How can I use multiple refs for an array of elements with hooks?
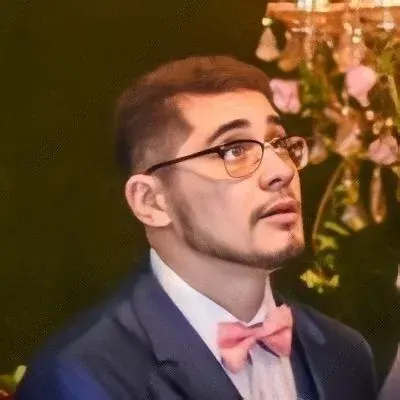
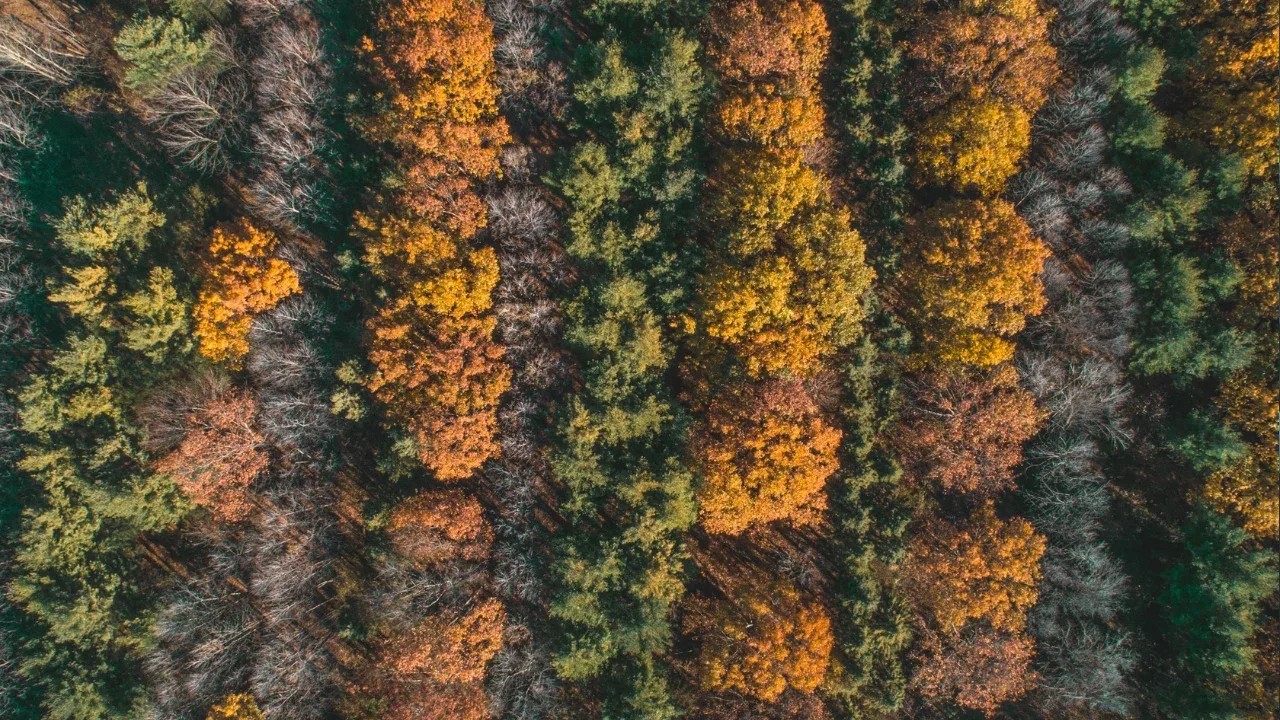
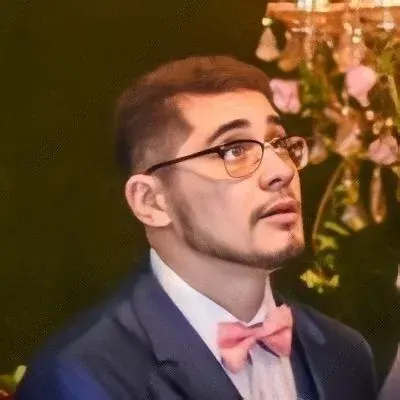
Using Multiple Refs for an Array of Elements with Hooks
š¤ Have you ever wondered how to use multiple refs for an array of elements with React Hooks? Well, you're not alone! Many developers have faced this issue and struggled to find an easy solution. But fret not, I'm here to guide you through it step by step! š
The Problem
In React, using a single ref for a single element is straightforward. You simply create a ref using the useRef
hook, attach it to the desired element, and voila! But when it comes to working with an array of elements, things get a bit trickier. You can't simply use the same ref for multiple elements, right? Let's see how the solution to this problem unfolds. š
The Solution
The solution lies in creating an array of refs, where each ref corresponds to an element in the array. This way, you can individually handle each element separately. Here's how you can do it:
Import the necessary hooks from the React library:
const { useRef, useState, useEffect } = React;
Begin by creating a functional component, let's call it
App
:const App = () => { // Step 3 and 4 will go here };
Declare two new state variables:
elRefs
andelWidths
. TheelRefs
array will store the refs for each element, whileelWidths
will store the width of each element:const [elRefs, setElRefs] = useState([]); const [elWidths, setElWidths] = useState([]);
In the
useEffect
hook, initialize theelRefs
array with refs for each element and set theelWidths
array to match the number of elements, but with all widths initially set to 0:useEffect(() => { setElRefs((refs) => Array(3) // Replace 3 with the actual number of elements in your array .fill() .map((_, i) => refs[i] || React.createRef()) ); setElWidths(Array(3).fill(0)); // Replace 3 with the actual number of elements in your array }, []);
š” In this example, we assume you have three elements in your array. Replace the
3
with the actual number of elements in your case.For each element in your array, render a
<div>
element and attach the corresponding ref from theelRefs
array:return ( <div> {[1, 2, 3].map((el, index) => ( <div key={index} ref={elRefs[index]} style={{ width: `${el * 100}px` }} > Width is: {elWidths[index]}px </div> ))} </div> );
š” Remember to replace
[1, 2, 3]
with your actual array.Update the
useEffect
hook to calculate and update the width of each element when the component mounts or whenever theelRefs
array changes:useEffect(() => { elRefs.forEach((ref, index) => { setElWidths((widths) => widths.map((_, i) => (i === index ? ref.current.offsetWidth : widths[i])) ); }); }, [elRefs]);
š” The
elRefs
array is added as a dependency to theuseEffect
hook to trigger the recalculation of widths whenever it changes.Finally, render the
App
component within the root element of your HTML document:ReactDOM.render(<App />, document.getElementById("root"));
⨠And there you have it! By following these steps, you can easily use multiple refs for an array of elements with React Hooks. š
Conclusion
Using multiple refs for an array of elements might have seemed challenging at first, but with the right approach, it can be easily accomplished. By creating an array of refs and managing their state, you can handle each element individually and perform actions specific to each one.
If you found this guide helpful, don't forget to share it with other developers who might be struggling with the same issue. And also, let me know in the comments how you used this solution in your own projects! I'm excited to see the creative ways you implement it! š
Happy coding! š»š