How can I tell if a DOM element is visible in the current viewport?
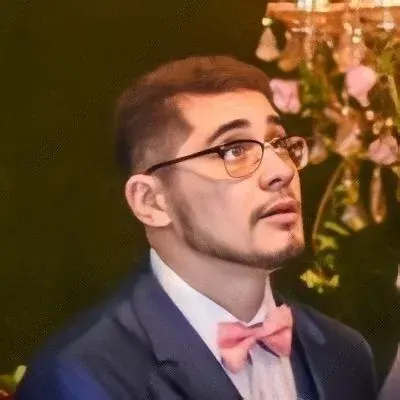
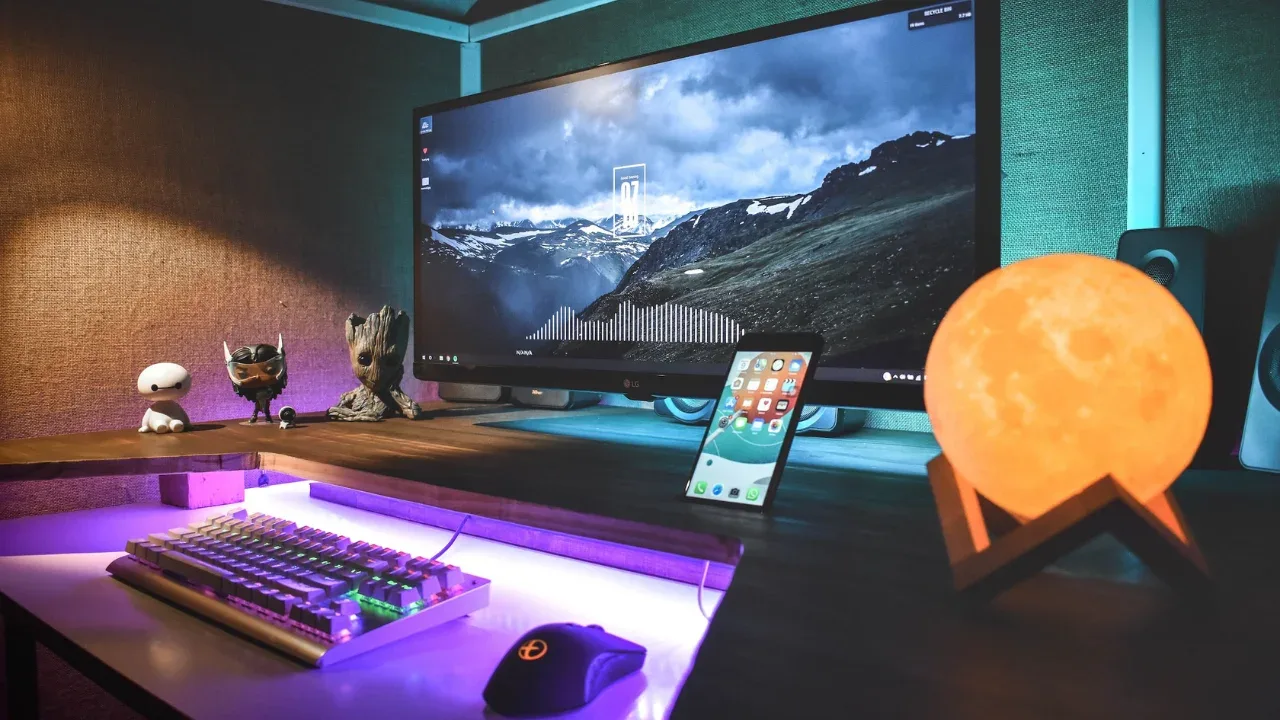
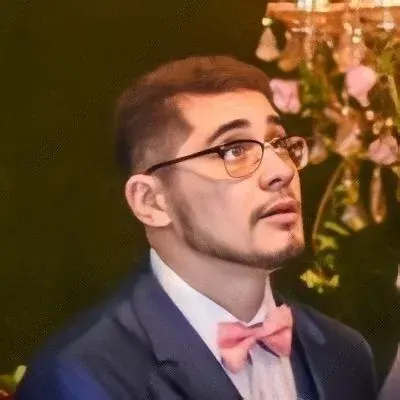
š Tech Blog Post: How to Check if a DOM Element is Visible in the Current Viewport
š Hey there, tech-savvy readers! š Welcome to our blog, where we tackle tricky tech problems in a way that's easy to understand. Today, we're diving into the world of DOM elements and how to determine if they are visible within the current viewport. šÆ
š¤ The Question: Is There an Efficient Way to Check DOM Element Visibility?
We come across this question a lot, and it's a good one! So let's provide some clarity. š
š§ Understanding the Challenge
Before we jump into solutions, let's quickly address what "visibility in the viewport" means. In simple terms, it refers to whether a DOM element is currently visible on the screen or hidden beyond the visible area.
š The Solution: Intersection Observer API
To determine if a DOM element is visible, we have a powerful tool at our disposal: the Intersection Observer API. It's like having a personal sleuth that keeps an eye on DOM element visibility for you! šµļøāāļø
āļø Step 1: Setting Up the Observer
First, we need to create an instance of the Intersection Observer and provide it with a callback function. This function will be executed whenever the observed element comes into or goes out of the viewport.
const observer = new IntersectionObserver(entries => {
entries.forEach(entry => {
// Your code to handle visibility changes here
});
});
āļø Step 2: Observing the Target Element
Next, we need to select the element we want to track and start observing it. This can be done using the observe()
method of the Intersection Observer.
const targetElement = document.querySelector('.your-element');
observer.observe(targetElement);
āļø Step 3: Checking Visibility
Inside the callback function, we can now check the visibility state of the target element using the isIntersecting
property of the entry
object.
const observer = new IntersectionObserver(entries => {
entries.forEach(entry => {
if (entry.isIntersecting) {
// The target element is visible
} else {
// The target element is not visible
}
});
});
š Congratulations! You're now a Visibility Detective!
You did it! With the Intersection Observer API, you can now easily determine if a DOM element is visible within the current viewport. So go ahead and start implementing this technique in your projects. š
š„ Your Turn: Share Your Experience!
Have you encountered challenges related to DOM element visibility in the past? How did you solve them? We'd love to hear your thoughts, experiences, and even alternative solutions in the comments below. Let's geek out and help each other! š¤š¬
That's all for today, tech enthusiasts! We hope this guide has shed some light on this common challenge. Stay tuned for more cool tech tips and tricks coming your way. Until next time, happy coding! ššØāš»