How can I use optional chaining with arrays and functions?
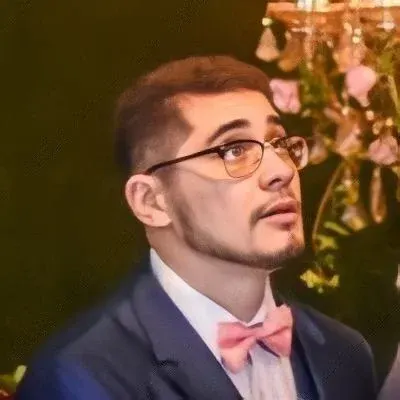
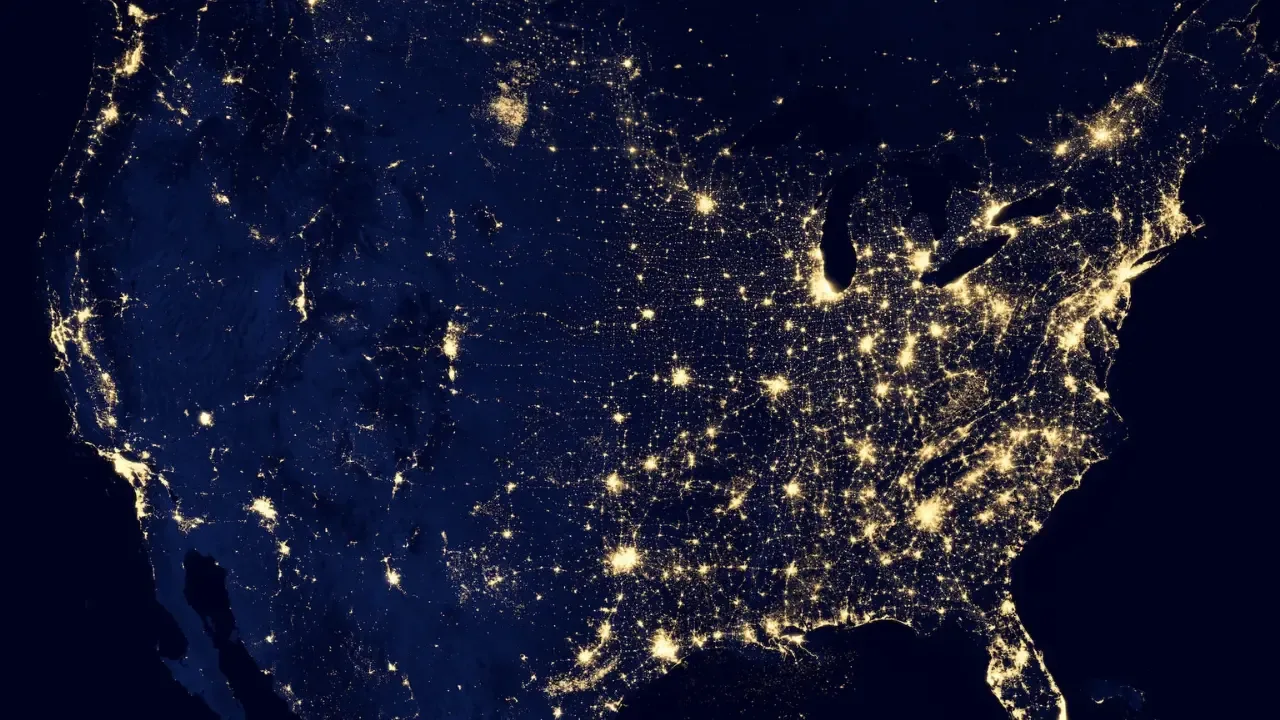
How to Use Optional Chaining with Arrays and Functions 🚀
Are you struggling with using optional chaining with arrays and functions? Don't worry, you're not alone! This can be a tricky concept to grasp at first, but we're here to help you out. In this guide, we'll walk you through the common issues you might encounter and provide easy solutions to overcome them. Let's dive right in! 💪
The Problem 😓
Let's start by understanding the problem at hand. Optional chaining allows you to safely access nested properties or methods without causing an error if any intermediate value is null
or undefined
. While it's commonly used with objects, many developers wonder if it can also be used with arrays and functions.
