How can I write a test which expects an "Error" to be thrown in Jasmine?
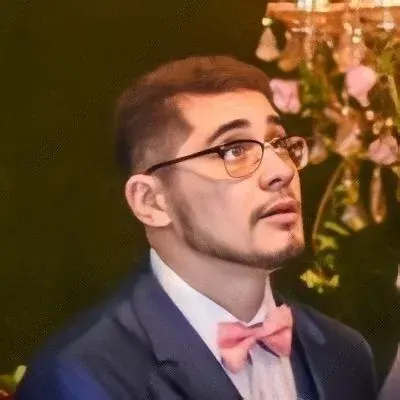
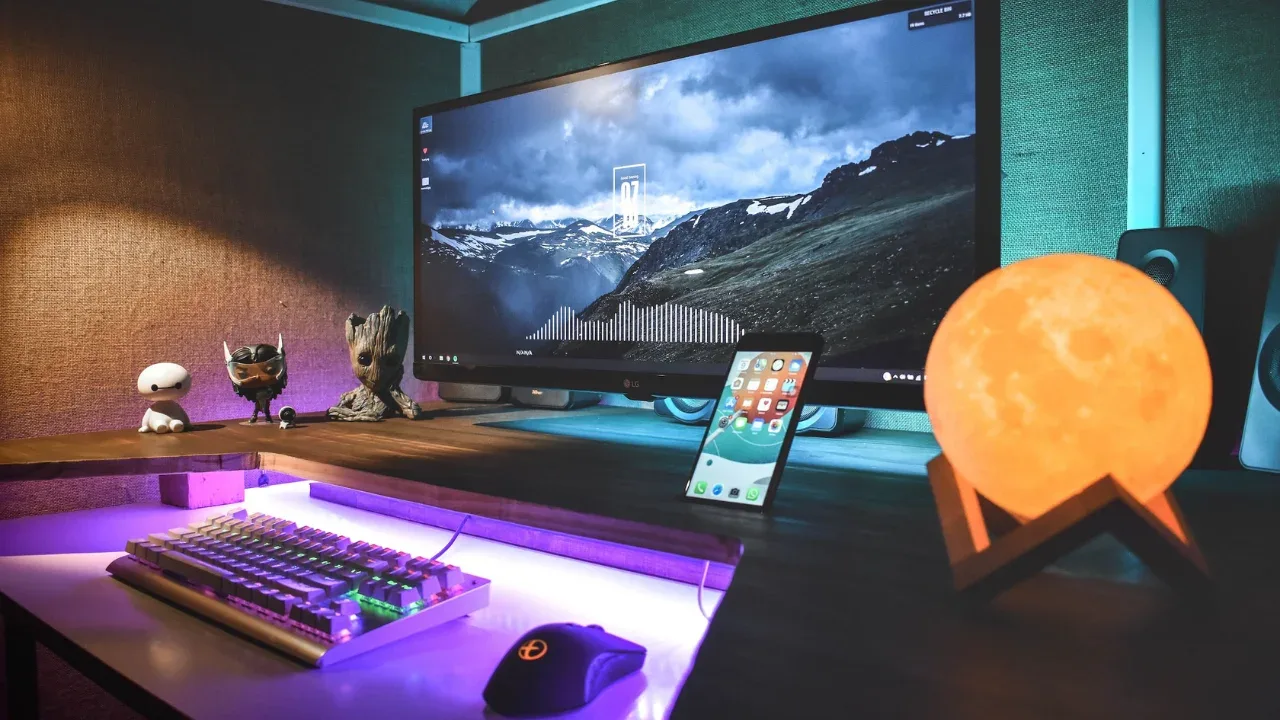
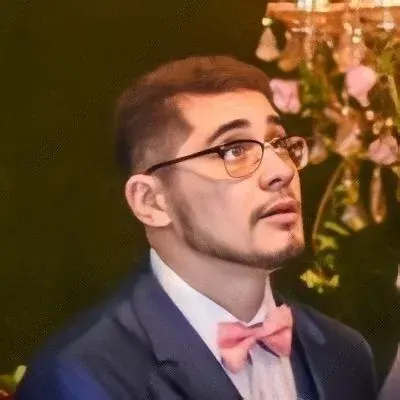
How to Write a Jasmine Test that Expects an 'Error' to Be Thrown ๐ฑ
Writing tests is an essential part of the development process, ensuring that our code functions as expected. But what happens when we want to test if an error is thrown? ๐ค In this post, we'll explore how you can write a test in Jasmine that expects an 'error' to be thrown, and we'll address some common issues you might encounter along the way.
The Conundrum ๐คท
Let's start by setting the scene. You're writing a test for a Node.js module using Jasmine, and you want to verify that a certain error is thrown correctly. Your code looks something like this:
throw new Error("Parsing is not possible");
And your test code is as follows:
describe('my suite...', function() {
// ...
it('should not parse foo', function() {
// ...
expect(parser.parse(raw)).toThrow(new Error("Parsing is not possible"));
});
});
However, no matter what you try, the test keeps failing. ๐ซ
The Issue: Incorrect Syntax โ
The problem lies in the syntax you're using for the toThrow
matcher. The correct syntax is not what you might expect. Instead of passing the error message directly, you need to pass a function that throws the error.
The Solution: Wrapped Function ๐
To solve this issue, we need to wrap the code that throws the error in a function. Here's how you can do it:
describe('my suite...', function() {
// ...
it('should not parse foo', function() {
// ...
expect(function() {
parser.parse(raw);
}).toThrow(new Error("Parsing is not possible"));
});
});
By wrapping the code that throws the error in an anonymous function, we can now correctly assert that the error is thrown.
Sidenote: Async Code
If you're dealing with asynchronous code and want to test if an error is thrown, you also need to handle Promise rejections appropriately. Here's an example:
describe('my asynchronous suite...', function() {
// ...
it('should handle errors', async function() {
// ...
await expectAsync(someAsyncFunction()).toBeRejectedWith(new Error("Some error"));
});
});
In the above example, we use the expectAsync
function to handle the Promise rejection and check if the error matches our expectation.
Try it out! ๐ ๏ธ
Now that you know how to write a Jasmine test that expects an 'error' to be thrown, it's time to put it into practice. Open up your editor, fix the syntax as we mentioned earlier, and run your tests. ๐โโ๏ธ
Conclusion ๐
Writing tests that handle errors is an important aspect of software development. With Jasmine, it's easy to write tests that expect errors to be thrown once you understand the correct syntax. Remember to wrap your code in a function and use the toThrow
matcher correctly. ๐งช
So go ahead and level up your testing game by including error handling in your Jasmine tests. And don't forget to share this post with your fellow developers who might be struggling with the same issue. Happy testing! ๐