How can I remove a specific item from an array in JavaScript?
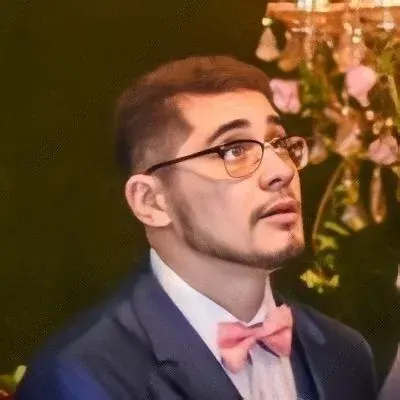
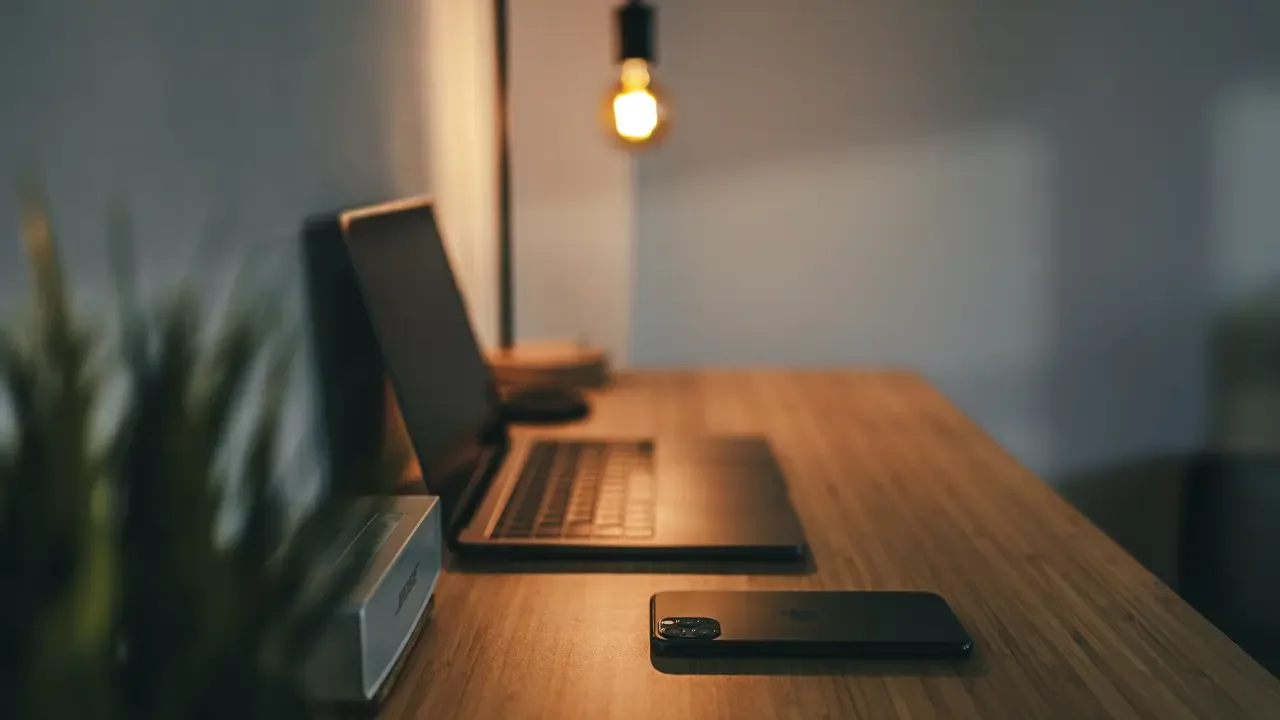
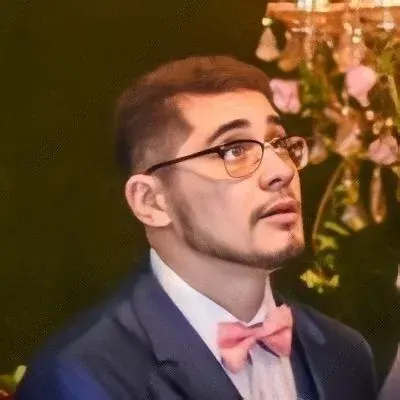
Removing a Specific Item from an Array in JavaScript 😎💪
So you want to remove a specific item from an array in JavaScript, huh? No worries, my friend! In this tutorial, we will walk through different solutions that will help you achieve this task using pure JavaScript. Let's dive in! 🏊♂️💥
The Challenge 🤔
The challenge here is to remove a particular value from an array without using any frameworks. Don't worry if it sounds tricky; I've got your back! 🙌
Here's what the question looks like:
array.remove(value);
Solution 1: Using the filter()
Method 🗑️
One of the easiest ways to remove a specific item from an array is by using the filter()
method. This method creates a new array based on a condition you specify. In our case, we will filter out the specific value we want to remove. Check out the code below: 🖥️👇
const newArray = array.filter(item => item !== value);
This code will create a new array called newArray
that excludes the specified value. Easy-peasy, right? 👌
Solution 2: Using the splice()
Method 🔪
Another option at your disposal is the trusty splice()
method. This method allows you to modify the contents of an array by removing or replacing existing elements. You can use it to remove a specific value by determining the index of the value you want to remove. Take a look at the code snippet below: 💻👇
const index = array.indexOf(value);
if (index > -1) {
array.splice(index, 1);
}
In this example, we find the index of the value using the indexOf()
method. If the index is greater than -1 (indicating that the value exists in the array), we remove it using splice()
. Boom! 💥
Solution 3: Using a for
Loop 🔄
You can always resort to good ol' loops if you prefer a more traditional approach. You can iterate through the array and remove the specific value using a for
loop. Check out the code snippet below: 🔁👇
for (let i = array.length - 1; i >= 0; i--) {
if (array[i] === value) {
array.splice(i, 1);
}
}
This code snippet iterates through the array in reverse order (to avoid index shifting), checks if each element matches the specified value, and removes it if it does. 💪
Final Thoughts and the Call to Action 🎉
Now that you have three different ways to remove a specific item from an array in JavaScript, it's time to put your knowledge into practice! Choose the method that suits your coding style and requirements, and start removing those pesky values. 💢💻
Do you know any other cool way to tackle this problem in JavaScript? Share it with us in the comments section! Let's learn and grow together. 😄✨
Remember, practice makes perfect! Get your hands dirty with some code and experience the power of JavaScript array manipulation!
Thanks for reading! 🙏📚 And don't forget to share this useful guide with your fellow developers! 😉
Happy coding! 💻💡