dive a bit deeper into some examples to solidify our understanding. Check out the following code snippet:
const numbers = [1, 2, 3, 4, 5];
console.log(numbers.includes(3)); // Output: true
console.log(numbers.includes(6)); // Output: false
In the first example, we check if the value 3
exists in the numbers
array. Since it does, the Array.includes()
method returns true
.
On the other hand, in the second example, we check if the value 6
exists in the numbers
array. Since it doesn't, the method returns false
.
🤟 Get Even More with Stack Overflow
If you're looking for additional information or alternative approaches, check out the Stack Overflow question Best way to find an item in a JavaScript Array?. It delves into finding objects in an array using the Array.indexOf()
method, which can be another useful tool in your coding arsenal!
⏭️ Conclusion
Congratulations, you're now equipped with a powerful technique to check if an array includes a value in JavaScript! 🎉 By utilizing the Array.includes()
method, you can shorten your code while maintaining efficiency.
So, what are you waiting for? Go forth, conquer your coding challenges, and spread the word to fellow developers! Don't forget to share this post if you found it helpful. 💌
Let the array exploration begin! 💪
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
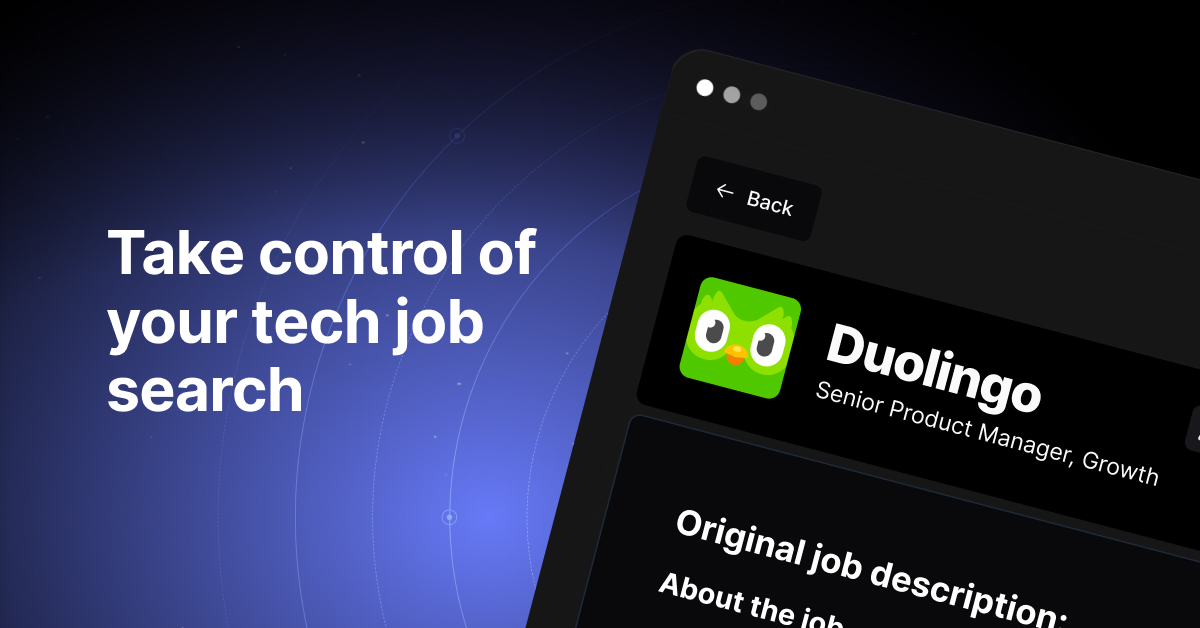