How do I attach events to dynamic HTML elements with jQuery?
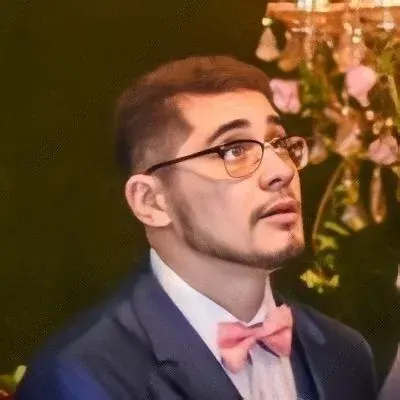
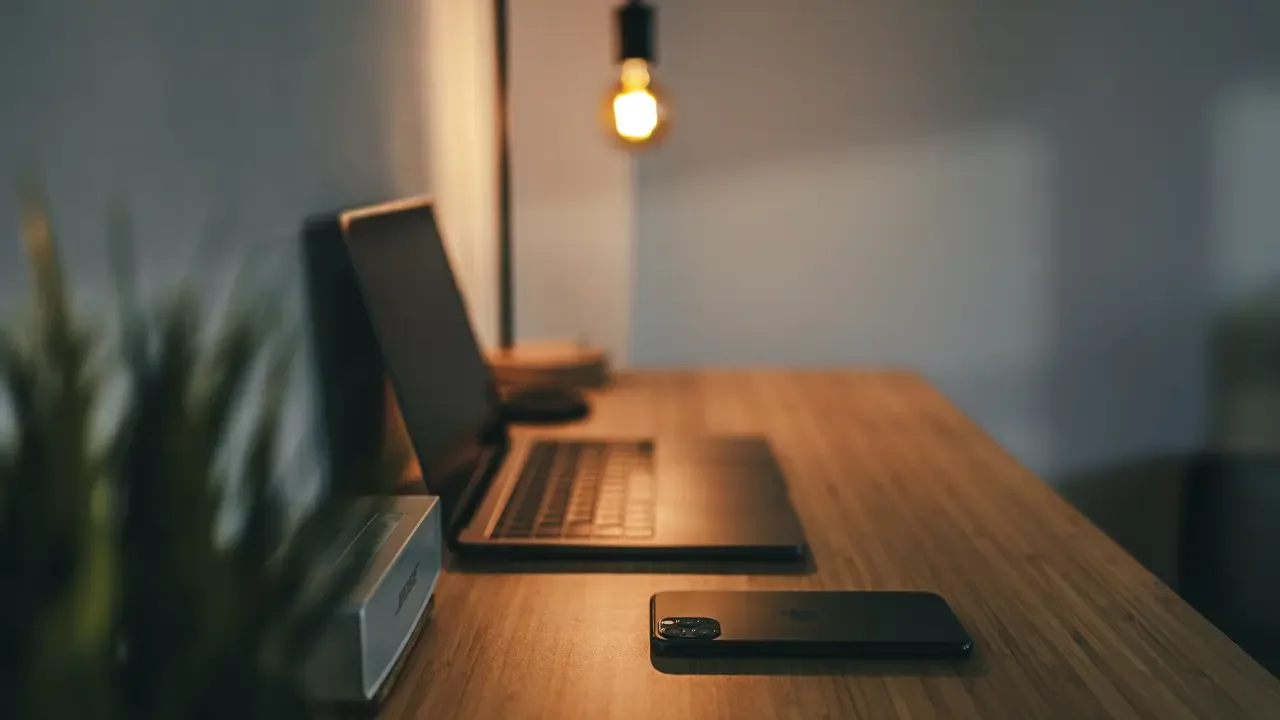
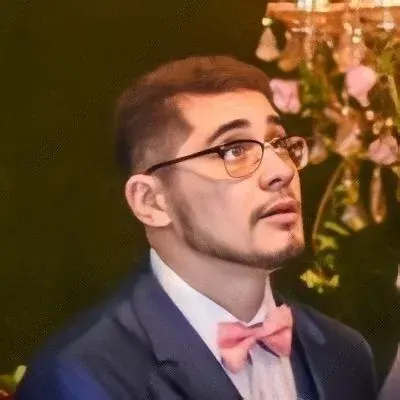
How to Attach Events to Dynamic HTML Elements with jQuery π»ππ‘
If you've ever found yourself wondering how to attach events to dynamic HTML elements with jQuery, you're not alone! It can be a bit tricky to ensure that your event handler applies to both elements present at page load and elements that are added dynamically later on, like through AJAX or DHTML. π€
Let's take a look at a common scenario to illustrate the issue. Suppose you have a jQuery code snippet that attaches a click event handler to all elements with the class .myclass
. Here's an example of how that might look:
$(function(){
$(".myclass").click(function() {
// do something
});
});
In your HTML, you might have a few elements with the class .myclass
, like this:
<a class="myclass" href="#">test1</a>
<a class="myclass" href="#">test2</a>
<a class="myclass" href="#">test3</a>
And that works perfectly fine! The click event is properly attached to each .myclass
element. However, things get a bit more complicated when you want to add a new element dynamically, after the page has loaded. π¬
Let's say you have an anchor
element with the id #anchor1
, and you want to add a new .myclass
link when this anchor element is clicked. Here's an example of how you might approach it:
<a id="anchor1" href="#">create link dynamically</a>
<script type="text/javascript">
$(function(){
$("#anchor1").click(function() {
$("#anchor1").append('<a class="myclass" href="#">test4</a>');
});
});
</script>
The problem arises when the new element, in this case, test4
, is created. Even though it has the class .myclass
, it doesn't have the click event handler associated with it. π
So, how do we solve this issue? Fear not, my fellow developer! There's a simple solution that will allow you to write the click()
handler once and have it apply to both initially loaded content and dynamically added content. π
The on()
Method to the Rescue! π¦ΈββοΈπ₯
The key to attaching events to dynamic HTML elements with jQuery lies in using the on()
method. Unlike the click()
method, which only attaches the event handler to elements that existed when the code ran, the on()
method allows you to attach an event handler to current and future elements.
Let's update our previous code example to use the on()
method instead:
$(function(){
$(document).on('click', '.myclass', function() {
// do something
});
});
By binding the click event to the document
and specifying the .myclass
selector as the second argument, we ensure that the click event handler will be triggered for both existing and dynamically added elements with the class .myclass
. π
Putting it All Together π¨βπ»π§
To summarize, here are the steps you need to follow to attach events to dynamic HTML elements with jQuery:
Use the
on()
method instead of theclick()
method to attach the event handler.
$(function(){
$(document).on('click', '.myclass', function() {
// do something
});
});
Replace
document
with the closest ancestor element that will contain all the elements you want the event to apply to. This will improve performance by limiting the event handling to a specific section of the page.
$(function(){
$('#container').on('click', '.myclass', function() {
// do something
});
});
By utilizing the on()
method and incorporating it into your jQuery code, you can ensure that event handlers are attached to both existing and dynamically added elements. No more headaches when dealing with dynamic content! π
Now it's your turn to give it a try! Apply this newfound knowledge to your own projects and see how it transforms your event handling capabilities. Don't forget to share your success stories in the comments below! π¬π
π Happy coding! π¨βπ»πͺ
Have any other jQuery questions or topics you'd like us to cover? Let us know! We love hearing from our readers and providing helpful tech guides like this one. βοΈππ