Here's an example to illustrate the problem:
myArray.filter(x => x.testKey === myTestKey)?[0]
And another example using a function:
let x = {a: () => {}, b: null}
console.log(x?b());
Both of these examples result in errors, indicating that optional chaining is not being applied correctly with arrays and functions.
The Solution 🙌
The good news is that optional chaining can indeed be used with arrays and functions, but the syntax is slightly different. Let's take a look at how to solve this problem:
Using Optional Chaining with Arrays 🌟
To use optional chaining with arrays, you need to wrap the array index or any array-related operation in parentheses followed by the question mark ?
. Let's modify our previous example to correctly use optional chaining with an array:
myArray.filter(x => x.testKey === myTestKey)?.[0]
By adding ?.
before the square brackets, we're ensuring that the array operation is only performed if myArray.filter(x => x.testKey === myTestKey)
is not null
or undefined
. This prevents any errors caused by accessing properties or methods on a null or undefined value.
Using Optional Chaining with Functions 🌟
With functions, the process is similar. Just like with objects, you can use optional chaining by placing a question mark ?
before the function call. Let's update our previous function example to use optional chaining correctly:
let x = {a: () => {}, b: null}
console.log(x?.b());
By adding ?.
before the function call, we ensure that the function is only invoked if x
is not null
or undefined
. This prevents any errors caused by calling methods on a null or undefined value.
That's it! You've now learned how to use optional chaining with arrays and functions. 🎉
Conclusion and Call-to-Action 🏁
Optional chaining is a powerful feature that allows you to write more robust and error-resistant code. By correctly applying optional chaining with arrays and functions, you can safely access nested properties or call methods without the fear of encountering runtime errors.
We hope this guide has provided you with easy-to-understand solutions to common problems when using optional chaining with arrays and functions. If you found this post helpful, be sure to share it with your fellow developers! And if you have any further questions or want to share your own experiences, feel free to leave a comment below.
Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
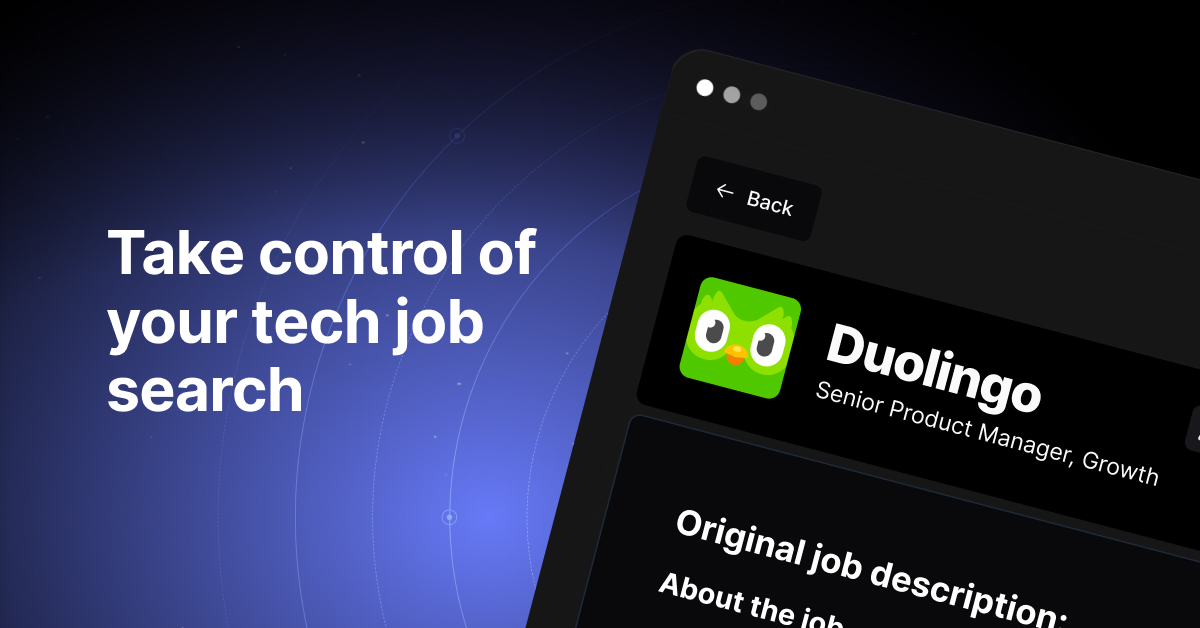