How do I call an Angular 2 pipe with multiple arguments?
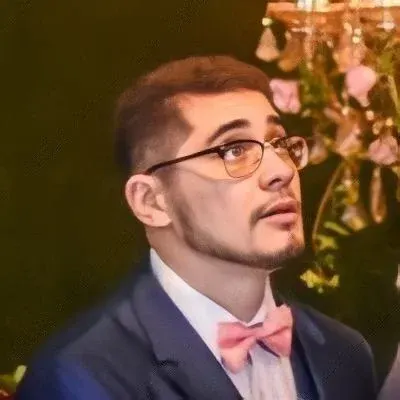
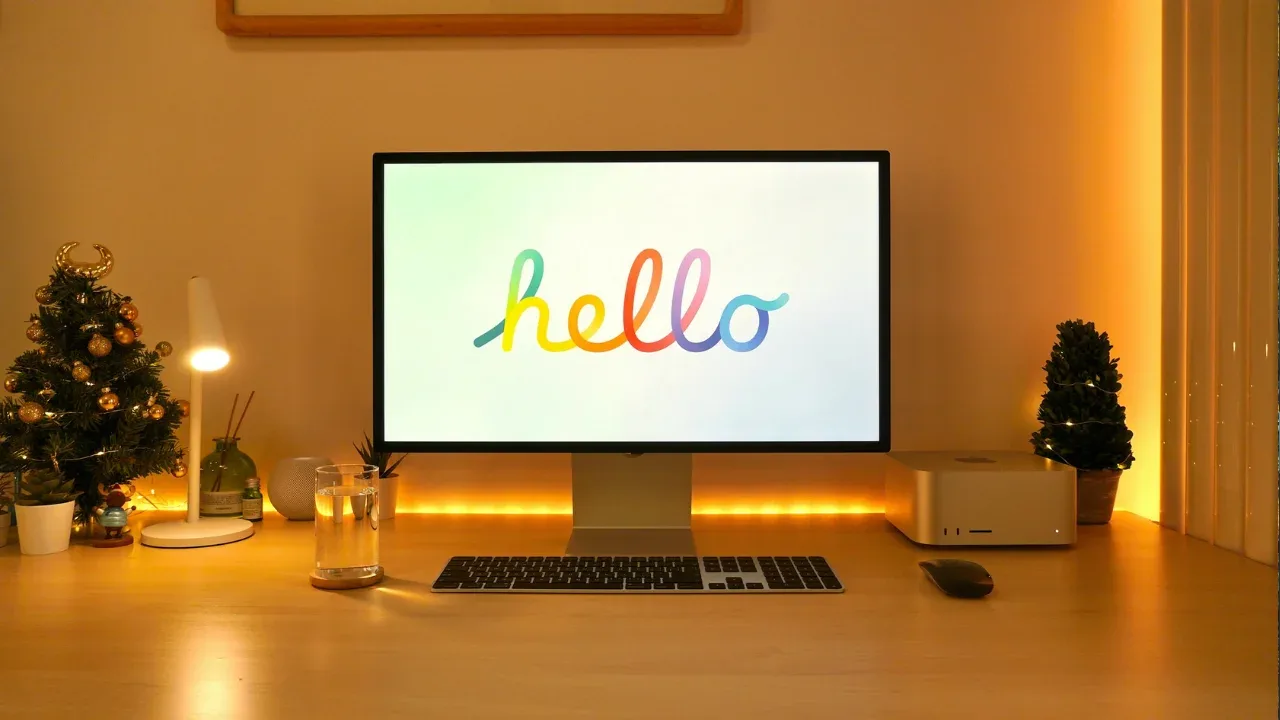
Calling an Angular 2 Pipe with Multiple Arguments: The Complete Guide 😎🔧
So, you're working with Angular 2 and you've stumbled upon an issue. You know how to call a pipe with a single argument, but you're not sure how to call a pipe with multiple arguments. Fear not! In this guide, we'll walk you through the process step by step. Let's dive in! 💪
1. Understanding the Syntax of Angular 2 Pipes
Before we jump into the solution, let's quickly recap the syntax of Angular 2 pipes. Pipes are used to transform data within a template. You can apply a pipe by using the |
symbol followed by the pipe name and any additional arguments. For example, to format a date using the date
pipe, you would write:
{{ myData | date:'fullDate' }}
In this case, the date
pipe takes a single argument, fullDate
, which specifies the format of the date.
