How can I remove a style added with .css() function?
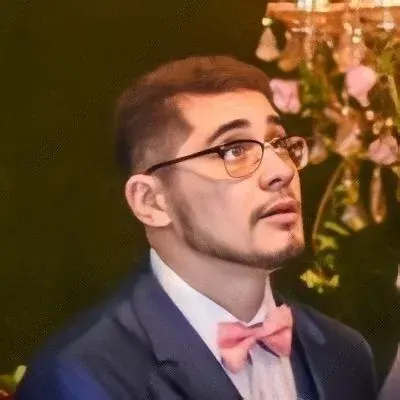
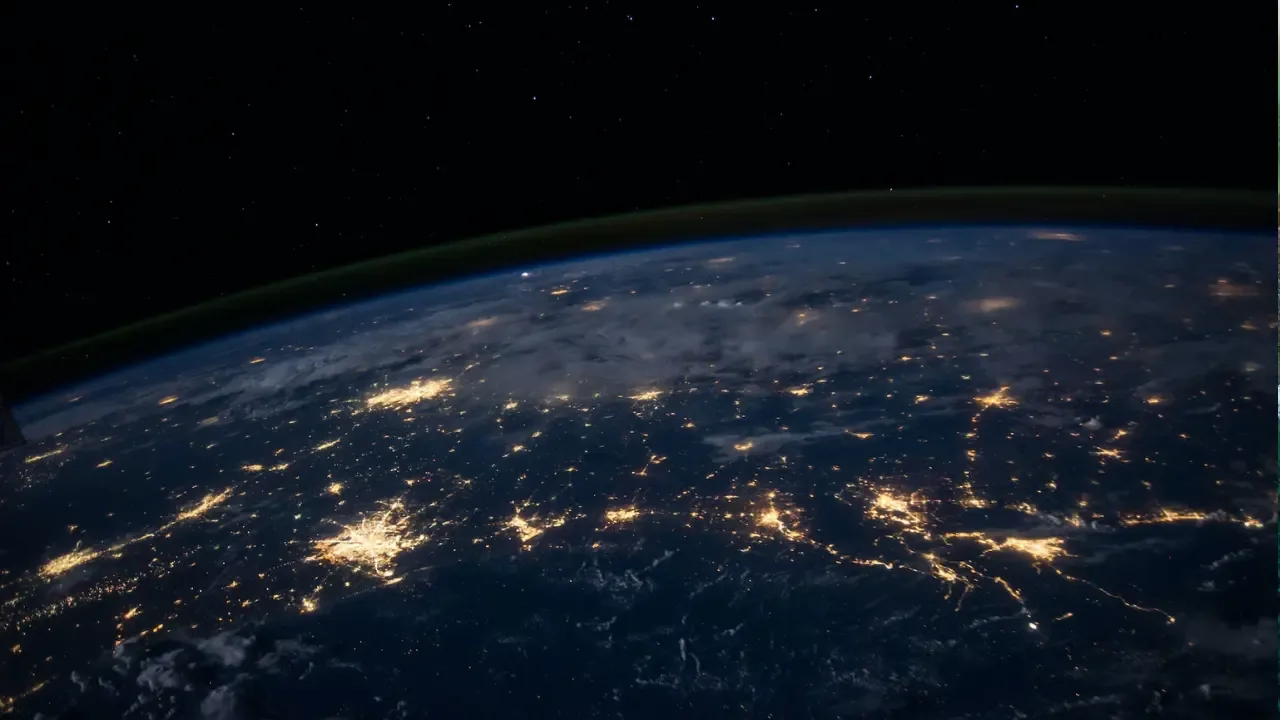
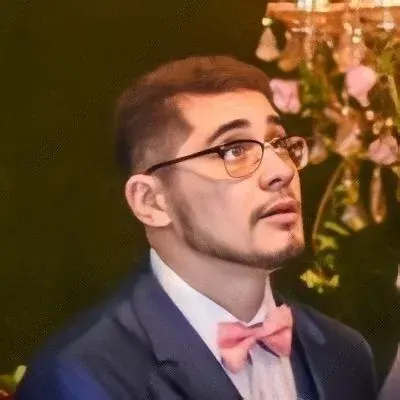
How to Remove a Style Added with .css() Function in jQuery
So, you're using jQuery to dynamically change the CSS of your HTML elements, but now you're facing a challenge: how do you remove a style that was added using the .css() function? 🤔
Let's dive into this common problem and explore some easy solutions!
The Scenario
Imagine you have some code that changes the background color of your <body>
element based on user input. Here's an example:
if (color != '000000') {
$("body").css("background-color", color);
} else {
// remove style ?
}
This code runs whenever a color is selected using a color picker. But how can you remove the inline style when the input value is '000000' (black)? Let's find out!
The Issue
You might initially think of using .css("background-color", "none")
to remove the styling. However, this would also remove the default styling from your CSS files, which is not what you want. 😫
So, how can you selectively remove only the inline style added with jQuery? Luckily, there are a couple of straightforward solutions!
Easy Solutions
1. Remove the property using .css()
One simple solution is to remove the specific CSS property altogether using the .css()
function. Here's how you can achieve that:
if (color != '000000') {
$("body").css("background-color", color);
} else {
$("body").css("background-color", "");
}
By passing an empty string as the value, you effectively remove the inline background-color
style.
2. Use .removeAttr()
Another way to solve this problem is by using the .removeAttr()
function. This method allows you to remove any attribute from an HTML element, including inline styles. Let's see how it works:
if (color != '000000') {
$("body").css("background-color", color);
} else {
$("body").removeAttr("style");
}
With .removeAttr("style")
, you remove the entire style
attribute from the <body>
element. This ensures that any inline styles, including the background-color
, are completely removed.
The Call-to-Action
Voila! You now have two easy solutions to remove a style added with the .css()
function in jQuery. 🎉
Next time you face a similar challenge, you can confidently implement one of these solutions and keep your code clean and efficient!
If you found this guide helpful or have any additional tips, we'd love to hear from you! Share your thoughts in the comments below. Let's keep learning together! 💡