How do I check if a variable is an array in JavaScript?
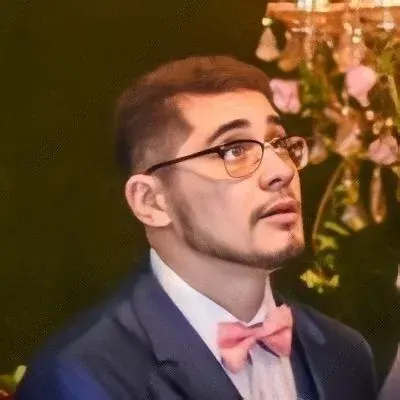
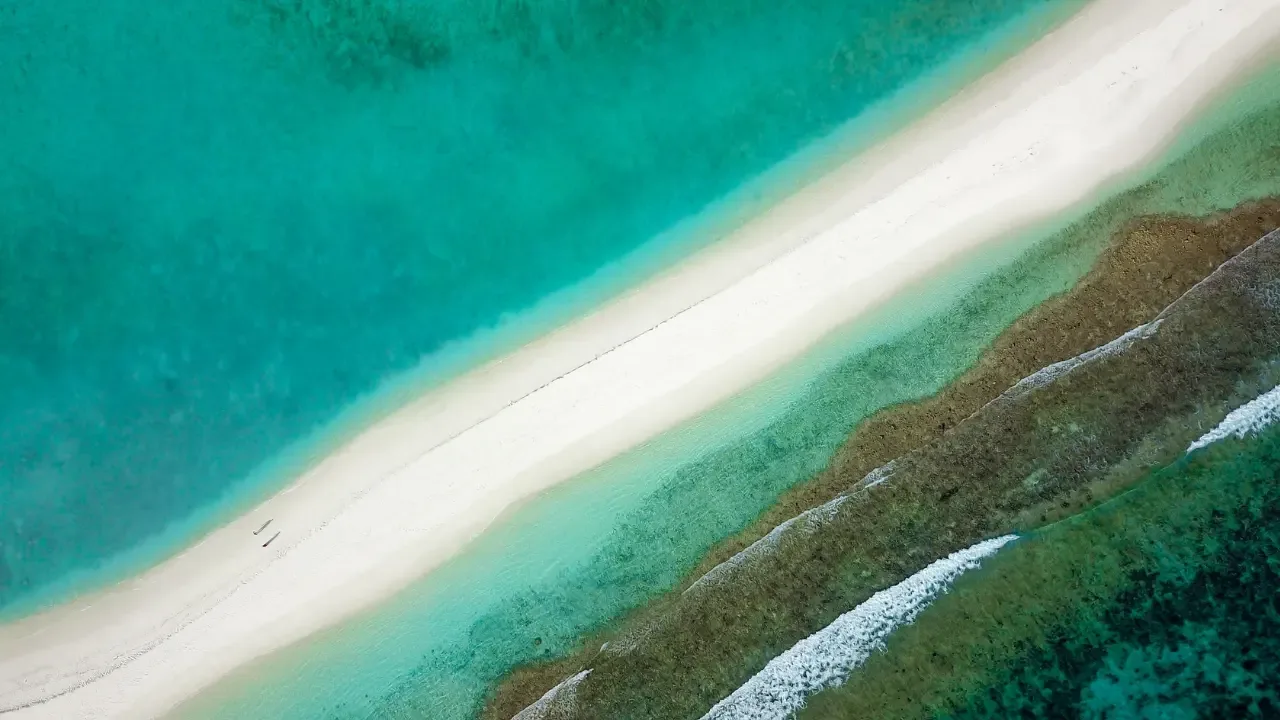
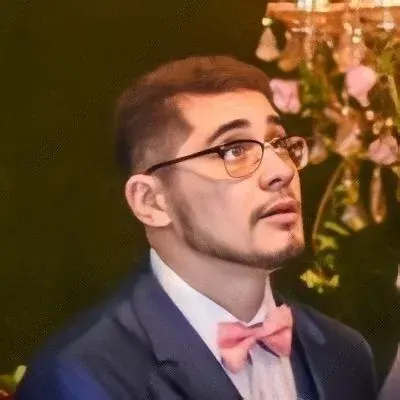
š Blog Post: "Is it an Array or Nah? Check Variables with JavaScript!"
š Hey there, JavaScript enthusiasts! Have you ever found yourself scratching your head wondering how to check if a variable is an array in JavaScript? š¤ Don't worry, you're not alone! It's a common stumbling block that can slow down your progress. But fear not, because I'm here to guide you through this seemingly thorny maze with ease! š
š” Let's dive right into the question at hand: "How do I check if a variable is an array in JavaScript?" Initially, you might think that comparing the constructor of the variable with the Array object would be a valid approach. Something like this:
if (variable.constructor == Array) {
console.log("It's an array!");
} else {
console.log("It's not an array.");
}
š§ Seems straightforward, right? But here's the catch: this method might not always give you the desired outcome. šÆ JavaScript has its quirks, and relying solely on the constructor property can lead to unexpected results. Let me show you an example:
const myVariable = { constructor: Array };
if (myVariable.constructor == Array) {
console.log("It's an array!"); // Oops! This will be executed incorrectly.
} else {
console.log("It's not an array.");
}
š Wow, that didn't go as planned! Even though myVariable is not an array, it has a constructor
property set to Array
, which confuses our initial check. That's why it's crucial to consider alternative methods to avoid falling into this trap. š
š So, what can we do to more reliably check if a variable is an array? JavaScript comes to the rescue with the Array.isArray()
method! š It's a built-in function specifically designed for this purpose. Take a look:
if (Array.isArray(variable)) {
console.log("It's an array!");
} else {
console.log("It's not an array.");
}
š This approach saves the day! Array.isArray()
is a foolproof way to determine whether a variable is an array. The method returns true
if the variable is an array, and false
otherwise. No more guesswork!
š Congratulations, you're now equipped with the knowledge to confidently check if a variable is an array in JavaScript! š But wait, there's more!
š£ I'd love to hear your thoughts! Which method do you prefer for checking arrays? Have you encountered any challenges along the way? Share your experiences and join the conversation in the comments below. Let's learn and grow together as a community! šŖāØ
š¢ Remember to share this blog post with your fellow JavaScript enthusiasts who might be struggling with this issue. Together, we can make coding easier and more enjoyable for everyone! š
Keep coding and stay curious! šš»
Your friendly neighborhood tech writer ššļø