2. Calling a Pipe with Multiple Arguments in the Template
Now, let's move on to calling a pipe with multiple arguments in the component's template HTML. To achieve this, you can pass additional arguments separated by colons. Let's say we want to format a date and time using the date
pipe, and we also want to provide the time zone as an argument. Here's how you would do it:
{{ myData | date:'fullDate':'shortTime':'EST' }}
In this example, we pass three arguments to the date
pipe:
'fullDate'
specifies the format of the date.'shortTime'
specifies the format of the time.'EST'
specifies the time zone.
By separating the arguments with colons, we can pass as many arguments as we need to the pipe.
3. Calling a Pipe with Multiple Arguments in Code
Calling a pipe with multiple arguments directly in code is slightly different than in the template. To achieve this, we need to leverage Angular's PipeTransform
interface.
First, import the PipeTransform
and Pipe
classes from the @angular/core
package:
import { PipeTransform, Pipe } from '@angular/core';
Next, create a custom pipe that implements the PipeTransform
interface:
@Pipe({
name: 'customPipe'
})
export class CustomPipe implements PipeTransform {
transform(value: any, arg1: any, arg2: any, arg3: any): any {
// Perform your logic here using the provided arguments
}
}
In the transform
method, you can access the passed arguments (arg1
, arg2
, arg3
, and so on) and perform your desired logic. Don't forget to return the transformed value!
Now, you can use your custom pipe with multiple arguments in your code:
const transformedValue = new CustomPipe().transform(myData, arg1, arg2, arg3);
You're all set! Time to shine! ✨
Congrats! You now know how to call an Angular 2 pipe with multiple arguments both in the template and directly in code. Feel free to experiment and explore the power of pipes to transform your data in innovative ways! 💡
If you found this guide helpful, don't hesitate to share it with your fellow developers on social media. And remember, always stay curious and keep coding! 🚀💻
Was this guide helpful? Did you encounter any issues or have any suggestions? We'd love to hear from you! Let us know in the comments below. Happy coding! 👇😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
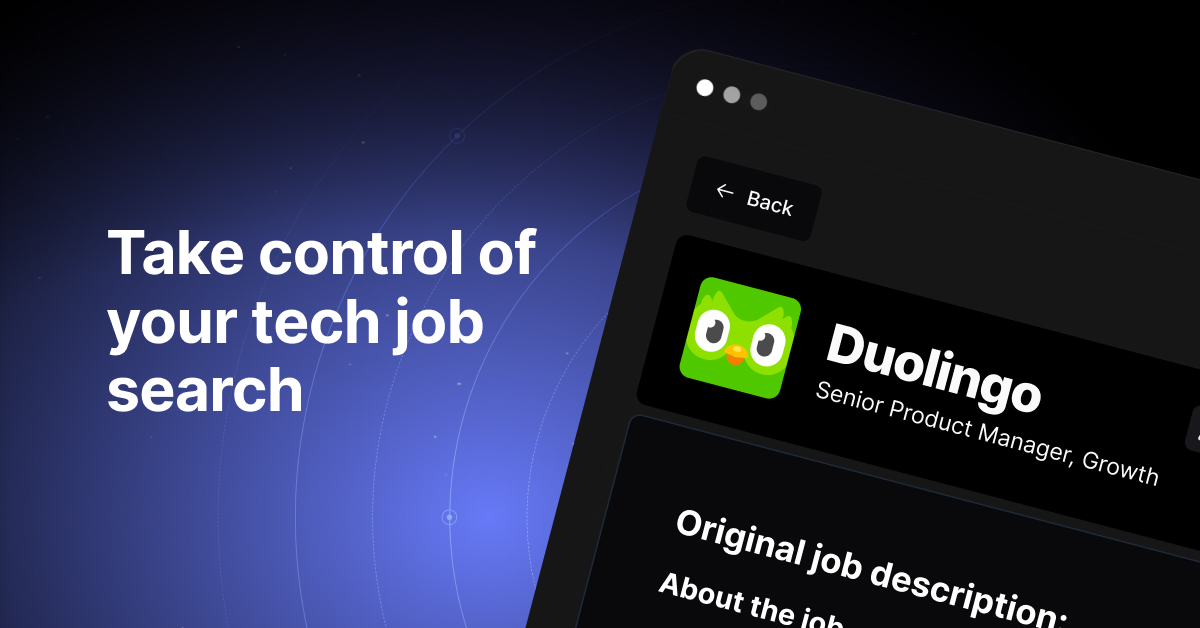