How can I wait In Node.js (JavaScript)? l need to pause for a period of time
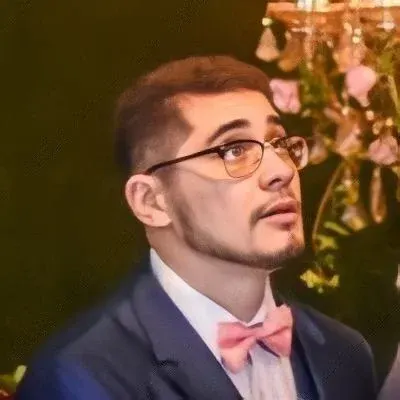
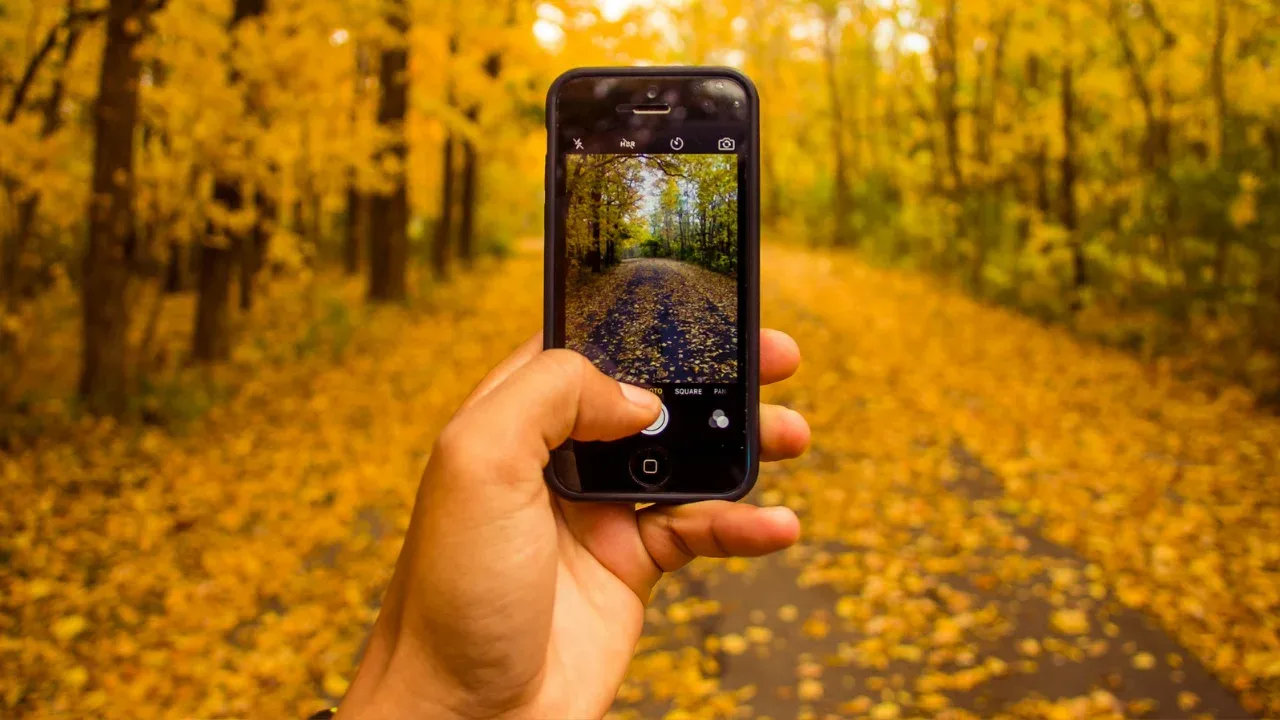
How to Wait in Node.js (JavaScript): A Complete Guide
Do you find yourself needing to pause your Node.js script for an extended period of time? Maybe you're working on a console script that requires a delay between certain actions. Whatever the reason may be, it can be frustrating to discover that Node.js doesn't provide a built-in way to achieve this. But don't worry! In this guide, we'll explore some common issues related to waiting in Node.js and provide easy solutions to help you overcome them.
The Problem: No Built-in Wait Function in Node.js
When it comes to waiting in Node.js, you might have come across code snippets like the following:
setTimeout(function() {
// Code to execute after a period of time
}, 3000);
While this approach might work for simple cases, it falls short when you need to ensure that everything after the line of code executes after the specified period of time. So how can you achieve an extended pause without any complications?
