How can I remove or replace SVG content?
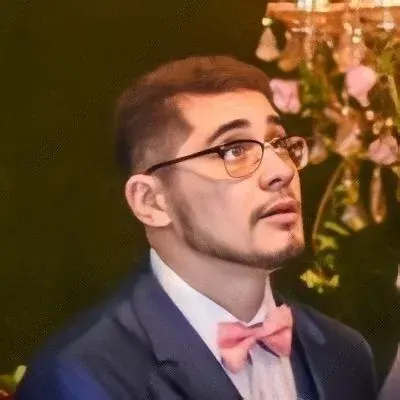
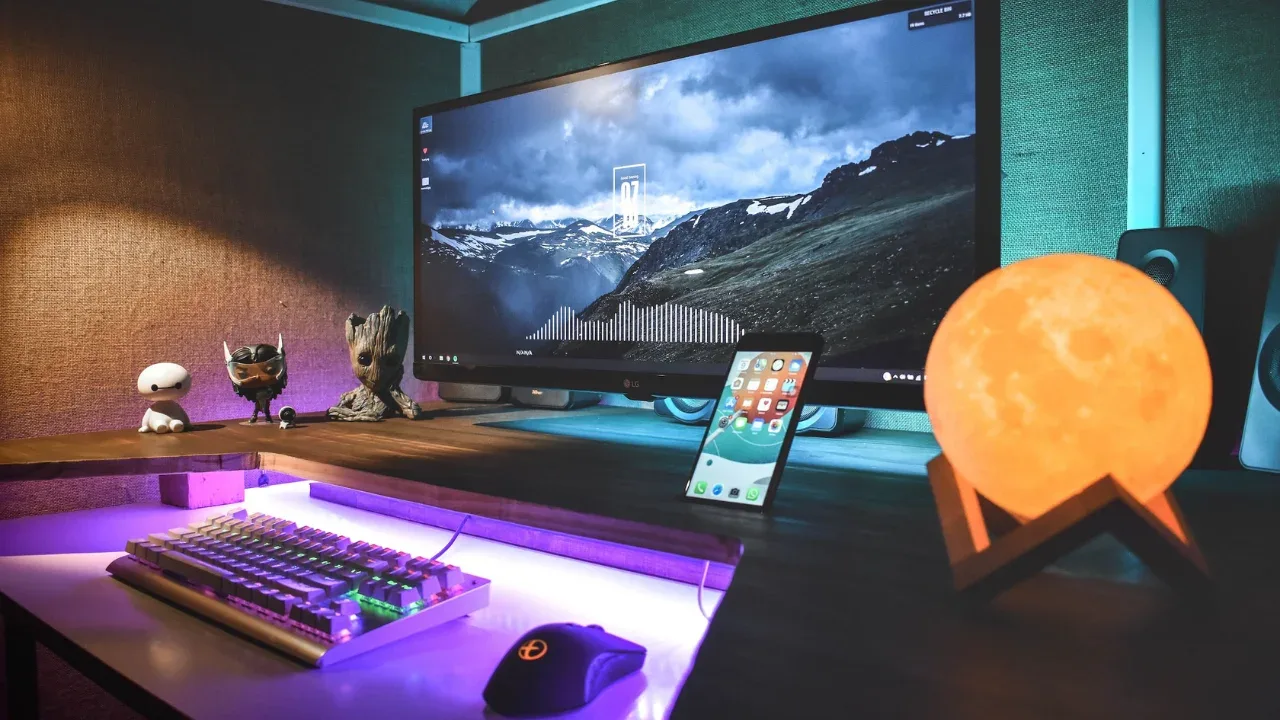
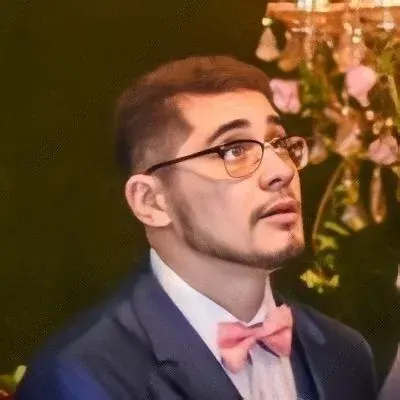
Removing or Replacing SVG Content Made Easy! 🎨🔀
So, you've created a beautiful SVG chart using D3.js, but now you're faced with the challenge of updating it with new data from a web service. Each time you click on the update button, a new SVG element is generated, leaving you with the task of removing the old one or updating its content. Don't worry, we've got you covered! 💪
Let's dive into some easy solutions to remove or replace SVG content. 💥
Solution 1: Removing the Old SVG Element 🗑️
To remove the old SVG element, you can use the .remove()
method provided by D3.js. Here's an example of how you can modify your code to achieve this:
var svg = d3.select("body")
.selectAll("svg") // targets all existing SVG elements
.remove(); // removes them from the DOM
In this code snippet, we use .selectAll("svg")
to select all existing SVG elements present on the page. Then, by chaining .remove()
, we remove these elements from the Document Object Model (DOM). Now, you're ready to create a fresh SVG element with updated content.
Solution 2: Replacing SVG Content 🔄
If you prefer to update the content of the SVG element rather than removing it entirely, D3.js also provides a simple solution. Here's an example of how you can achieve this:
var svg = d3.select("body")
.select("svg") // targets only the first SVG element
.html(""); // empties its content
In this code snippet, we use .select("svg")
to target the first SVG element present on the page. Then, using .html("")
, we empty the content of the SVG element, effectively clearing the slate for new data to be displayed.
Bonus Tip: Combining Solutions for Flexibility 🎭
Depending on your requirements, you can also combine both solutions for maximum flexibility. Here's an example code snippet that allows you to choose between removing the old SVG element or replacing its content:
var svgContainer = d3.select("body");
if (shouldRemoveSVG) {
svgContainer.selectAll("svg").remove(); // Solution 1: Remove existing SVG elements
} else {
svgContainer.select("svg").html(""); // Solution 2: Clear content of the first SVG element
}
In this code snippet, we introduce a conditional statement (if
) that allows you to choose between removing the old SVG elements or emptying the content of the first SVG element. Simply adjust the shouldRemoveSVG
variable based on your needs.
Now that you have these handy solutions, go forth and create amazing data visualizations with confidence! 📊✨
Your Turn! 👩💻👨💻
We hope this guide has helped you understand how to remove or replace SVG content with ease. Give these solutions a try and let us know how they work for you. If you have any questions or other tech-related topics you'd like us to cover, feel free to leave a comment below. Happy coding! 💻🚀