How can you sort an array without mutating the original array?
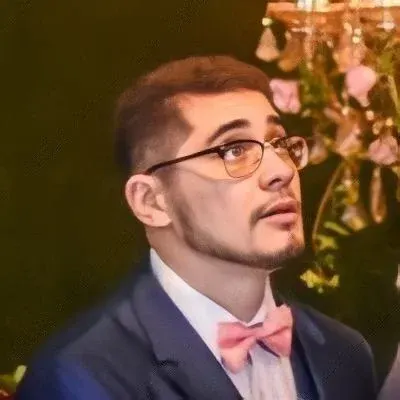

Sorting an Array Without Mutating the Original Array: A Complete Guide
Sorting an array is a commonly used operation in programming. However, what if you want to sort an array without actually mutating, or changing, the original array? In this blog post, we will explore this question and provide easy solutions to tackle this problem. So, let's dive in and find a straightforward way around this issue!
Understanding the Problem
To illustrate the issue, let's consider the following code snippet:
function sort(arr) {
return arr.sort();
}
var a = [2,3,7,5,3,7,1,3,4];
sort(a);
alert(a); //alerts "1,2,3,3,3,4,5,7,7"
In the above example, calling the sort
function on array a
leads to the mutation of the original array, which is not the desired behavior. Additionally, using Array.prototype.sort()
method like this:
function sort(arr) {
return Array.prototype.sort(arr);
}
doesn't work as expected.
The Solution: Array Spread Operator
Fortunately, there is a simple and elegant solution that doesn't involve writing your own sorting algorithm or copying every element of the array into a new one. Enter the Array Spread Operator!
The Array Spread Operator allows us to create a new array that includes all the elements of the original array. By combining it with the sort
method, we can achieve the desired effect of returning a sorted copy without modifying the original array.
Let's modify our sort
function to use the Array Spread Operator:
function sort(arr) {
return [...arr].sort();
}
Now, if we test our modified sort
function with the same example as before, we get the expected result:
var a = [2,3,7,5,3,7,1,3,4];
var sortedA = sort(a);
alert(a); //alerts "2,3,7,5,3,7,1,3,4"
alert(sortedA); //alerts "1,2,3,3,3,4,5,7,7"
Voilà! The original array remains unchanged, and we have a sorted copy in sortedA
.
Share Your Experience
Try implementing this solution in your own code and see how it works for you. We would love to hear about your experience and any other questions or issues you encounter. Join the conversation by leaving a comment below!
Conclusion
Sorting an array without mutating the original array is a common problem faced by many programmers. By using the Array Spread Operator in conjunction with the sort
method, we can easily create a sorted copy of the array while leaving the original array untouched. Remember to spread the love and share this solution with your fellow developers!
That's all for now! Stay tuned for more helpful tips and tricks in our next blog post. And remember, keep coding... 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
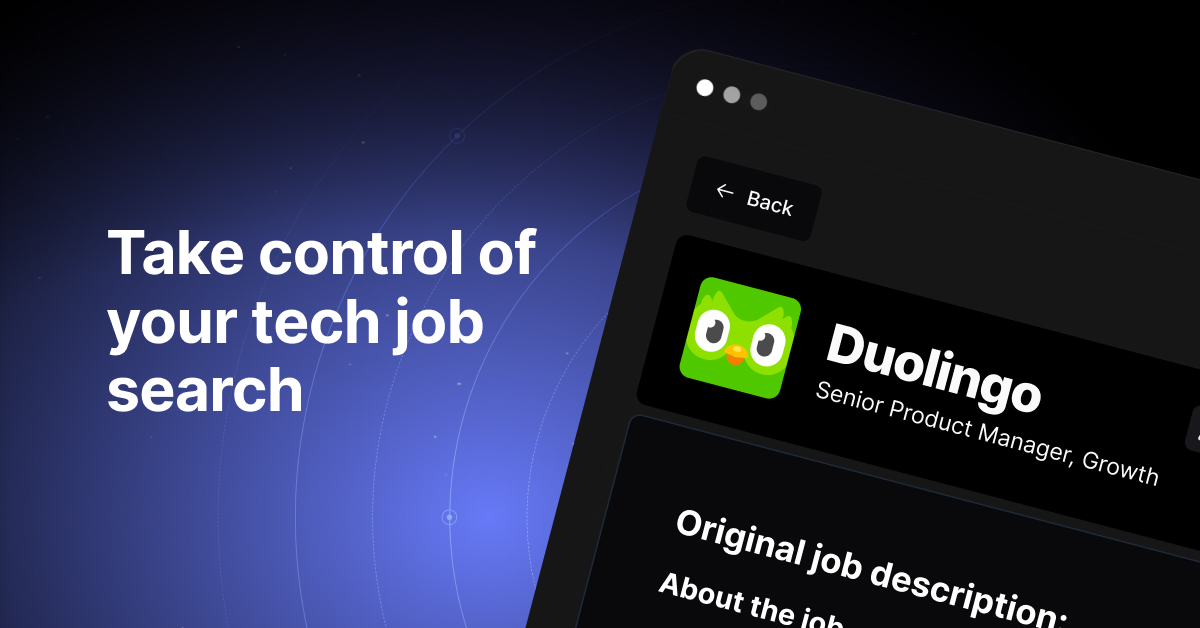