Solution 1: Promises and setTimeout
One way to achieve an extended pause in Node.js is by utilizing promises along with the setTimeout function. Promises allow you to handle asynchronous operations in a more organized and sequential manner. Here's how you can implement this approach:
function wait(ms) {
return new Promise((resolve) => {
setTimeout(resolve, ms);
});
}
// Example usage
async function myScript() {
console.log('Welcome to my console,');
// Pause for 10 seconds
await wait(10000);
console.log('Blah blah blah blah extra-blah');
}
myScript();
In the code above, we've defined a wait
function that returns a promise. This promise resolves after the specified time using the setTimeout function. By using the await
keyword in the myScript
function, we can pause the execution for the desired period of time.
Solution 2: Utilizing External Libraries
If you'd rather not reinvent the wheel and prefer a ready-to-use solution, there are external libraries available that can simplify waiting in Node.js. One popular library is sleep-promise
which provides a clean and straightforward way to introduce delays into your code.
To use sleep-promise
, start by installing it via npm:
npm install sleep-promise
Once installed, you can use it in your code as follows:
const sleep = require('sleep-promise');
// Example usage
(async function myScript() {
console.log('Welcome to my console,');
// Pause for 5 seconds
await sleep(5000);
console.log('Blah blah blah blah extra-blah');
})();
By calling the sleep
function, you introduce a pause in your script without needing to handle promises or managing the timer yourself. It simplifies your code and provides a clean and readable solution.
Conclusion
Waiting in Node.js might not be as straightforward as you initially expected. However, armed with the information and solutions provided in this guide, you now have the tools to achieve an extended pause in your Node.js scripts. Whether you prefer using promises and setTimeout or leveraging external libraries like sleep-promise
, you can now confidently tackle any waiting requirements in your projects.
Now it's your turn to put this knowledge into action! Try implementing these solutions in your own code and let us know how they worked for you. Have any other tips or tricks related to waiting in Node.js? Feel free to share them in the comments below. Happy coding! 😊🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
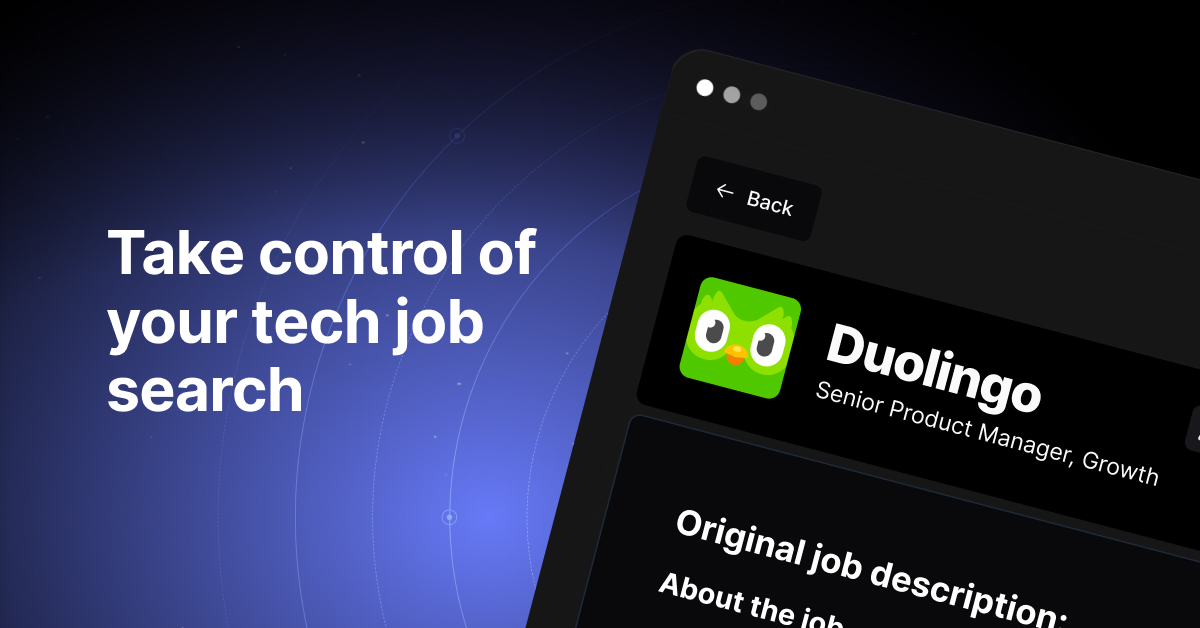