How can you check for a #hash in a URL using JavaScript?
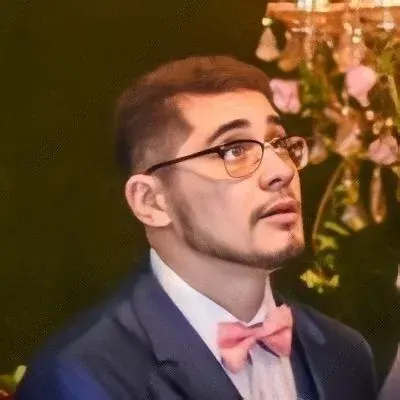
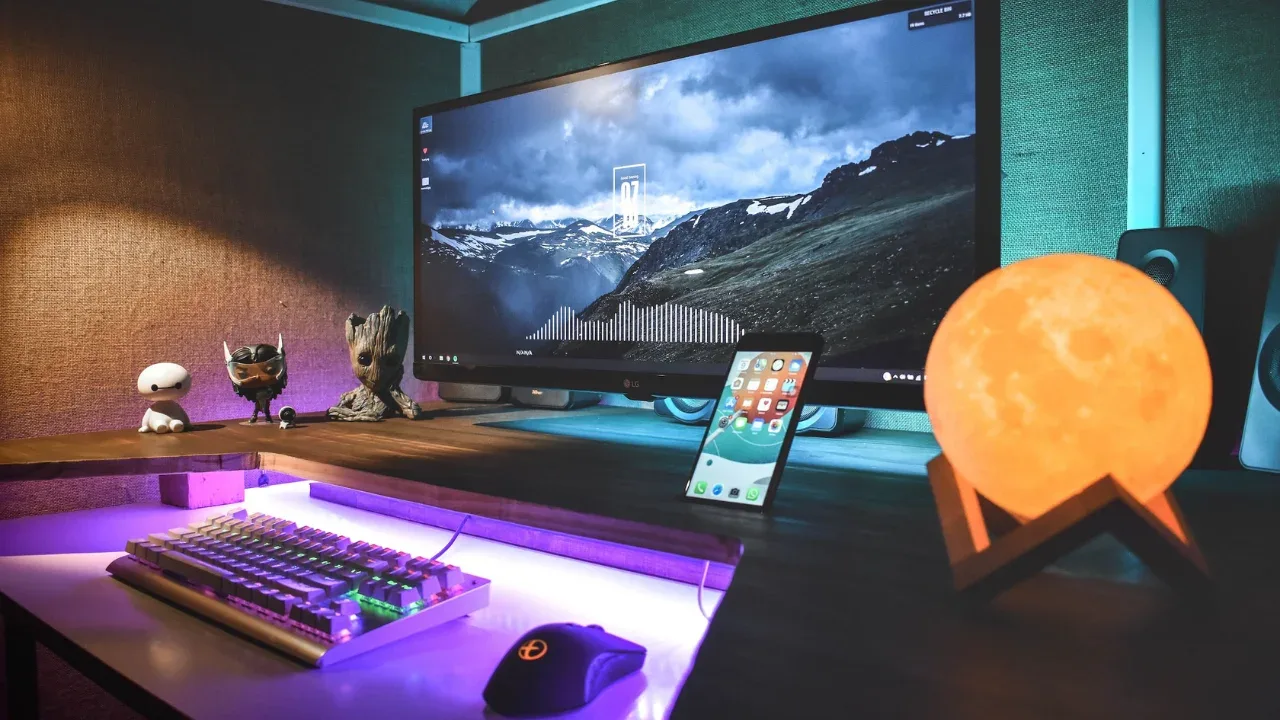
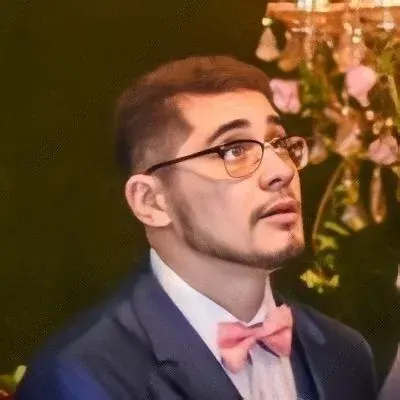
🔍 How to Check for a #hash in a URL using JavaScript
Hey there! 👋 Are you struggling to find a simple way to check if a URL contains a #hash in JavaScript? Don't worry, I've got you covered! In this blog post, I'll walk you through common issues and provide easy solutions to help you detect that pesky #hash in a URL. Let's dive in! 💪
The Problem: Checking for a #hash in a URL
So, you want to run some JavaScript code only when there is a #hash anchor link in a URL. And you're looking for a catch-all test to detect URLs like these:
example.com/page.html#anchor
example.com/page.html#anotheranchor
You're thinking something like this:
if (thereIsAHashInTheUrl) {
doThis();
} else {
doThat();
}
The Solution: Using JavaScript to Check for a #hash
Luckily, JavaScript provides a convenient way to check for a #hash in a URL. 🎉
Here's a simple and effective method:
if (window.location.hash) {
doThis(); // Execute your code when there is a #hash in the URL
} else {
doThat(); // Execute your code when there is no #hash in the URL
}
By using the window.location.hash
property, you can access the #hash portion of the URL. If it exists, this property will have a truthy value, triggering the doThis()
function. If not, it will be falsy, prompting the execution of doThat()
.
Easy, right? 🙌
Let's break it down:
window.location.hash
returns the #hash portion of the URL.The condition
if (window.location.hash)
checks if the #hash exists.If the #hash exists,
doThis()
will be executed.If the #hash doesn't exist,
doThat()
will be executed.
An Example to Make It Crystal Clear
To bring it all together, let's see an example of how this would work in practice:
<!DOCTYPE html>
<html>
<head>
<script>
function doThis() {
console.log("Executing code for #hash in the URL");
}
function doThat() {
console.log("Executing code for no #hash in the URL");
}
if (window.location.hash) {
doThis();
} else {
doThat();
}
</script>
</head>
<body>
<!-- Your awesome website content goes here -->
</body>
</html>
When you run this code in your web browser, open the console, and navigate to a URL with a #hash, you'll see the corresponding message:
For a URL like example.com/page.html#anchor, it will log: "Executing code for #hash in the URL"
For a URL like example.com/page.html, it will log: "Executing code for no #hash in the URL"
And there you have it! 🎉 You can now easily check for a #hash in a URL using JavaScript.
Get Coding and Share Your Experience!
Now that you have a clear solution, it's time to put it into practice. Go ahead and add your custom code to the doThis()
and doThat()
functions and unlock endless possibilities. Feel free to share your experience or ask any questions in the comments below.
Remember, the tech world is all about collaboration! So, don't forget to share this blog post with your fellow developers who might be facing the same challenge. Together, we can make JavaScript coding easier for everyone! 🤝
Happy coding! 💻✨